Why do you copy ALL BARS on every tick? This is the BIGGEST ERROR!
if(CopyBuffer(DEMA_handle, 0, 0, rates_total, DEMA) <= 0) return(rates_total); ArraySetAsSeries(DEMA, true); if(CopyBuffer(DEMA_handle2, 0, 0, rates_total, DEMA2) <= 0) return(rates_total); ArraySetAsSeries(DEMA2, true); if(CopyLow(Symbol(), PERIOD_CURRENT, 0, rates_total, Low) <= 0) return(rates_total); ArraySetAsSeries(Low, true); if(CopyBuffer(ATR_handle, 0, 0, rates_total, ATR) <= 0) return(rates_total); ArraySetAsSeries(ATR, true); if(CopyHigh(Symbol(), PERIOD_CURRENT, 0, rates_total, High) <= 0) return(rates_total); ArraySetAsSeries(High, true); if(CopyTime(Symbol(), Period(), 0, rates_total, Time) <= 0) return(rates_total); ArraySetAsSeries(Time, true)
cryptadels: but was giving me some errors, wouldn't mind your help fixing it
- "Doesn't work" is meaningless — just like saying the car doesn't work. Doesn't start, won't go in gear, no electrical, missing the key, flat
tires — meaningless.
Do you really expect an answer with the information you've given? There are no mind readers here and our crystal balls are cracked. - Help you with what? You haven't stated a problem, you stated a want. Show us your attempt (using the CODE button)
and state the nature of your problem.
No free help 2017.04.21
Thanks for you help Vladimir, but am getting error after correcting as you pointed out. Pls can you check through and fix it as appropriate for me pls
cryptadels :
Thanks for you help Vladimir, but am getting error after correcting as you pointed out. Pls can you check through and fix it as appropriate for me pls
Please read the help. That's not difficult.
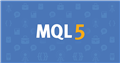
Documentation on MQL5: Technical Indicators / iDEMA
- www.mql5.com
//| Demo_iDEMA.mq5 | //| Copyright 2011, MetaQuotes Software Corp. | //| https://www.mql5.com | "The method of creation of the handle is set through the 'type' parameter (function type...

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Hi guys,
Am trying to fix this code but was giving me some errors, wouldn't mind your help fixing it
It does gives popup signals but shows all the previous signal concurrently with the present signal.
Thanks