I was just curious: for debugging purposes I want some of Print() functions to be commented at once. Here's my approach that does not work:
Is it possible with MQL5?
PS: I've read https://www.mql5.com/en/articles/654 but want exactly above approach.
Thank's
Of course not.
#define DEBUG // -debug switcher, comment line for production #ifdef DEBUG Print("some debug info.."); #endif
- Comments are stripped before processing the tokens (#define) so no.
#ifdef DEBUG #define DB // This will not work, same in either case. #else #define DB #endif
#ifdef DEBUG #define DB true #else #define DB false #endif if(DB) Print("some debug info.."); else …
You can use a #define to switch logging on altogether like so:
#define DEBUG // comment out with '//' when done #ifdef DEBUG #define DebugPrint(s) Print(s) #else #define DebugPrint(s) #endif void OnTick() { ... DebugPrint("debug idx="+(string)idx); }
It will become even more advanced if you introduce a log level.
Usual ways takes too much typing, anyway thanks for your time!
William Roeder: all those "if" would stay in compiled code, even if DEBUG is not defined, correct? That would impact execution speed.
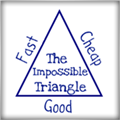
Should be removed at the optimization stage. Don't assume — profile it.
Usual ways takes too much typing, anyway thanks for your time!
The brute force solution. You'll get "some expression has no effect" warnings, but no need to change any line of code.
#define DEBUG // comment out with '//' when done #ifdef DEBUG #else #define Print false&&""==StringFormat #endif
The brute force solution. You'll get "some expression has no effect" warnings, but no need to change any line of code.
it does not compile with first line commented out:
//#define DEBUG // comment out with '//' when done #ifdef DEBUG #else #define Print false&&""==StringFormat #endif
'test.mq5' test.mq5 1 1 'DefineGlobal.mqh' DefineGlobal.mqh 1 1 'StringFormat' - wrong parameters count test.mq5 18 1 result of expression not used test.mq5 18 1 'StringFormat' - wrong parameters count test.mq5 19 1 result of expression not used test.mq5 19 1 'StringFormat' - wrong parameters count test.mq5 20 4 result of expression not used test.mq5 20 4
it does not compile with first line commented out:
Looks like I overlooked your answer, sorry.
Yes, unfortunately this doesn't compile in all places.
Try this instead:
#define Print while(false)Print
There's a small chance to break the code with statements like this
int i=0; do Print(i); while(++i<10);
But it's rather uncommon to occur.
Edit:
This looks better
#define DEBUG // comment out with '//' when done #ifdef DEBUG #else #define Print for(;false;)Print #endif
And the executable is getting smaller with the substitution - that means the optimizer was able to recognize and remove the empty statements.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I was just curious: for debugging purposes I want some of Print() functions to be commented at once. Here's my approach that does not work:
Is it possible with MQL5?
PS: I've read https://www.mql5.com/en/articles/654 but want exactly above approach.
Thank's