Magic number allows the EA to identify its orders.
You only need one per strategy per timeframe.
You do not need one per symbol; that is part of your Order Select loop filtering.
There is no need to generate them. Simply make sure all different EAs use different ones.
Magic number allows the EA to identify its orders.
You only need one per strategy per timeframe.
You do not need one per symbol; that is part of your Order Select loop filtering.
There is no need to generate them. Simply make sure all different EAs use different ones.
ok thx
Magic number allows the EA to identify its orders.
You only need one per strategy per timeframe.
You do not need one per symbol; that is part of your Order Select loop filtering.
There is no need to generate them. Simply make sure all different EAs use different ones.
one more question Sir, do I need to use for every order a different MagicNumber?
Example: EURUSD M5 Order1 magic: 123456
EURUSD M5 Order2 magic: 123456
or
Example: EURUSD M5 Order1 magic: 123456
EURUSD M5 Order2 magic: 654321
does MetaTrader have randomNumber generator?
No, not needed as each order gets a unique ticket number that you can track! You can continue to use same magic number for all orders irrespective of symbol, for a particular EA working on a particular time-frame.
- different Symbol = same magic number (as long as your code checks for Symbol name as well)
- different Time-frame = different magic number
- different EA = different magic number
Yes, MathRand(). Please read the documentation!
EDIT: However, I DO NOT recommend generating random magic number as you will end up having a different Magic each time an EA starts and then you will have a much more difficult task when you want to pick up with trades that were left stranded if the terminal or EA crashes and needs to restart.
And you will also have a very difficult time keeping track of all magic numbers in order to produce reports and analysis of your trading.
Use fixed magic number as per your requirements and keep a hard record of how they are used, per EA per time-frame.
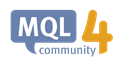
No, not needed as each order gets a unique ticket number that you can track! You can continue to use same magic number for all orders irrespective of symbol, for a particular EA working on a particular time-frame.
- different Symbol = same magic number (as long as your code checks for Symbol name as well)
- different Time-frame = different magic number
- different EA = different magic number
Thank you so much Sir that was all I need to know
Yes, MathRand(). Please read the documentation!
EDIT: However, I DO NOT recommend generating random magic number as you will end up having a different Magic each time an EA starts and then you will have a much more difficult task when you want to pick up with trades that were left stranded if the terminal or EA crashes and needs to restart.
And you will also have a very difficult time keeping track of all magic numbers in order to produce reports and analysis of your trading.
Use fixed magic number as per your requirements and keep a hard record of how they are used, per EA per time-frame.
thx for the function name "mathrand()"

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I'm working the course of Jim Hodges at the moment he is teaching how to use magicNumbers, my question is there a better way to do it or is this the normal way(generating Magicnumber)?