A solution could be to track OnTrade() and recreate the objects there.
Else you could check in OnTradeTransaction () if the order has "transformed" into a position and then not remove the objects.
Dominik Egert:
A solution could be to track OnTrade() and recreate the objects there.
Else you could check in OnTradeTransaction () if the order has "transformed" into a position and then not remove the objects.
Thank you!
I gave this a try and it seems to work fine:
if (trans.type==TRADE_TRANSACTION_ORDER_DELETE && trans.order_state==ORDER_STATE_CANCELED)
Try this:
//+------------------------------------------------------------------+ //| TradeTransaction function | //+------------------------------------------------------------------+ void OnTradeTransaction(const MqlTradeTransaction &trans, const MqlTradeRequest &request, const MqlTradeResult &result) { //--- if(trans.type == TRADE_TRANSACTION_HISTORY_ADD && (trans.order_type >= 2 && trans.order_type < 6) && trans.order_state == ORDER_STATE_CANCELED) { PrintFormat("Pending order is deleted. (order ticket #%I64u, symbol: %s, type: %s)", trans.order, trans.symbol, EnumToString(trans.order_type)); } } //+------------------------------------------------------------------+
You could also use my TradeTransaction Class to handle all types of trade transactions, easily.
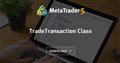
amrali:
Try this:
You could also use my TradeTransaction Class to handle all types of trade transactions, easily.
Your TradeTransaction Class is very interesting. Thank you!

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Hello guys,
when I place a pending order I draw three objects to visualize the SL and TP.
If I delete this pending order manually I want that these three objects are deleted automatically. That works fine.
My problem is that these objects are also deleted when the order is executed. I read that this is normal
because TRADE_TRANSACTION_ORDER_DELETE also reacts if the pending order is executed.
I tried to use HistoryOrderGetInteger and used ORDER_STATE but that didn't work.
Can anyone tell me how to code it so that the three objects are only deleted if the pending order is cancelled?
This is my code so far: