Why i cant change the lot for the code, i have tried several times to change the lot from the code but it still appear 0.01 when i execute it in metatrader 5
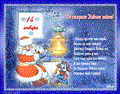
Блог слесаря-ремонтника и механика по наладке оборудования | Советы для ремонтников
- wmua.ru
С первого раза даже не поверил, что в таком небольшом чемоданчике можно разместить столько электроинструмента! В общем, как говорится, лучше один раз увидеть: С Праздником 8 Марта! С Праздником Весны! Милые дамы, женщины, мамы и бабушки. Поздравляю Вас с праздником весны, праздником жизни. Спасибо Вам за то, что Вы у нас есть. Спасибо Вам за...
- Scripts: Pending orders DOWN
- CAN ANYONE HELP TO CORRECT ERROR ON MY EA??????
- Multi pair indicators
Forum on trading, automated trading systems and testing trading strategies
When you post code please use the CODE button (Alt-S)!
Mjame116: Why i cant change the lot for the code, i
have tried several times to change the lot from the code but it still
appear 0.01 when i execute it in metatrader 5
-
When you post code please use the CODE button (Alt-S)!
(For large amounts of code, attach it.)
Please edit
your (original) post.
General rules and best pratices of the Forum. - General - MQL5 programming forum
Messages Editor - You tried? Where?
m_trade.BuyLimit(m_symbol.LotsMin(),m_symbol.NormalizePrice(price_ask),m_symbol.Name(), ... : m_trade.SellStop(m_symbol.LotsMin(),m_symbol.NormalizePrice(price_bid),m_symbol.Name(), ...
Is this about position size ?! A Lot is a brokers constant and cannot be changed.
Sergey Golubev:
Okay Done, Sorry i am newbie
whroeder1:
-
When you post code please use the CODE button (Alt-S)!
(For large amounts of code, attach it.)
Please edit
your (original) post.
General rules and best pratices of the Forum. - General - MQL5 programming forum
Messages Editor - You tried? Where?
input double InpLots = 5; // LotsI thought I put this values, so that my lot will be change.
whroeder1:
-
When you post code please use the CODE button (Alt-S)!
(For large amounts of code, attach it.)
Please edit
your (original) post.
General rules and best pratices of the Forum. - General - MQL5 programming forum
Messages Editor - You tried? Where?
m_trade.BuyLimit(m_symbol.LotsMax(),m_symbol.NormalizePrice(price_ask),m_symbol.Name(), m_trade.SellStop(m_symbol.LotsMax(),m_symbol.NormalizePrice(price_bid),m_symbol.Name(),I have change it to the max value and the lot size has being changed to the maximum, Thank yoo but can i know, how can i edit the code, so that i can put my desire lot to it. As an example, i want to put lot size of 2

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register