... Unexpected token, probably type is missing? & Function already defined and has different type
class CZigZag : public CObject { <<< Open (1) private: void Calculate(); void AddExtremum(double value, datetime time); public: void Create(string symbol,int depth,int deviation,int backstep,ENUM_TIMEFRAMES timeframe=PERIOD_CURRENT); } <<< What is this? }; <<< Close (2)
I will not even try to check.
Seriously, you have all the information on the error messages. You can't hope for help posting snippets of code where the line numbers doesn't
appear.
If you want help, provide the file itself so it's possible to check directly.
I'm sorry for the mistake Sir, already corrected
Best regards
It could be that you have a typing error related to ; } { somewhere in your code, resulting in error messages about things further down your code.
Searched this many times and is not the current problem, but thanks a lot Mr.Windmill.
Best Regards
Thank you Mr. William, was my mistake tipping the relevant code to the forum, I apolagise for that, already corrected it.
Best Regards
Ferox875
Really still don't understand the problem ";" and "{" "}" are good, objects are well created, return type is void and the return is empty .
Any hints how to solve this?
Thanks in advance, best regards
CZigZag::Create(string symbol, … CZigZag::AddExtremum(double value, datetime time) // line 280No function type?
No function type?
Mr. William, you are so awesome so awesome, thank you very much!!!
Problem solved, thank you again Mr.William,
Best Regards
Ferox875
I also found the same error in codebase, can someone help me correct it, thank you~~
https://www.mql5.com/en/code/159
***
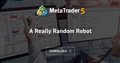
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello , in my ZigZag.mqh file when I compile I'm receive the following errors :
'Create' - unexpected token, probably type is missing? ZigZag.mqh 72 10
'Calculate' - unexpected token, probably type is missing? ZigZag.mqh 89 10
'AddExtremum' - unexpected token, probably type is missing? ZigZag.mqh 280 10
there is the object creation :
there are the functions wich give compiling error :
Already searched a lot about the errors but don't understand what is wrong here, hope someone more experienced can tell me what is missing or not right .
Thanks in advance, best regards
Ferox