This is probably because the original rates_total is too high for CopyBuffer on the higher timeframe. You need to 'translate' rates_total and prev_calculated.
But if you only intend to use Macd there's an easier solution - instead of calculating it for the higher timeframe you can multiply its periods. A Macd(12,26,9) on D1 is roughly the same as Macd(12*6,26*6,9*6) on H4.
So instead of working with MTF history data you could just adapt the Macd periods and stick with the current timeframe.
This is probably because the original rates_total is too high for CopyBuffer on the higher timeframe. You need to 'translate' rates_total and prev_calculated.
But if you only intend to use Macd there's an easier solution - instead of calculating it for the higher timeframe you can multiply its periods. A Macd(12,26,9) on D1 is roughly the same as Macd(12*6,26*6,9*6) on H4.
So instead of working with MTF history data you could just adapt the Macd periods and stick with the current timeframe.
Thanks for reply.
Can you please help me and give more details how to 'translate' rates_total and prev_calculated?
That was max what I could do so if you will able to help me and edit code I will be very very grateful. Thanks in advanced.
int D1factor=PeriodSeconds(PERIOD_D1)/PeriodSeconds(); if(D1factor==0) { ...bad timeframe message return INIT_FAILED; } macdD1Handle = iCustom(_Symbol, _Period, "Market\\Traditional MACD", "", MACDD1FastEMAPeriod*D1factor, MACDD1SlowEMAPeriod*D1factor, MACDD1SignalEMAPeriod*D1factor, MACDD1AppliedPrice, true, false, "", 5000, false, clrRed, clrDarkGreen, STYLE_SOLID, 2, false, STYLE_DOT, 1, false, clrSalmon, clrLawnGreen, STYLE_SOLID, 2, false, STYLE_DOT, 1, "", false, false, false, "alert.wav", false, false);
Like so maybe.
Like so maybe.
No this is not helping. I want to get correct points. I have download many MTF indicators and all has this problem error:4806. Even code provided by MetaQuote itself has this problem when I use different timeframe then current. link for MetaQuote code: https://www.mql5.com/en/docs/indicators/imacd
So if you can and it will be easier for you just help me with this demo from MetaQuote and then I will do rest in my code.
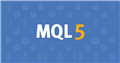
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
My name is Revazi, I am trying to made MultiTimeframe indicator based on Custom indicator called Traditional MACD from MQL5 Market(https://www.mql5.com/en/market/product/2593).
I am using this indicators main line and as soon as line crosses above 0 level I want to make blue arrow(ArrowCode=116) and make reversal for below cross of 0 level with red dot.
Everything works fine till I am using Curreent_Timeframe but when I change it to different timeframe then indicator doesn't draw anything. Below is full source code:
I have check lots of articles and posts but unfortunately couldn't fixed this indicator still so if someone can help me I will be very very grateful.
Thanks to all in advanced.