Hi Guys ,
Does anybody know what's wrong with this code ?
Wrong code: at every tick, it redraws bars throughout the entire HISTORY.
Thanks for your reply. So what's the right code ? :)
Thanks for your reply. So what's the right code ? :)
You can't count the WHOLE HISTORY AT EVERY TICK. You need to use a lean algorithm.
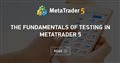
- www.mql5.com
You cannot stop ALL bars at every tick. Read the help. Look for articles on "Zero Bar Recalculation".
Vladimir , I'm reading the help but it's 11 years old guide made out of horrible short explanations. If the ref guide where clear enough there were no need to post thousands of threads and articles.
I just don't understand why Metaquotes don't give a fuck about that...
Anyway I'm not able to find that article. Could you please link it ? :)
Tks
You are showing ignorance and disrespect for MetaQuotes. The company invests enormous resources in training. It should be respectful of such a titanic work. Open any indicator from the standard delivery - this is an example of economical recalculation on a zero bar.
Sorry Vladimir ,
I could run a petition to let you know how many people think the same thing. I have expressed my idea whether you like it or not. The ref guide is briefly written and there are dozens of articles and threads to read in order to learn just one simple thing. Do you really think that learning mql5 in this way it's fun and productive ? Anyway thank you for your reply I'll look another way to fix the problem by myself before switching to jforex again. Tks
Sorry Vladimir ,
I could run a petition to let you know how many people think the same thing. I have expressed my idea whether you like it or not. The ref guide is briefly written and there are dozens of articles and threads to read in order to learn just one simple thing. Do you really think that learning mql5 in this way it's fun and productive ? Anyway thank you for your reply I'll look another way to fix the problem by myself before switching to jforex again. Tks
I will now prepare an example ...
I will now prepare an example ...
Thank you very much Vladimir. Nothing against you of course. But believe me , approaching MQL5 for a beginner it's an absolute mess and you give up after a few trials. That's what I'm trying to say.
:)
Code:
//+------------------------------------------------------------------+ //| DRAW_COLOR_CANDLES.mq5 | //| Copyright 2011, MetaQuotes Software Corp. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2011, MetaQuotes Software Corp." #property link "https://www.mql5.com" #property version "1.00" #property description "An indicator to demonstrate DRAW_COLOR_CANDLES." #property indicator_separate_window #property indicator_buffers 5 #property indicator_plots 1 //--- plot ColorCandles #property indicator_label1 "ColorCandles" #property indicator_type1 DRAW_COLOR_CANDLES //--- Define 8 colors for coloring candlesticks (they are stored in the special array) #property indicator_color1 clrRed,clrBlue,clrGreen #property indicator_style1 STYLE_SOLID #property indicator_width1 1 //--- input parameters //--- //--- Indicator buffers double BufferOpen[]; double BufferHigh[]; double BufferLow[]; double BufferClose[]; double BufferColors[]; //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- indicator buffers mapping SetIndexBuffer(0,BufferOpen,INDICATOR_DATA); SetIndexBuffer(1,BufferHigh,INDICATOR_DATA); SetIndexBuffer(2,BufferLow,INDICATOR_DATA); SetIndexBuffer(3,BufferClose,INDICATOR_DATA); SetIndexBuffer(4,BufferColors,INDICATOR_COLOR_INDEX); //--- An empty value PlotIndexSetDouble(0,PLOT_EMPTY_VALUE,0.0); //--- The name of the symbol, for which the bars are drawn string symbol=Symbol(); //--- Set the display of the symbol PlotIndexSetString(0,PLOT_LABEL,symbol+" Open;"+symbol+" High;"+symbol+" Low;"+symbol+" Close"); IndicatorSetString(INDICATOR_SHORTNAME,"DRAW_COLOR_CANDLES("+symbol+")"); //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { int limit=prev_calculated-1; if(prev_calculated==0) limit=0; for(int i=limit; i<rates_total; i++) { BufferOpen[i]=open[i]; BufferHigh[i]=high[i]; BufferLow[i]=low[i]; BufferClose[i]=close[i]; if(open[i]<close[i]) BufferColors[i]=0.0; else if(open[i]>close[i]) BufferColors[i]=1.0; else BufferColors[i]=2.0; } //--- return value of prev_calculated for next call return(rates_total); } //+------------------------------------------------------------------+
Result:

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi Guys ,
Does anybody know what's wrong with this code ?