You need to use the ontimer event handler instead.
https://www.mql5.com/en/docs/event_handlers/ontimer
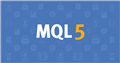
- www.mql5.com
Why? Nothing is changing on the chart. Return and wait for a new call to OnTick.
Hello guys;
I started to work with MQL5 in some months, and I have a question about the Sleep function.
I have a EA that needs to evaluate a condition every second during for exemple 1200 seconds, for this I use a while and the Sleep.
The problem is that when a use the while and the Sleep function to wait for some seconds, I can't get some values from the symbol like the bid, ask or volume, because the EA it's like "PAUSED" until the seconds is over.
Even if I put some code inside the while to calculate the prices for exemple this will not work.
There are some way to work around this?
Or maybe another way to make this verification every second?
Thanks.
Yes, there is!
I wrote a full example for you. You can use it on Indicators and/or on ExpertAdvisors.
In this example I created it on an Indicator. Load it on any chart window and observe the messages printed on the log tab.
//+------------------------------------------------------------------+ //| CustomTImers_Example.mq5 | //| Copyright 2018-2019, Rafael Prado Rocchi | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2018-2019, Rafael Prado Rocchi" #property link "https://www.mql5.com" #property version "1.00" #property indicator_chart_window #property indicator_plots 0 /* Full example of creating multiple Custom Timers, for executing commands or functions at various different intervals of time. This will NOT block like Sleep() does. In this example I am creating TWO Custom Timers: > one at 1 second inverval > another one at 5 seconds interval */ //We need to create a general control variable [for each of our timers]. double TimerControl_1sec = 0; // Control variable for the first timer double TimerControl_5sec = 0; // Control variable for the second timer //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit() { // Here we create a 500 milisecond MAIN timer. EventSetMillisecondTimer(500); // Main Timer created. return(INIT_SUCCEEDED); } //+---------------------------------------------------------+ //| Main Timer function, automatically runs every 500ms | //+---------------------------------------------------------+ void OnTimer() { //========= SubTimer: 1 second =================== // Put your code to run at every second inside it. // or call your functions inside it. // if (timer_1seg()) { Print("This message prints every 1 second"); } //========= SubTimer: 5 seconds ================== // Put your code to run every 5 seconds // or call your functions inside it. // if (timer_5seg()) { Print("I am printing every 5 seconds"); MyFunction(); // Or call your functions } } //+---------------------------------------------------------+ //| Example function | //+---------------------------------------------------------+ void MyFunction() { Print(__FUNCTION__, ": I was called"); } // =============== TIMER CONTROLLERS ======================== /* Timers Functions: When called, return TRUE if time elapsed since last called time is greater than it´s internal predefined time settings, or FALSE if time is not elapsed yet. Make use of GetTickCount(): The GetTickCount() function returns the number of milliseconds that elapsed since the system start. GetTickCount() is limited by the restrictions of the system timer. It´s value is stored as an unsigned integer, so it's overfilled every 49.7 days if the computer works uninterruptedly. PS: Our Custom Timers will NOT 'continue working normally' in case of GetTickCount() is overfilled. (After 49 days) But you can implement a check for this situation and reset the custom timers if you neeed to keep Metatreder opened without restarting it for more than 49 days. */ //+------------------------------------+ //| Custom Timer Controller: 1 second | //+------------------------------------+ bool timer_1seg() { int myTime = 1000; // 1000ms double now = GetTickCount(); bool res = false; if(TimerControl_1sec < now) { TimerControl_1sec = now + myTime; res = true; } return res; } //+------------------------------------+ //| Custom Timer Controller: 5 seconds | //+------------------------------------+ bool timer_5seg() { int myTime = 5000; // 5000ms double now = GetTickCount(); bool res = false; if(TimerControl_5sec < now) { TimerControl_5sec = now + myTime; res = true; } return res; } //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { //--- //--- return value of prev_calculated for next call return(rates_total); } //+------------------------------------------------------------------+
Hello guys;
I started to work with MQL5 in some months, and I have a question about the Sleep function.
I have a EA that needs to evaluate a condition every second during for exemple 1200 seconds, for this I use a while and the Sleep.
The problem is that when a use the while and the Sleep function to wait for some seconds, I can't get some values from the symbol like the bid, ask or volume, because the EA it's like "PAUSED" until the seconds is over.
Even if I put some code inside the while to calculate the prices for exemple this will not work.
There are some way to work around this?
Or maybe another way to make this verification every second?
Thanks.
Spread 2 < 0 ? do you mean Swap_1 , Swap_2 ,Swap_3 ?
What is the required function ? Triple Rollover Trading for positive swaps ?

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello guys;
I started to work with MQL5 in some months, and I have a question about the Sleep function.
I have a EA that needs to evaluate a condition every second during for exemple 1200 seconds, for this I use a while and the Sleep.
The problem is that when a use the while and the Sleep function to wait for some seconds, I can't get some values from the symbol like the bid, ask or volume, because the EA it's like "PAUSED" until the seconds is over.
Even if I put some code inside the while to calculate the prices for exemple this will not work.
There are some way to work around this?
Or maybe another way to make this verification every second?
Thanks.