Greetings! Please see this sample:
input int numCandle=0; input double maxLowerWickSize=1.0; input double maxBodySize=1.0; input int Myperiod=15;
MqlRates rates[];
int OnInit()
{
ArraySetAsSeries(rates, true);
}
void OnDeinit(const int reason)
{
ArrayFree(rates);
}
void OnTick()
{bool resultM15=false; bool resultM30=false;
//- for PERIOD_M15
if(CopyRates(Symbol(), PERIOD_M15, 1, MyPeriod, rates)!=MyPeriod)
{Print("Error CopyRates errcode = ",GetLastError()); return;}
//code of function isBullPinBarType(...) ,included to OnTick()
if (rates[numCandle].high-rates[numCandle].close > 0) {
double upperWick = rates[numCandle].high-rates[numCandle].open;
double body = rates[numCandle].open-rates[numCandle].close;
double lowerWick = rates[numCandle].close-rates[numCandle].low;
double totalCandle = upperWick + body + lowerWick;
if (lowerWick > 0.0 && lowerWick <= totalCandle*maxLowerWickSize && body > 0.0 && body <= totalCandle*maxBodySize)
{resultM15=true;}
}
//- for PERIOD_M30
if(CopyRates(Symbol(), PERIOD_M30, 1, MyPeriod, rates)!=MyPeriod)
{Print("Error CopyRates errcode = ",GetLastError()); return;}
//code of function isBullPinBarType(...) ,included to OnTick()
if (rates[numCandle].high-rates[numCandle].close > 0) {
upperWick = rates[numCandle].high-rates[numCandle].open;
body = rates[numCandle].open-rates[numCandle].close;
lowerWick = rates[numCandle].close-rates[numCandle].low;
totalCandle = upperWick + body + lowerWick;
if (lowerWick > 0.0 && lowerWick <= totalCandle*maxLowerWickSize && body > 0.0 && body <= totalCandle*maxBodySize)
{resultM30=true;}
}
}
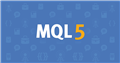
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I have an EA that draws rectangle on a specifically defined bearish pinbar followed by a bullish candle. Please see the code below. It basically shows the rectangle on the timeframe displayed on the chart.
How can I search for this candlestick pattern on timeframes within an H1 candlestick down to M2 in such a way that I can filter the pattern that has the longest bearish pinbar from all timeframes?