Forum on trading, automated trading systems and testing trading strategies
When you post code please use the CODE button (Alt-S)!
jackmql5:
The function below is intended to close a Long Position but it seems to open a new Sell order. How can it be modiffied to close a Long Position properly? Thanks.
Code:
//+------------------------------------------------------------------+ //| Simple adviser Open position.mq5 | //| Copyright © 2019, Vladimir Karputov | //| http://wmua.ru/slesar/ | //+------------------------------------------------------------------+ #property copyright "Copyright © 2019, Vladimir Karputov" #property link "http://wmua.ru/slesar/" #property version "1.000" //--- #include <Trade\Trade.mqh> CTrade m_trade; // trading object //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- LongPositionClose(void); } //+------------------------------------------------------------------+ //| Close Long position | //+------------------------------------------------------------------+ void LongPositionClose(void) { for(int i=PositionsTotal()-1; i>=0; i--) // returns the number of open positions if(m_position.SelectByIndex(i)) if(m_position.PositionType()==POSITION_TYPE_BUY) m_trade.PositionClose(m_position.Ticket()); } //+------------------------------------------------------------------+
Used Standard Library:
Trade class CTrade.
Method "Closes a position with the specified ticket." PositionClose
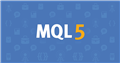
Documentation on MQL5: Standard Library / Trade Classes / CTrade
- www.mql5.com
Standard Library / Trade Classes / CTrade - Reference on algorithmic/automated trading language for MetaTrader 5
Thank you for the modified code. Now my position closes beautifully. And I am starting to like Class in MQL5 :)
Vladimir Karputov:
Code:
Used Standard Library:
Trade class CTrade.
Method "Closes a position with the specified ticket." PositionClose
Sergey Golubev:
Thank you for pointing out to me. I am getting more familiarise here.

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
The function below is intended to close a Long Position but it seems to open a new Sell order. How can it be modiffied to close a Long Position properly? Thanks.
//+------------------------------------------------------------------+
//| Close Long position |
//+------------------------------------------------------------------+
void LongPositionClose()
{
MqlTradeRequest mrequest; // Will be used for trade requests
MqlTradeResult mresult; // Will be used for results of trade requests
ZeroMemory(mrequest);
ZeroMemory(mresult);
double Ask = SymbolInfoDouble(_Symbol,SYMBOL_ASK); // Ask price
double Bid = SymbolInfoDouble(_Symbol,SYMBOL_BID); // Bid price
{
mrequest.action = TRADE_ACTION_DEAL; // Immediate order execution
mrequest.price = NormalizeDouble(Bid,_Digits); // Lastest Bid price
mrequest.sl = 0; // Stop Loss
mrequest.tp = 0; // Take Profit
mrequest.symbol = _Symbol; // Symbol
mrequest.volume = Lot; // Number of lots to trade
mrequest.magic = 0; // Magic Number
mrequest.type= ORDER_TYPE_SELL; // Sell order
mrequest.type_filling = ORDER_FILLING_IOC; // Order execution type
mrequest.deviation=5; // Deviation from current price
OrderSend(mrequest,mresult); // Send order
}
}