I guess the problem is: for an integer number a1 an expression like "copyopen_a1="+a1 means you're trying to add a number to a string. You can only add a string to a string (concatenation).
The Print function accepts a comma separated list of the arguments, so there's no need for this addition: Print("copyopen_a1=",a1); should work.
Use IntegerToString() for those cases where a conversion is really necessary.I guess the problem is: for an integer number a1 an expression like "copyopen_a1="+a1 means you're trying to add a number to a string. You can only add a string to a string (concatenation).
The Print function accepts a comma separated list of the arguments, so there's no need for this addition: Print("copyopen_a1=",a1); should work.
Use IntegerToString() for those cases where a conversion is really necessary."Print (" copyopen_a1 =" + a1);"The output is all strings because the + is used.
"Print (" copyopen_a1 =", a1);"The output is "copyopen_a1=" is a string, a1 is an integer,
Did I know that right?
Therefore, all numeric values must be cast to the string type.
Print("copyopen_a1 = ", IntegerToString(a1)); Print("copyopen_a2 = ", IntegerToString(a2)); Print("copyopen_a3 = ", IntegerToString(a3)); Print("copyopen_a4 = ", IntegerToString(a4)); Print("copyopen_a5 = ", IntegerToString(a5));
Or
Print("copyopen_a1 = " + (string)a1); Print("copyopen_a2 = " + (string)a2); Print("copyopen_a3 = " + (string)a3); Print("copyopen_a4 = " + (string)a4); Print("copyopen_a5 = " + (string)a5);
Additionally the PrintFormat() function needs the types specifically.
https://www.mql5.com/en/docs/common/printformat
type #
Type specifier is the only obligatory field for formatted output.
Symbol |
Type |
Output Format |
c |
int |
Symbol of short type (Unicode) |
C |
int |
Symbol of char type (ANSI) |
d |
int |
Signed decimal integer |
i |
int |
Signed decimal integer |
o |
int |
Unsigned octal integer |
u |
int |
Unsigned decimal integer |
x |
int |
Unsigned hexadecimal integer, using "abcdef" |
X |
int |
Unsigned hexadecimal integer, using "ABCDEF" |
e |
double |
A real value in the format [-] d.dddde[sign] ddd, where d - one decimal digit, dddd - one or more decimal digits, ddd - a three-digit number that determines the size of the exponent, sign - plus or minus |
E |
double |
Similar to the format of e, except that the sign of exponent is output by upper case letter (E instead of e) |
f |
double |
A real value in the format [-] dddd.dddd, where dddd - one or more decimal digits. Number of displayed digits before the decimal point depends on the size of number value. Number of digits after the decimal point depends on the required accuracy. |
g |
double |
A real value output in f or e format depending on what output is more compact. |
G |
double |
A real value output in F or E format depending on what output is more compact. |
a |
double |
A real number in format [−]0xh.hhhh p±dd, where h.hhhh – mantissa in the form of hexadecimal digits, using "abcdef", dd - One or more digits of exponent. Number of decimal places is determined by the accuracy specification |
A |
double |
A real number in format [−]0xh.hhhh P±dd, where h.hhhh – mantissa in the form of hexadecimal digits, using "ABCDEF", dd - One or more digits of exponent. Number of decimal places is determined by the accuracy specification |
s |
string |
String output |
Instead of PrintFormat() you can use printf().
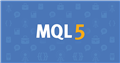
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
In addition, who determines the values of a1,a2,a3,a4, and a5?