Before you try to explore every potential error you can make as a beginner here have you searched (top right the lens) for already existing code?
Maybe e.g. fractals will do what you want at least you can look how they have managed to find highs and lows..
But you can search for resistance and support as well.
Before you try to explore every potential error you can make as a beginner here have you searched (top right the lens) for already existing code?
Maybe e.g. fractals will do what you want at least you can look how they have managed to find highs and lows..
But you can search for resistance and support as well.
-
for(i2=i-1;i2<i-200 && !IsStopped();i--)
If i2 ever becomes negative you have array exceeded.
-
to_copy=rates_total-prev_calculated; to_copy++; } //copy indicator buffers to arrays int copyATR = CopyBuffer(handleATR,0,0,to_copy,ATRBuffer); int copySH = CopyBuffer(handleSHSL,0,0,to_copy,SHBuffer); int copySL = CopyBuffer(handleSHSL,1,0,to_copy,SLBuffer);
Your i2 loop goes back to the earlier candles, yet you don't provide them.
-
If i2 ever becomes negative you have array exceeded.
- Your i2 loop goes back to the earlier candles, yet you don't provide them.
// check there is enough bars if(rates_total<barsback) return(0); int to_copy; //number of values to copy from indicator buffers if(prev_calculated>rates_total || prev_calculated<=0) to_copy=rates_total; else { to_copy=rates_total-prev_calculated; to_copy++; } //copy indicator buffers to arrays int copyATR = CopyBuffer(handleATR,0,0,to_copy,ATRBuffer); int copySH = CopyBuffer(handleSHSL,0,0,to_copy,SHBuffer); int copySL = CopyBuffer(handleSHSL,1,0,to_copy,SLBuffer); //error checks if(copySL<0) Print("copy SL failed" + (string)GetLastError()); if(copySH<0) Print("copy SH failed" + (string)GetLastError()); if(copyATR<0) Print("copy ATR failed" + (string)GetLastError()); //set as series ArraySetAsSeries(SHBuffer,false); ArraySetAsSeries(SLBuffer,false); ArraySetAsSeries(ATRBuffer,false); int limit,i,i2; // if it the first call, then set the limit to calculate for all bars, otherwise only calculate for new bars. Set arrays to empty if(prev_calculated <= barsback +1) { limit = barsback; ArrayInitialize(BottomSupportLine,EMPTY_VALUE); ArrayInitialize(TopSupportLine,EMPTY_VALUE); ArrayInitialize(BottomResistanceLine,EMPTY_VALUE); ArrayInitialize(TopResistanceLine,EMPTY_VALUE); } //else check everytime a new swing could appera. else limit = rates_total-(SHSLperiod*2); for(i=limit;i<rates_total && !IsStopped();i++) { //check for a swing high on the current bar, if its true begin a loop which looks back for a second swing high in the last n bars if(SHBuffer[i] != EMPTY_VALUE) { for(i2=i-1;i2<i-barsback && !IsStopped();i--) { if(SHBuffer[i2] != EMPTY_VALUE) //check to see if this new swing high is within ATR above or below the current swing high if(SHBuffer[i2] > SHBuffer[i]-ATRBuffer[i] && SHBuffer[i2] < SHBuffer[i]+ATRBuffer[i]) { // if its above, then set the top line to this swing high and the bottom to the current and break. otherwise do the opposite. if(SHBuffer[i2] > SHBuffer[i]) { TopResistanceLine[i2] = high[i2]; TopResistanceLine[i] = high[i2]; BottomResistanceLine[i2] = high[i]; BottomResistanceLine[i] = high[i]; break; } else { TopResistanceLine[i2] = high[i]; TopResistanceLine[i] = high[i]; BottomResistanceLine[i2] = high[i2]; BottomResistanceLine[i] = high[i2]; break; } } //otherwise set to empty else { TopResistanceLine[i2] = EMPTY_VALUE; TopResistanceLine[i] = EMPTY_VALUE; BottomResistanceLine[i2] = EMPTY_VALUE; BottomResistanceLine[i] = EMPTY_VALUE; } } } } return(rates_total); } //+------------------------------------------------------------------+
input int SHSLperiod = 5; input int barsback = 200;
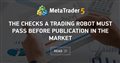
- www.mql5.com
Hello,
I am at the beginning of trying to create a support and resistance indicator which uses swing high and lows.
I have started with a bit of code which should look back looks for two swings highs and checks whether they come within ATR of each other. The issue I am having is I want to run a loop within the overall indicator loop, but it doesn't seem to be running, or isn't outputting what I am looking for. I cant for the life of me find a solution, so I feel like I am missing something! The final indicator will likely need a few nested loops for multiple look backs so I need to understand if I am using nesting loops incorrectly.
I have used a custom indicator to swings, which is attached.
Any help is much appreciated..
I would also advise you to stay consistent with your code if you use Deinit of void type then use void OnInit function instead of int OnInit function .
I would also advise you to stay consistent with your code if you use Deinit of void type then use void OnInit function instead of int OnInit function .

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello,
I am at the beginning of trying to create a support and resistance indicator which uses swing high and lows.
I have started with a bit of code which should look back looks for two swings highs and checks whether they come within ATR of each other. The issue I am having is I want to run a loop within the overall indicator loop, but it doesn't seem to be running, or isn't outputting what I am looking for. I cant for the life of me find a solution, so I feel like I am missing something! The final indicator will likely need a few nested loops for multiple look backs so I need to understand if I am using nesting loops incorrectly.
I have used a custom indicator to swings, which is attached.
Any help is much appreciated..