You can not create the same object again (4200) you have to either delete the old object first or give the new object a different name, or you can check the existence of the object before you create it.
ERR_OBJECT_ALREADY_EXISTS | 4200 | Object already exists. |
If you want to move it use ObjectMove() or ObjectSetDouble() etc. to assign the object it's new values.
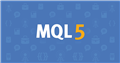
- www.mql5.com
MetaTrader4 v4.00 Build 1260
Tested on MetaQuotes Data from 2020.06.15 to 2020.06.16 on EURUSD M1
Using a For Loop Operator and ObjectCreate(), we create a VLine the first time a candle has had a higher low and a higher high than iBar1 (last finished candle).
Method 1 doesn't work even though it reports the correct x before and after the object create function, while method 2 works while reporting an incorrect x value both before and after the object create function.
As per method 1; why does Object Create not use the x variable as the Print() suggests and we instead need to reverse the For Loop (as per method 2) just to get the Object Create function to correctly use the desired x value as originally intended?
Try something like this
ObjectCreate(ChartID(),"NewVLine_"+IntegerToString(x),OBJ_VLINE,0,iTime(_Symbol,PERIOD_CURRENT,x),iHigh(_Symbol,PERIOD_CURRENT,x),iTime(_Symbol,PERIOD_CURRENT,1),iLow(_Symbol,PERIOD_CURRENT,1));
You can not create the same object again (4200) you have to either delete the old object first or give the new object a different name, or you can check the existence of the object before you create it.
ERR_OBJECT_ALREADY_EXISTS | 4200 | Object already exists. |
If you want to move it use ObjectMove() or ObjectSetDouble() etc. to assign the object it's new values.
- nadiawicket:
ObjectCreate(ChartID(),"NewVLine",OBJ_VLINE,0,iTime(_Symbol,PERIOD_CURRENT,x),iHigh(_Symbol,PERIOD_CURRENT,x),iTime(_Symbol,PERIOD_CURRENT,1),iLow(_Symbol,PERIOD_CURRENT,1));
You can only create one of a given name ("NewVLine")
- Fernando Morales: Try something like this
ObjectCreate(ChartID(),"NewVLine_"+IntegerToString(x),OBJ_VLINE,0,iTime(_Symbol,PERIOD_CURRENT,x),iHigh(_Symbol,PERIOD_CURRENT,x),iTime(_Symbol,PERIOD_CURRENT,1),iLow(_Symbol,PERIOD_CURRENT,1));
You can't use an as-series index in names as they are not unique. As soon as a new bar starts, you will be trying to create a new name (e.g. "name0",) same, existing, previous, name (e.g. "name0" now on bar one.)
Use time (as int) or a non-series index:
#define SERIES(I) (Bars - 1 - I) // As-series to non-series or back.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
MetaTrader4 v4.00 Build 1260
Tested on MetaQuotes Data from 2020.06.15 to 2020.06.16 on EURUSD M1
Using a For Loop Operator and ObjectCreate(), we create a VLine the first time a candle has had a higher low and a higher high than iBar1 (last finished candle).
Method 1 doesn't work even though it reports the correct x before and after the object create function, while method 2 works while reporting an incorrect x value both before and after the object create function.
As per method 1; why does Object Create not use the x variable as the Print() suggests and we instead need to reverse the For Loop (as per method 2) just to get the Object Create function to correctly use the desired x value as originally intended?