39863092:
Hello,
after several hours of searching on google, Youtube and on the forum I finally found a way to export the mt5 data to Excel thanks to a small MFC application (DDE server), the problem is that the code allows to have only the name of a company that serves the account, the server time the current mobile average as well as the price of some currencies.
Could someone help me with the code so that I can get the transaction history (purchase, sales, dates, value), gain, loss, balance, margin, portfolio, profit ...
Thank you in advance.
Suggestion: https://www.mql5.com/en/code/18801
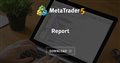
Report
- www.mql5.com
The MetaTrader 4/5 library allows generating reports based on the trading history.
There is a line in which the company name is being set, you could overwrite that part with a static string instead of using the company name given by the terminal.
Also it may be an option to export/import as a csv file.

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Hello,
after several hours of searching on google, Youtube and on the forum I finally found a way to export the mt5 data to Excel thanks to a small MFC application (DDE server), the problem is that the code allows to have only the name of a company that serves the account, the server time the current mobile average as well as the price of some currencies.
Could someone help me with the code so that I can get the transaction history (purchase, sales, dates, value), gain, loss, balance, margin, portfolio, profit ...
Thank you in advance.