Hi everyone,
I have an EA that I have written that works perfectly 99% of the time. Occasionally though, it will just not process a block of code. When this happens, it almost always follows a change in indicator, the most recent example is a flip in the PSAR value. I know that it is not executing the code because there are Print() instructions that are not executed and therefore not showing up in the experts tab. The EA is running on a VPS. The point at which the failure occurs is at the open of a new candle and the testing of the indicator values at the close of the previous candle. The most likely root cause is an error with one of the indicator values at the close of the last candle. No errors were thrown up although I don't have explicit error trapping in place at this point in the code. Has anyone experienced anything like this before? Can you suggest a way to troubleshoot this / error trap?
Thanks in advance
**EDIT**: I have 2 EA's, slight variations on each other, running on the same market on different charts. Both EAs failed to execute the code at the same time. The code is identical at this point in both EA's
seems like a coding problem. my best blind guess is : you're not comparing double values correctly. but who knows?
your code will help pinpointing the fail/error/whatever reason
seems like a coding problem. my best blind guess is : you're not comparing double values correctly. but who knows?
your code will help pinpointing the fail/error/whatever reason
Hi Code. Yes, I expect it is a coding problem :) What I am really struggling with is finding out why the code is failing. I don't believe it is a type mismatch in the comparison but if the variable used to store the indicator value isn't updated correctly, that could cause an unexpected problem. Below is the code to update the indicator variables. How do I check if each variable has been correctly updated? If the variable comparisons work 99% of the time, it suggests it is not a basic type mismatch error.
Thanks
double close1=iClose(Symbol(),0,bar); double close2=iClose(Symbol(),0,bar+1); double high1 = iHigh(Symbol(),0,bar); double low1 = iLow(Symbol(),0,bar); double open1 = iOpen(Symbol(),0,bar); double open2 = iOpen(Symbol(),0,bar+1); double supertrend1 =iCustom(Symbol(),0,SuperTrend_indicator_name,"v1.00, www.xaphod.com","________________________",ST_Period_1,ST_Multiplier_1,0,bar); double supertrend2 =iCustom(Symbol(),0,SuperTrend_indicator_name,"v1.00, www.xaphod.com","________________________",ST_Period_1,ST_Multiplier_1,0,bar+1); double ma_fast1=iMA(Symbol(),0,ma_fast_period,ma_fast_shift,ma_fast_method,ma_fast_apply_to,bar); double ma_fast2=iMA(Symbol(),0,ma_fast_period,ma_fast_shift,ma_fast_method,ma_fast_apply_to,bar+1); double ma_slow1=iMA(Symbol(),0,ma_slow_period,ma_slow_shift,ma_slow_method,ma_slow_apply_to,bar); double ma_slow2=iMA(Symbol(),0,ma_slow_period,ma_slow_shift,ma_slow_method,ma_slow_apply_to,bar+1); double psar1 = iCustom(Symbol(),0,"Parabolic",InpSARStep,InpSARMaximum,0,bar); double psar2 = iCustom(Symbol(),0,"Parabolic",InpSARStep,InpSARMaximum,0,bar+1);
Hi Code. Yes, I expect it is a coding problem :) What I am really struggling with is finding out why the code is failing. I don't believe it is a type mismatch in the comparison but if the variable used to store the indicator value isn't updated correctly, that could cause an unexpected problem. Below is the code to update the indicator variables. How do I check if each variable has been correctly updated? If the variable comparisons work 99% of the time, it suggests it is not a basic type mismatch error.
Thanks
So what is the value of bar in your code 0 or 1? If it is zero then one of the indicators that you depend upon may not be valid when a bar has not been determined.
So what is the value of bar in your code 0 or 1? If it is zero then one of the indicators that you depend upon may not be valid when a bar has not been determined.
Bar = 1
double supertrend1 =iCustom(Symbol(),0,SuperTrend_indicator_name,"v1.00, www.xaphod.com","________________________",ST_Period_1,ST_Multiplier_1,0,bar); double supertrend2 =iCustom(Symbol(),0,SuperTrend_indicator_name,"v1.00, www.xaphod.com","________________________",ST_Period_1,ST_Multiplier_1,0,bar+1);
Is that a variable or the indicator name?
Is that a variable or the indicator name?
Hi Victor,
It's an indicator name
Hi Victor,
It's an indicator name
https://docs.mql4.com/indicators/icustom
Please check the format. I think the error is when you are calling an variable in an custom indicator that doesn't exist, resulting to your problem.
I think green and red highlighted words are indicator names.
double supertrend1 =iCustom(Symbol(),0,SuperTrend_indicator_name,"v1.00, www.xaphod.com","________________________",ST_Period_1,ST_Multiplier_1,0,bar); double supertrend2 =iCustom(Symbol(),0,SuperTrend_indicator_name,"v1.00, www.xaphod.com","________________________",ST_Period_1,ST_Multiplier_1,0,bar+1);
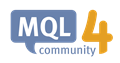
- docs.mql4.com
https://docs.mql4.com/indicators/icustom
Please check the format. I think the error is when you are calling an variable in an custom indicator that doesn't exist, resulting to your problem.
I think green and red highlighted words are indicator names.
Hi Victor,
The variable you are referring to are defined as a constant global variable, please see below.
string SuperTrend_indicator_name="xSuperTrend";
Is it possible that the MT4 has not finished the closing calculation for an indicator by the time I am requesting a new value. In effect, I am looking for a value which does not exist yet. If that is possible, how could I check for it or error trap it so it doesn't crash the system?
Thanks
Hi Victor,
The variable you are referring to are defined as a constant global variable, please see below.
Is it possible that the MT4 has not finished the closing calculation for an indicator by the time I am requesting a new value. In effect, I am looking for a value which does not exist yet. If that is possible, how could I check for it or error trap it so it doesn't crash the system?
Thanks
I think what Victor has pointed out has some merit. If those values after the name are default input values we can delete them and just leave the last two parameters like below.
double supertrend1 =iCustom(Symbol(),0,SuperTrend_indicator_name,0,bar); double supertrend2 =iCustom(Symbol(),0,SuperTrend_indicator_name,0,bar+1);
You could print the value of the indicator after it is computed to check if it is valid.
double supertrend1 =iCustom(Symbol(),0,SuperTrend_indicator_name,0,bar); Print("supertrend1 = ", supertrend1); double supertrend2 =iCustom(Symbol(),0,SuperTrend_indicator_name,0,bar+1); Print("supertrend2 = ", supertrend2);
The next time your EA fails just check your Expert tab to see if it got a valid value, I guess you already know this from your problem description, however, I do not see the failing Print commands in your code are they located in your indicators?.
Also, can we see your code that detects a new candle?

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi everyone,
I have an EA that I have written that works perfectly 99% of the time. Occasionally though, it will just not process a block of code. When this happens, it almost always follows a change in indicator, the most recent example is a flip in the PSAR value. I know that it is not executing the code because there are Print() instructions that are not executed and therefore not showing up in the experts tab. The EA is running on a VPS. The point at which the failure occurs is at the open of a new candle and the testing of the indicator values at the close of the previous candle. The most likely root cause is an error with one of the indicator values at the close of the last candle. No errors were thrown up although I don't have explicit error trapping in place at this point in the code. Has anyone experienced anything like this before? Can you suggest a way to troubleshoot this / error trap?
Thanks in advance
**EDIT**: I have 2 EA's, slight variations on each other, running on the same market on different charts. Both EAs failed to execute the code at the same time. The code is identical at this point in both EA's