You haven't posted any outcomes of your backtests, so it is difficult to judge whether the code behaves as it should.
However, there are some other mistakes in your code. You create handles to functions in the OnTick() function. For example:
myMovingAverageDefinition = iMA (_Symbol,_Period,500,0,MODE_EMA,PRICE_MEDIAN);
You have done this for several iMA and iRSI handles. This is incorrect! These handle definitions must be done only once, and should be placed in the OnInit() function.
Thank for the reply, sorry if I was unclear the issue that I am specifically confused by is that as you can see from the log,the orders for Sell were all placed at once. The code should be cycling through the orders and checking them against a time counter as seen. Where as the code is written as current time subtracted from when the time the order was placed plus a 24 hour time period. Meaning in theory that when the counter gets past that 24hr time period it sends a signal that it is okay to place a trade. Which is where the confusion is coming from since clearly that is not happening, so I was wondering if this i a nature part of it. As in it just instantly recognized that an order could be filled and maxed it out under the given count. Or is it an issue with the code. As always, Thank you in advance
PR 0 21:55:03.577 Tester EURUSD,H1 (MetaQuotes-Demo): every tick generating
HK 0 21:55:03.577 Tester EURUSD,H1: testing of Experts\MOA Alternatiting robot 3.ex5 from 1980.07.01 00:00 to 2020.04.23 00:00 started
QI 0 21:55:12.733 Trade 1985.10.28 00:00:00 instant sell 0.05 EURUSD at 0.74080 tp: 0.72080 (0.74080 / 0.74130 / 0.74080)
EH 0 21:55:12.733 Trades 1985.10.28 00:00:00 deal #2 sell 0.05 EURUSD at 0.74080 done (based on order #2)
EG 0 21:55:12.733 Trade 1985.10.28 00:00:00 deal performed [#2 sell 0.05 EURUSD at 0.74080]
OM 0 21:55:12.733 Trade 1985.10.28 00:00:00 order performed sell 0.05 at 0.74080 [#2 sell 0.05 EURUSD at 0.74080]
MQ 0 21:55:12.739 Trade 1985.10.28 00:00:00 instant sell 0.05 EURUSD at 0.74082 tp: 0.72082 (0.74082 / 0.74132 / 0.74082)
IO 0 21:55:12.739 Trades 1985.10.28 00:00:00 deal #3 sell 0.05 EURUSD at 0.74082 done (based on order #3)
OO 0 21:55:12.739 Trade 1985.10.28 00:00:00 deal performed [#3 sell 0.05 EURUSD at 0.74082]
LE 0 21:55:12.739 Trade 1985.10.28 00:00:00 order performed sell 0.05 at 0.74082 [#3 sell 0.05 EURUSD at 0.74082]
EI 0 21:55:12.754 Trade 1985.10.28 00:00:00 instant sell 0.05 EURUSD at 0.74085 tp: 0.72085 (0.74085 / 0.74135 / 0.74085)
QG 0 21:55:12.754 Trades 1985.10.28 00:00:00 deal #4 sell 0.05 EURUSD at 0.74085 done (based on order #4)
NG 0 21:55:12.754 Trade 1985.10.28 00:00:00 deal performed [#4 sell 0.05 EURUSD at 0.74085]
IM 0 21:55:12.754 Trade 1985.10.28 00:00:00 order performed sell 0.05 at 0.74085 [#4 sell 0.05 EURUSD at 0.74085]
JR 3 21:55:16.841 Tester stopped by user
DE 0 21:55:16.848 127.0.0.1 prepare for shutdown
MG 0 21:55:16.855 test Experts\MOA Alternatiting robot 3.ex5 on EURUSD,H1 thread finished
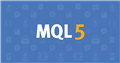
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hey so I have coded an expert advisor but I am having an issue with initiating a part of the code correctly
Specifically with this portion of the code. I cant tell if its spamming multiple orders as soon as it has reached true and just. Or it is just not initiating at all. The idea behind it is to count the time since and order was placed so every 24 hrs it will place a new order. Cant tell if i messed up on writing the code or its just working in a way that i did not anticipate.
They are pretty much the same code just one is to loop through buy, and the other for sell.
Thank you in advance.