When placing MACD and RSI on the same window in MT5, they are automatically rescaled to fit on the same coordinate axis. I am trying to create an EA with these two indicators and need to points where they will intersect each other. However, I am unable to rescale the MACD to be on the same scale as the RSI in order to find the exact intersection points as would be found on the automatic rescaling of the software itself. I hope that makes sense...
Any help or advise will be greatly appreciated. Thanks in advance.
I will help you, now I’ll start writing an example of an adviser.
In the meantime, read the help (look carefully at the word "handle") :
int iRSI( string symbol, // symbol name ENUM_TIMEFRAMES period, // period int ma_period, // averaging period ENUM_APPLIED_PRICE applied_price // type of price or handle );
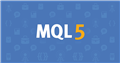
- www.mql5.com
Here's how we work with indicators manually:
- add the MACD indicator,
- add the RSI indicator (ATTENTION: add from the Navigator window !!!) -> drag the RSI indicator from the Navigator window to the MACD window,
- in the "Parameters" tab, select "Previous Indicator's Data")
And in the adviser, we act differently: we create the MACD handle, and when creating the RSI handle we write the MACD handle there:
//+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- create handle of the indicator iMACD handle_iMACD=iMACD(Symbol(),Period(),Inp_MACD_fast_ema_period,Inp_MACD_slow_ema_period, Inp_MACD_signal_period,Inp_MACD_applied_price); //--- if the handle is not created if(handle_iMACD==INVALID_HANDLE) { //--- tell about the failure and output the error code PrintFormat("Failed to create handle of the iMACD indicator for the symbol %s/%s, error code %d", Symbol(), EnumToString(Period()), GetLastError()); //--- the indicator is stopped early return(INIT_FAILED); } //--- create handle of the indicator iRSI handle_iRSI=iRSI(Symbol(),Period(),Inp_RSI_ma_period,handle_iMACD); //--- if the handle is not created if(handle_iRSI==INVALID_HANDLE) { //--- tell about the failure and output the error code PrintFormat("Failed to create handle of the iRSI indicator for the symbol %s/%s, error code %d", Symbol(), EnumToString(Period()), GetLastError()); //--- the indicator is stopped early return(INIT_FAILED); } //--- return(INIT_SUCCEEDED); }
Result (indicator Windows 3) :
The RSI is a bounded indicator, 0 to 100, while the MACD does not. There is therefore no point of comparison possible between the two since there is no common reference.
And now the main thing: the coefficient cannot be picked up - since this coefficient is floating, the coefficient changes all the time.
Look at an example: at first there seems to be an intersection of MACD and RSI, but if you move the chart, the intersection disappears
in my opinion, will it be optimal if there is a limit for MACD transactions at a value of -300 to 300 and added to the direction of the MACD trend? I think it will match the RSI from 0 to 100.
No, it will be a special case.
You can check it yourself: on different timeframes, on different symbols ...
Here's how we work with indicators manually:
- add the MACD indicator,
- add the RSI indicator (ATTENTION: add from the Navigator window !!!) -> drag the RSI indicator from the Navigator window to the MACD window,
- in the "Parameters" tab, select "Previous Indicator's Data")
And in the adviser, we act differently: we create the MACD handle, and when creating the RSI handle we write the MACD handle there:
Result (indicator Windows 3) :
These Values are not comparable at all.
Macd is in decimals but rsi id tooooo high.
How to fix it?
I don't care if crossover value changes on zoom in or out
i want the crossover according to the current zoom
These Values are not comparable at all.
Macd is in decimals but rsi id tooooo high.
How to fix it?
I don't care if crossover value changes on zoom in or out
i want the crossover according to the current zoom
Since both MACD and RSI are oscillators, it is possible to normalize both under another 3rd scale common to both.
// Normalize any array adjusting all its values to a range // between 0 and 1 (range will be decimal values between 0 and 1) // // // It is possible to chosse another range: // like 0 to 100, or -100 to 100, or -1 to 1, etc.. // // Only use this function if the initial array // contains values from an OSCILLATOR-kind indicator. // void NormalizadorDeArrays(double &a[]) { // -- Define the range interval of the final scale -- // here I choose a final range interval between 0 and 1 // But it can be 'd1 = -100;', and 'd2 = 100;' if a // scale ranging from -100 to +100 is desired. // double d1 = 0.0; // Minimum range of the final transposition scale double d2 = 1.0; // Maximum range of the final transposition scale double x_min; double x_max; x_min = a[ArrayMinimum(a)]; // index position which has the MAX value x_max = a[ArrayMaximum(a)]; // index position which has the MIN value // -------- sanity checks ---------------------- if(ArraySize(a) == 1) { printf("ALERT!!! -> You tried do normalize an array of 1 element? Need 2 or more elements!"); return; } if ((x_max - x_min) == 0) { printf("ALERT!!! -> Impossible to normalize! (Min value = Max value) !?"); return; } // --------------------------------------------- // All systems go! Lets make the normalization // for(int i = 0; i < ArraySize(a); i++) { a[i] = (((a[i] - x_min) * (d2 - d1)) / (x_max - x_min)) + d1; } // The result is the array itself, it read the array and the same existing // array range will be rewriten to itself, just converting it to such // normalized scale. Math relations between elements remais the same since // we are using this over an oscillator array. } //+------------------------------------------------------------------+

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
When placing MACD and RSI on the same window in MT5, they are automatically rescaled to fit on the same coordinate axis. I am trying to create an EA with these two indicators and need to points where they will intersect each other. However, I am unable to rescale the MACD to be on the same scale as the RSI in order to find the exact intersection points as would be found on the automatic rescaling of the software itself. I hope that makes sense...
Any help or advise will be greatly appreciated. Thanks in advance.