I use it like that all the time.
And i write it from the EA to the folder as FILE_BIN from a char array[];
FYI:
//--- open new file int filewritehandle = FileOpen(filename,FILE_READ|FILE_WRITE|FILE_BIN); //--- inject head css blah blah etc.. //--- inject javascript engine script script = "<script type = \"text/JavaScript\">\n"; FileSeek(filewritehandle,0,SEEK_END); FileWriteString(filewritehandle, script, StringLen(script)); FileSeek(filewritehandle,0,SEEK_END); FileWriteArray(filewritehandle,enginejs,0,ArraySize(enginejs)); script = "</script>\n"; FileSeek(filewritehandle,0,SEEK_END); FileWriteString(filewritehandle, script, StringLen(script)); //--- //+------------------------------------------------------------------+ char enginejs[] = {47,42,33,10,32,42,32,67,104,97,114,116,46,106,115,32,118,50,46,57,46,51,10,32,42,32,104,116,116,112, 115,58,47,47,119,119,119,46,99,104,97,114,116,106,115,46,111,114,103,10,32,42,32,40,99,41,32,50,48,49, 57,32,67,104,97,114,116,46,106,115,32,67,111,110,116,114,105,98,117,116,111,114,115,10,32,42,32,82,101,108, 101,97,115,101,100,32,117,110,100,101,114,32,116,104,101,32,77,73,84,32,76,105,99,101,110,115,101,10,32,42, 47,10,40,102,117,110,99,116,105,111,110,32,......
You can probably also just hard code it no i mean just put in a link to another source that serves the file in the html to save you the hassle.
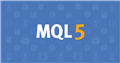
- www.mql5.com
I use it like that all the time.
And i write it from the EA to the folder as FILE_BIN from a char array[];
FYI:
You can probably also just hard code it no i mean just put in a link to another source that serves the file in the html to save you the hassle.
It is no problem to write a .html file with or without all the malware javascript you like embeded in it. It is not possible to write a .js file. Ah well... Buy high, sell low..
For anyone looking for solution:
//+------------------------------------------------------------------+ //| JavascriptTest.mq5 | //| Copyright 2019, MetaQuotes Software Corp. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2019, MetaQuotes Software Corp." #property link "https://www.mql5.com" #property version "1.00" #import "kernel32.dll" int MoveFileW(string ExistingFilename, string NewFilename); int CopyFileW(string strExistingFile, string strCopyOfFile, int OverwriteIfCopyAlreadyExists); int GetLastError(); #import //+------------------------------------------------------------------+ //| Script program start function | //+------------------------------------------------------------------+ void OnStart() { //--- const int handle2 = ::FileOpen("JavascriptFile.jsX", FILE_WRITE | FILE_TXT ); if (handle2 != INVALID_HANDLE) { FileWrite(handle2, "var data = 123; "); FileClose(handle2); } else { Print ("Write Error ", GetLastError()); } string FileFrom = "JavascriptFile.jsX"; if (FileIsExist(FileFrom)) Print (FileFrom, " Found!"); else Print (FileFrom, " not Found"); string PathFileFrom = TerminalInfoString(TERMINAL_DATA_PATH) + "\\MQL5\\Files\\" + FileFrom; string PathFileTo = TerminalInfoString(TERMINAL_DATA_PATH) + "\\MQL5\\Files\\" + "JavascriptFile.js"; Print (PathFileFrom); //MoveFileW(PathFileFrom, PathFileTo); //Print("MoveFileW error ",kernel32::GetLastError()); CopyFileW(PathFileFrom, PathFileTo,0); Print("CopyFileW error ",kernel32::GetLastError()); } //+------------------------------------------------------------------+

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Is this an (undocumented) MQL5 limitation or is my windows preventing writing files with .js extension?