Hello guys, I'm new to coding EA. I wanna create an EA that can buy when all three moving averages are crossed over to the upside and the distance
between the FastMA (21 EMA) and SlowMA(50EMA) is at least 50 pips. The opposite is the same for selling condition. Another problem is that how
do I code so that the price will
buy at the FastMA(Whenever the price touches the FastMA) when all the conditions are met?
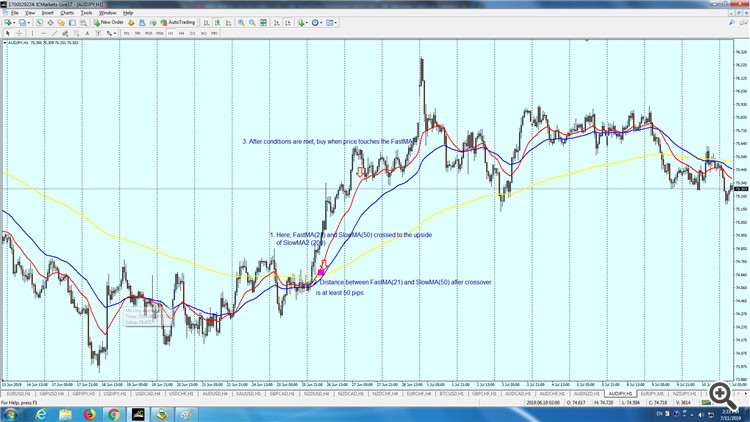
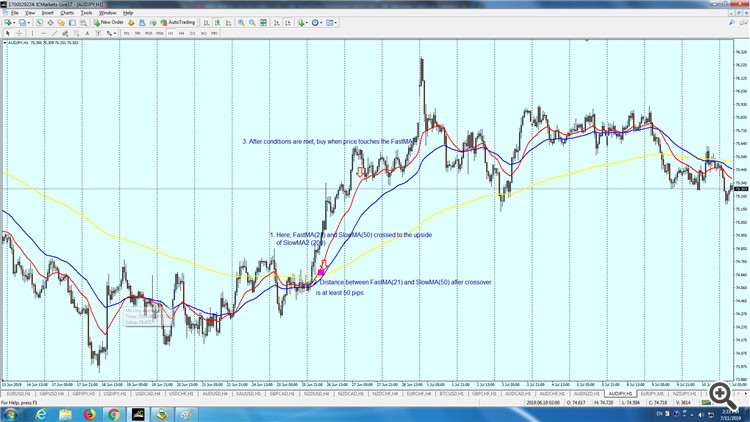
- HELP, please try to help me.
- HELP me with EA Coding ...
- Moving Average Cross over problem.
Your conditions for going into a trade are too strong:
if(PreviousFast<PreviousSlow && PreviousFast<PreviousSlow2 && PreviousSlow<PreviousSlow2)
if(CurrentFast>CurrentSlow && CurrentFast>CurrentSlow2 && CurrentSlow>CurrentSlow2)
See it? This will come true if a) fast MA crosses slow MA, b) fast MA crosses slow MA2 and c) slow MA crosses slow MA2 at the same tick. How
realistic is that.
I tried to change to the code below and it does not work as well.
if(PreviousFast<PreviousSlow && CurrentFast>CurrentSlow) if(pipsMABuy>=50) OrderEntry(0); if(PreviousFast>PreviousSlow && CurrentFast<CurrentSlow) if(pipsMABuy>=50) OrderEntry(0);
-
if(Bars==BarsOnChart)
For a new bar test, Bars is unreliable (a refresh/reconnect can change number of bars on chart,) volume is unreliable (miss ticks,) Price is unreliable (duplicate prices and The == operand. - MQL4 programming forum.) Always use time.
I disagree with making a new bar function, because it can only be called once per tick. A variable can be tested multiple times.
New candle - MQL4 programming forum -
double PreviousFast= iMA(NULL,0,FastMA,FastMaShift,FastMaMethod,FastMaAppliedTo,2);
Why did you post your MT4 question in the Root / MT5 EA section instead of the MQL4 section, (bottom of the Root page?)
General rules and best pratices of the Forum. - General - MQL5 programming forum
Next time post in the correct place. The moderators will likely move this thread there soon. -
pipsMABuy=(int)MathFloor(MathAbs(CurrentFast-CurrentSlow)/(_Point)); if(pipsMABuy>=50) OrderEntry(0);
A pip is not a point. Your test is now 50 points not 50 pips. - Price has touched fastMA when the Low <= fastMA and High >= fastMA.
Gary Hon:
I tried to change to the code below and it does not work as well.
I tried to change to the code below and it does not work as well.
Sure. What I meant is that you just check for the fast MA cross while the other 2 checks are reduced to a simple "need to be above" resp "need to be below". Like so:
if(PreviousFast<PreviousSlow /* && PreviousFast<PreviousSlow2 && PreviousSlow<PreviousSlow2 */ ) if(CurrentFast>CurrentSlow && CurrentFast>CurrentSlow2 && CurrentSlow>CurrentSlow2)
or if you clean it up a bit:
if( PreviousFast<PreviousSlow && CurrentFast>CurrentSlow && // fast MA upcross CurrentFast>CurrentSlow2 && CurrentSlow>CurrentSlow2 && // further MA conditions (CurrentFast-CurrentSlow2)/_Point>=pointDistanceBuy ) // at least x points distance OrderEntry(OP_BUY);
The check whether price touched fast MA is not required I think because if fast MA moves up, the price must have surpassed it since MAs always lag.

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register