Second parameter is not index but buffer number. There is no buffer 1 for iMA
Check here : https://www.mql5.com/en/docs/series/copybuffer
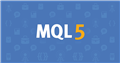
- www.mql5.com
Second parameter is not index but buffer number. There is no buffer 1 for iMA
Check here : https://www.mql5.com/en/docs/series/copybuffer
Thanks for getting back!
What you mean "There is no buffer 1 for iMA"?
I thought I bound a buffer via SetIndexBuffer(1, buffer_2, INDICATOR_DATA); (to index 1).
Or is it something that the iMA documentation says here - https://www.mql5.com/en/docs/indicators/ima - "... It has only one buffer..."?
How should I maintain multiple buffers of moving averages then?
I'm planning to make juicy calculations using those buffers, but also would like to display each.
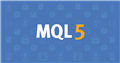
- www.mql5.com
I thought I bound a buffer via SetIndexBuffer(1, buffer_2, INDICATOR_DATA); (to index 1).
Or is it something that the iMA documentation says here - https://www.mql5.com/en/docs/indicators/ima - "... It has only one buffer..."?
Not your buffer_2. iMA has no buffer two — you already linked to the documentation — only one buffer. You are trying to
copy
iMA to your buffer_2.
Thanks, I thought the buffer index parameter of CopyBuffer referenced the target buffer index (the one I set with SetIndexBuffer).
Now I can see that it rather references the source - iMA -
buffer index. Which has only one, so it should be
0 at both call.
hello coders ;
sorry my english;
i have run time ERROR 4007 ;i think error come line141
i don't understand why handle3 get this ERROR in copybuffer() function
#property indicator_separate_window #property indicator_minimum 0 #property indicator_maximum 100 #property indicator_level1 20 #property indicator_level2 50 #property indicator_level3 80 #property indicator_buffers 9 #property indicator_plots 1 #property indicator_color1 clrAliceBlue //---- input parameters input int FastMA=12; input int SlowMA=24; input int Crosses=50; input ENUM_APPLIED_PRICE InpAppliedPrice=PRICE_CLOSE; // Applied price input ENUM_APPLIED_PRICE InpAppliedPrice2=PRICE_TYPICAL; // Applied price2 //---- buffers double MA[]; double MCD1[]; double MCD2[]; double MAfast[],MAslow[]; double Cross[]; double max_min[]; double PointDeviation[]; double PeriodTimeAVG[]; //---- var double smconst,ST,max,min; int ShiftFirstCross; int ShiftCrossesCross; int k,handle1,handle2,handle3,handle4; //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ void OnInit() { SetIndexBuffer(0, MA,INDICATOR_DATA); PlotIndexSetInteger(0,PLOT_DRAW_TYPE,DRAW_LINE); PlotIndexSetInteger(0,PLOT_LINE_STYLE,STYLE_SOLID); SetIndexBuffer(1, MCD1,INDICATOR_CALCULATIONS); SetIndexBuffer(1, MCD2,INDICATOR_CALCULATIONS); SetIndexBuffer(3, MAfast,INDICATOR_CALCULATIONS); SetIndexBuffer(4, MAslow,INDICATOR_CALCULATIONS); SetIndexBuffer(5, Cross,INDICATOR_CALCULATIONS); SetIndexBuffer(6, max_min,INDICATOR_CALCULATIONS); SetIndexBuffer(7, PointDeviation,INDICATOR_CALCULATIONS); SetIndexBuffer(8, PeriodTimeAVG,INDICATOR_CALCULATIONS); IndicatorSetString(INDICATOR_SHORTNAME,"cycle"); PlotIndexSetDouble(0,PLOT_EMPTY_VALUE,0.0); PlotIndexSetDouble(1,PLOT_EMPTY_VALUE,0.0); PlotIndexSetDouble(2,PLOT_EMPTY_VALUE,0.0); PlotIndexSetDouble(3,PLOT_EMPTY_VALUE,0.0); PlotIndexSetDouble(4,PLOT_EMPTY_VALUE,0.0); PlotIndexSetDouble(5,PLOT_EMPTY_VALUE,0.0); PlotIndexSetDouble(6,PLOT_EMPTY_VALUE,0.0); PlotIndexSetDouble(7,PLOT_EMPTY_VALUE,0.0); handle1=iMA(NULL,PERIOD_CURRENT,FastMA,0,MODE_SMA,PRICE_CLOSE); handle2=iMA(NULL,PERIOD_CURRENT,SlowMA,0,MODE_SMA,PRICE_CLOSE); handle3=iMA(NULL,PERIOD_CURRENT,FastMA,0,MODE_EMA,PRICE_TYPICAL); handle4=iMA(NULL,PERIOD_CURRENT,SlowMA,0,MODE_EMA,PRICE_TYPICAL); ShiftFirstCross=0; ShiftCrossesCross=0; k=0; max=0.; min=1000000.; } //+------------------------------------------------------------------+ //| Schaff Trend Cycle | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { int counted_bars=prev_calculated-1; int i,j,limit,NumberCross,BarsCross; double prev,MinMACD,MaxMACD,delta,Sum_max_min; if(rates_total<=SlowMA) return(-1); if(counted_bars>0) counted_bars--; limit=rates_total-counted_bars; if(limit>rates_total-SlowMA-1) limit=rates_total-SlowMA-1; //+------------------------------------------------------------------+ int to_copy; if(prev_calculated>rates_total || prev_calculated<0) to_copy=rates_total; else { to_copy=rates_total-prev_calculated; if(prev_calculated>0) to_copy++; } //+------------------------------------------------------------------+ //--- get handle1 buffer if(IsStopped()) return(0); //Checking for stop flag if(CopyBuffer(handle1,0,0,to_copy,MAfast)<=0) { Print("Getting handle1 is failed! Error",GetLastError()); return(0); } //--- get handle2 buffer if(IsStopped()) return(0); //Checking for stop flag if(CopyBuffer(handle2,0,0,to_copy,MAslow)<=0) { Print("Getting handle2 is failed! Error",GetLastError()); return(0); } //--- get handle3 buffererror if(IsStopped()) return(0); //Checking for stop flag if(CopyBuffer(handle3,0,0,to_copy,MCD1)<=0) { Print("Getting handle3 is failed! Error",GetLastError()); return(0); } //--- get handle4 buffererror if(IsStopped()) return(0); //Checking for stop flag if(CopyBuffer(handle4,0,0,to_copy,MCD2)<=0) { Print("Getting handle4 is failed! Error",GetLastError()); return(0); } ArraySetAsSeries(MAfast,true); ArraySetAsSeries(MAslow,true); ArraySetAsSeries(MCD1,true); ArraySetAsSeries(MCD2,true); //+------------------------------------------------------------------+ //--- not all data may be calculated int calculated=BarsCalculated(handle1); if(calculated<rates_total) { Print("Not all data of handle1 is calculated (",calculated,"bars ). Error",GetLastError()); return(0); } calculated=BarsCalculated(handle2); if(calculated<rates_total) { Print("Not all data of handle2 is calculated (",calculated,"bars ). Error",GetLastError()); return(0); } calculated=BarsCalculated(handle3); if(calculated<rates_total) { Print("Not all data of handle3 is calculated (",calculated,"bars ). Error",GetLastError()); return(0); } calculated=BarsCalculated(handle4); if(calculated<rates_total) { Print("Not all data of handle4 is calculated (",calculated,"bars ). Error",GetLastError()); return(0); } for(i=limit; i>0; i--) { Cross[i]=0.; if(MAfast[i]>=MAslow[i] && MAfast[i+1]<MAslow[i+1]) { if(ShiftFirstCross==0) ShiftFirstCross=i; if(ShiftCrossesCross==0) { k++; if(k==Crosses+1) ShiftCrossesCross=i; } Cross[i]=1.; max_min[i]=max-min; max=0.; min=1000000.; } else if(MAfast[i]<=MAslow[i] && MAfast[i+1]>MAslow[i+1]) { if(ShiftFirstCross==0) ShiftFirstCross=i; if(ShiftCrossesCross==0) { k++; if(k==Crosses+1) ShiftCrossesCross=i; } Cross[i]=-1.; max_min[i]=max-min; max=0.; min=1000000.; } else { if(max<high[i]) max=high[i]; if(min>low[i]) min=low[i]; } } if(limit>ShiftCrossesCross) limit=ShiftCrossesCross; for(i=limit; i>0; i--) { j=i; while(Cross[j]==0.) j++; NumberCross=0; BarsCross=0; Sum_max_min=0.; while(NumberCross<Crosses) { if(Cross[j]!=0.) { NumberCross++; Sum_max_min=Sum_max_min+max_min[j]; } j++; BarsCross++; } PeriodTimeAVG[i]=BarsCross/Crosses; PointDeviation[i]=NormalizeDouble(Sum_max_min/Crosses/2./_Point,0); } //+------------------------------------------------------------------+ for(i=limit; i>=0; i--) { MinMACD=(MCD1[i]-MCD2[i]); MaxMACD=(MCD1[i]-MCD2[i]); for(j=i+1; j<i+PeriodTimeAVG[i+1]; j++) { if((MCD1[j]-MCD2[j])<MinMACD) MinMACD=(MCD1[j]-MCD2[j]); if((MCD1[j]-MCD2[j])>MaxMACD) MaxMACD=(MCD1[j]-MCD2[j]); } delta=MaxMACD-MinMACD; if(delta==0.) ST=50.; else { ST=((MCD1[i]-MCD2[i])-MinMACD)/delta*100; } prev=MA[i+1]; MA[i]=(2./(1.+PeriodTimeAVG[i+1]/2.))*(ST-prev)+prev; } return(rates_total); }
Error:
SetIndexBuffer(1, MCD1,INDICATOR_CALCULATIONS); SetIndexBuffer(1, MCD2,INDICATOR_CALCULATIONS); SetIndexBuffer(3, MAfast,INDICATOR_CALCULATIONS);
Correct code
SetIndexBuffer(1, MCD1,INDICATOR_CALCULATIONS); SetIndexBuffer(2, MCD2,INDICATOR_CALCULATIONS); SetIndexBuffer(3, MAfast,INDICATOR_CALCULATIONS);
Error:
Correct code
Not your buffer_2. iMA has no buffer two — you already linked to the documentation — only one buffer. You are trying to copy iMA to your buffer_2.
Is it possible to have 10 handles in arrray[10] by for cycle?
for(uchar x = 0; x < ArraySize(pairs); x++)
{
switch(f)
{
case 4:
h[x] = iCustom(pairsT[x], PERIOD_CURRENT, "indicator_1", 1);
break;
case 5:
h[x] = iCustom(pairsT[x], PERIOD_CURRENT, "Valu indicator_2", r1);
break;
case 6:
h[x] = iCustom(pairsT[x], PERIOD_CURRENT, "Valu indicator_3", r1);
break;
case 7:
h[x] = iCustom(pairsT[x], PERIOD_CURRENT, "Examples\\indicator_4", 2);
etc.....

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I just created a simple indicator with two moving averages, but CopyBuffer for the second indicator keep failing, so the terminal shows only the first moving average line instead of both.
The code is pretty simple textbook example:
It always shows:
Please help, I'm trial and erroring for long hours now.