- Add Fibo level to an object
- Custom Indicator with many buffers
- Fibonacci EA
if you developed EA that displays fibo levels just change the percent for a new level in a similar line of code..
bool FiboLevelsSet(int levels, // количество линий уровня double &values[], // значения линий уровня color &colors[], // цвет линий уровня ENUM_LINE_STYLE &styles[], // стиль линий уровня int &widths[], // толщина линий уровня const long chart_ID=0, // ID графика const string name="FiboLevels") // имя объекта { //--- установим количество уровней ObjectSetInteger(chart_ID,name,OBJPROP_LEVELS,levels1); //--- установим свойства уровней в цикле for(int i=0;i<levels1;i++) { //--- значение уровня ObjectSetDouble(chart_ID,name,OBJPROP_LEVELVALUE,i,values1[i]); //--- цвет уровня ObjectSetInteger(chart_ID,name,OBJPROP_LEVELCOLOR,i,colors1[i]); //--- стиль уровня ObjectSetInteger(chart_ID,name,OBJPROP_LEVELSTYLE,i,styles1[i]); //--- толщина уровня ObjectSetInteger(chart_ID,name,OBJPROP_LEVELWIDTH,i,widths1[i]); //--- описание уровня ObjectSetString(chart_ID,name,OBJPROP_LEVELTEXT,i,DoubleToString(100*values1[i],1)); } //--- успешное выполнение return(true); }
long TestFibo(long n) { //--- первый член ряда Фибоначчи if(n<2) return(1); //--- все последующие члены вычисляются по этой формуле return(TestFibo(n-2)+TestFibo(n-1)); }
You need create object fibo, then add levels and define levels with value in percents.
You need create object fibo, then add levels and define levels with value in percents.
Ok but where you create? I see only objectset() ObjectCreate() is missing.
https://www.mql5.com/en/docs/constants/objectconstants/enum_object/obj_fibo
Level 78.6% will be 0.76% 1 percent = 100%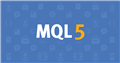
- www.mql5.com
https://www.mql5.com/en/docs/constants/objectconstants/enum_object/obj_fibo
Level 78.6% will be 0.76% 1 percent = 100%Ok and If I want to open position to a fibo level ? Shall I add last candle value with a fibo percent?
This not common practice of using fibolevels. Yes it's allow such creation.
Am i wrong or it is simply:
..after creation the fibo...
ObjectSetDouble(0, "Fibonacci", OBJPROP_LEVELVALUE, 0.786);
ObjectSetString(0, "Fibonacci", OBJPROP_LEVELTEXT, "78.6");
maybe i've missunderstand the question, hope it is usefull...
-----------------------------------------------------------------------------------------------------------------------
edit:
When you call the function above, it will erase the first Fibolevel i.e the "0 level";
i did not found a solution to add an new level, but ive found a method to edit an existing level:
ObjectSetDouble(0, "Fibonacci", OBJPROP_LEVELVALUE, 8, 0.786);
ObjectSetString(0, "Fibonacci", OBJPROP_LEVELTEXT, 8, "78.6");
it will takes the 8th level in my case the "423.6 level" witch is i mean useless, and set it to 78.6.
ty have fun.
--------------------------------------------------------------------------------------------------------------------------
edit edit:
ive finally found a solution to add a new level:
first of all we need to add an new Level with: ( 10 because we have allready 9 Levels )
ObjectSetInteger(0, "Fibonacci", OBJPROP_LEVELS, 10, 10);
then we mofier the Level like: ( 9 because the levels will count from 0 to 9 = 10.)
ObjectSetDouble(0, "Fibonacci", OBJPROP_LEVELVALUE, 9, 0.786);
ObjectSetString(0, "Fibonacci", OBJPROP_LEVELTEXT, 9, "78.6");
here we are..

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use