Hey guys, can someone explain me the idea behind this code?
Can it not be simply replaced with this?
Help very much appreciated!
When the least significant digit of SYMBOL_TRADE_TICK_SIZE is a '1' (as in currencies market), NormalizeDouble(value, Digits()) will work so long as Digits() represent the right number of floating points.
So the function you showed is more like a "catch all" solution - so that the same code works for other markets.
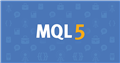
- www.mql5.com
-
Floating point has infinite number of decimals,
it's your not understanding floating point and that some numbers can't be represented exactly. (like 1/10.)
Double-precision floating-point format - Wikipedia, the free encyclopedia - Print out your values to the precision you want with DoubleToString - Conversion Functions - MQL4 Reference.
- SL/TP (stops) need to be normalized to tick size (not Point.) (On 5Digit Broker Stops are only allowed to be placed on full pip values. How to find out in mql? - MQL4 programming forum) and abide by the limits Requirements and Limitations in Making Trades - Appendixes - MQL4 Tutorial and that requires understanding floating point equality Can price != price ? - MQL4 programming forum
- Open price for pending orders need to be adjusted. On Currencies, Point == TickSize, so you will get the same answer, but it won't work on Metals. So do it right: Trailing Bar Entry EA - MQL4 programming forum or Bid/Ask: (No Need) to use NormalizeDouble in OrderSend - MQL4 programming forum
- Lot size must also be adjusted to a multiple of LotStep and check against min and max. If that is not a power of 1/10 then NormalizeDouble is wrong. Do it right.
- MathRound() and NormalizeDouble() are rounding in a different way. Make it explicit.
MT4:NormalizeDouble - General - MQL5 programming forum
How to Normalize - Expert Advisors and Automated Trading - MQL5 programming forum
NormalizeDouble, It's use is usually wrong,
-
Floating point has infinite number of decimals,
it's your not understanding floating point and that some numbers can't be represented exactly. (like 1/10.)
Double-precision floating-point format - Wikipedia, the free encyclopedia - Print out your values to the precision you want with DoubleToString - Conversion Functions - MQL4 Reference.
- SL/TP (stops) need to be normalized to tick size (not Point.) (On 5Digit Broker Stops are only allowed to be placed on full pip values. How to find out in mql? - MQL4 programming forum) and abide by the limits Requirements and Limitations in Making Trades - Appendixes - MQL4 Tutorial and that requires understanding floating point equality Can price != price ? - MQL4 programming forum
- Open price for pending orders need to be adjusted. On Currencies, Point == TickSize, so you will get the same answer, but it won't work on Metals. So do it right: Trailing Bar Entry EA - MQL4 programming forum or Bid/Ask: (No Need) to use NormalizeDouble in OrderSend - MQL4 programming forum
- Lot size must also be adjusted to a multiple of LotStep and check against min and max. If that is not a power of 1/10 then NormalizeDouble is wrong. Do it right.
- MathRound() and NormalizeDouble() are rounding in a different way. Make it explicit.
MT4:NormalizeDouble - General - MQL5 programming forum
How to Normalize - Expert Advisors and Automated Trading - MQL5 programming forum
double NormalizePrice(double p) { double ts=SymbolInfoDouble(_Symbol, SYMBOL_TRADE_TICK_SIZE); return(NormalizDouble(p/ts,0)*ts); }NormalizeDouble() is optimized to handle rounding of edge numbers better than MathRound()
Normalization (rounding) is needed only after you do any math operation (+ - * /), as this introduces minor errors in the result. That includes calculating a takeprofit/stoploss/pending order price/lotsize, etc..
If no math operator is involved, then rounding is not needed.
For example:double bid=SymbolInfoDouble(_Symbol, SYMBOL_BID);There is no need to normalize here.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hey guys, can someone explain me the idea behind this code?
Can it not be simply replaced with this?
Help very much appreciated!