The indicator handle in MQL5 SHOULD BE RECEIVED ONCE - IN OnInit ()!
The indicator handle in MQL5 SHOULD BE RECEIVED ONCE - IN OnInit ()!
If not then pls elaborate.
You mean that those double ma[] & double close[] declarations should be in OnInit() fn?
If not then pls elaborate.
Reference Guide: iMA
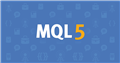
- www.mql5.com
I am still learning to code and this code was given as an introductory sample...
It used ORDER_FILLING_FOK, but with both ORDER_FILLING_FOK and ORDER_FILLING_RETURN it gave an error as "unsupported filling mode".
So i tried with ORDER_FILLING_IOC. Now it opened the order but didn't seem to close it. In the journal it seems to be unable to change sl/tp saying failed to modify position(details) & position doesn't exist.
i tried increasing the sleep delay and deviation but still no help.
Pls help me find the error.
The position id is missing in your modify request.
The position id is missing in your modify request.
Ty...i'll try modifying it.
request.position = PositionGetInteger(POSITION_TICKET);
Code samples see here:
https://www.mql5.com/en/docs/constants/tradingconstants/enum_trade_request_actions
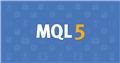
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I am still learning to code and this code was given as an introductory sample...
It used ORDER_FILLING_FOK, but with both ORDER_FILLING_FOK and ORDER_FILLING_RETURN it gave an error as "unsupported filling mode".
So i tried with ORDER_FILLING_IOC. Now it opened the order but didn't seem to close it. In the journal it seems to be unable to change sl/tp saying failed to modify position(details) & position doesn't exist.
i tried increasing the sleep delay and deviation but still no help.
Pls help me find the error.
Here is the original code with ORDER_FILLING_FOK