If the above method is hard to explain or correct, I'm completely open to an entirely new approach.
Any help would be much appreciated, thanks.
Any help would be much appreciated, thanks.
- Just code it in MT4. No need for DLLs
-
Start using the new Event Handling Functions.
Event Handling Functions - Functions - Language Basics - MQL4 Reference
- Just code it in MT4. No need for DLLs
-
Start using the new Event Handling Functions.
Event Handling Functions - Functions - Language Basics - MQL4 Reference
Thank you for the response. However, Event handling functions won't do the trick. The project am working requires certain functions that is either not existent in mql4 or would be very difficult to implement. That's why I resorted to dll.
Check here : https://github.com/vdemydiuk/mtapi
You might find example(s) there how to do what you need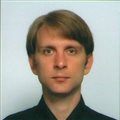
Check here : https://github.com/vdemydiuk/mtapi
You might find example(s) there how to do what you needThank you for the response. However, Event handling functions won't do the trick. The project am working requires certain functions that is either not existent in mql4 or would be very difficult to implement. That's why I resorted to dll.
There is not much that can't be done in mql. The main advantages of other languages is availability of libraries.
Anyway, you should think about switching to MT5/mql5 which allow to work directly with C# DLL now. Example.
There is not much that can't be done in mql. The main advantages of other languages is availability of libraries.
Anyway, you should think about switching to MT5/mql5 which allow to work directly with C# DLL now. Example.
Yes true the libraries are what makes the difference.
I'm trying to run a function written in C# on MT4 by means of a dll. The dll compiles correctly, however when I try to run it on MT4 i get an unresolved import call cannot find Add in TestMe.dll
Am following a guide on this link https://www.mql5.com/en/articles/249, the dll am using I downloaded it from the page as well. Funny enough when I run the same program using the dll already compiled on the site the code runs fine but when I compile the dll myself using the same code uploaded on the website that's when I start getting the error.
In case you were wondering, I did install the nugget package to enable using RGiesecke.DllExport, also I have the dll located in the mql4 libraries folder and I also enabled "Allow dll imports" in the script common tab.
I don't know if this bit is important but am using Visual Studio Enterprise 2015 and am using .Net Framework 4.5.2
//This is the C# code for the dll
//This is the MQL4 code for running the dll
this problem is very simple.
MT4-> https://c.mql5.com/3/256/Ekran_Alznt9sy.JPG
MT5->https://c.mql5.com/3/256/MT5DLL.JPG
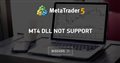
- 2018.12.04
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I'm trying to run a function written in C# on MT4 by means of a dll. The dll compiles correctly, however when I try to run it on MT4 i get an unresolved import call cannot find Add in TestMe.dll
Am following a guide on this link https://www.mql5.com/en/articles/249, the dll am using I downloaded it from the page as well. Funny enough when I run the same program using the dll already compiled on the site the code runs fine but when I compile the dll myself using the same code uploaded on the website that's when I start getting the error.
In case you were wondering, I did install the nugget package to enable using RGiesecke.DllExport, also I have the dll located in the mql4 libraries folder and I also enabled "Allow dll imports" in the script common tab.
I don't know if this bit is important but am using Visual Studio Enterprise 2015 and am using .Net Framework 4.5.2
//This is the C# code for the dll
//This is the MQL4 code for running the dll