//+------------------------------------------------------------------+ //| UpDownBars.mq4 | //| Copyright © 2011, MetaQuotes Software Corp. | //| http://www.metaquotes.net | //+------------------------------------------------------------------+ #property copyright "Copyright © 2011, MetaQuotes Software Corp." #property link "http://www.metaquotes.net" #property indicator_separate_window #property indicator_buffers 4 // Number of buffers #property indicator_color1 Aqua #property indicator_color2 Red #property indicator_color3 Blue #property indicator_color4 Blue #property indicator_width1 4 #property indicator_width2 4 #property indicator_width3 1 #property indicator_width4 1 extern int period = 20; double UpDown[]; double SignalUpDown[]; double Up[]; double Down[]; //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int init() { SetIndexBuffer(0,Up); SetIndexLabel(0,"Up"); SetIndexStyle (0,DRAW_HISTOGRAM); SetIndexBuffer(1,Down); SetIndexLabel(1,"Down"); SetIndexStyle (1,DRAW_HISTOGRAM); SetIndexBuffer(2,UpDown); SetIndexLabel(2,"UpDown"); SetIndexStyle (2,DRAW_NONE); SetIndexBuffer(3,SignalUpDown); SetIndexLabel(3,"SignalUpDown"); SetIndexStyle (3,DRAW_LINE); return(0); } //+------------------------------------------------------------------+ //| Custom indicator deinitialization function | //+------------------------------------------------------------------+ int deinit() { //---- //---- return(0); } //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int start() { if(Bars<=period) return(0); int i; int counted_bars=IndicatorCounted(); double bar, ups, downs, upsDivide, downsDivide; int upsCount, downsCount; int limit=Bars-counted_bars; ArrayResize(UpDown,limit); ArraySetAsSeries(UpDown,true); //---- macd counted in the 1-st buffer for(i=0; i<limit; i++) { ups = 0; downs = 0; bar = 0; upsCount = 0; downsCount = 0; upsDivide=0; downsDivide=0; for(int j=period;j>=0;j--) { bar=(Close[i+j]-Open[i+j])*100000; if(bar>0) { ups+=(MathSqrt(bar)*((period+1-j))); upsCount++; } if(bar<0) { downs+=(MathSqrt(-bar)*((period+1-j))); downsCount++; } } if(upsCount!=0) upsDivide = ups/upsCount; if(downsCount!=0) downsDivide = downs/downsCount; UpDown[i]=NormalizeDouble(((upsDivide)-(downsDivide)),3); if (NormalizeDouble(((upsDivide)-(downsDivide)),3) > 0) Up[i]=NormalizeDouble(((upsDivide)-(downsDivide)),3); else Up[i]=0; if (NormalizeDouble(((upsDivide)-(downsDivide)),3) < 0) Down[i]=NormalizeDouble(((upsDivide)-(downsDivide)),3);else Down[i]=0; } for(int ii=0; ii<limit; ii++) { SignalUpDown[ii]=iMAOnArray(UpDown,Bars,5,0,MODE_LWMA,ii); } ObjectsRedraw(); return(0); } //+------------------------------------------------------------------+I code follow you!
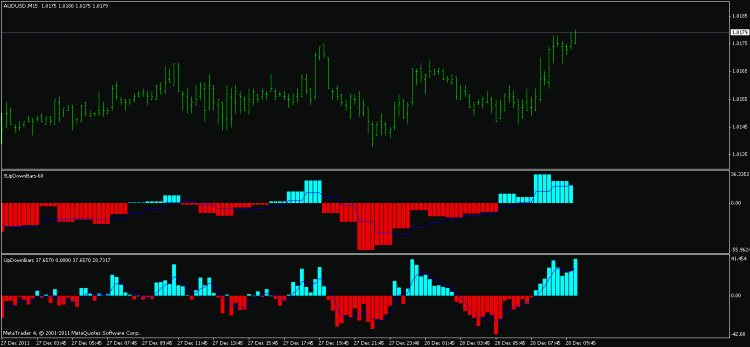
//+------------------------------------------------------------------+ //| !!UpDownBars.mq4 | //| Copyright © 2011, MetaQuotes Software Corp. | //| http://www.metaquotes.net | //+------------------------------------------------------------------+ #property copyright "Copyright © 2011, MetaQuotes Software Corp." #property link "http://www.metaquotes.net" #property indicator_separate_window #property indicator_buffers 4 #property indicator_color1 Aqua #property indicator_color2 Red #property indicator_color3 DeepPink #property indicator_color4 Blue #property indicator_width1 4 #property indicator_width2 4 #property indicator_width3 1 #property indicator_width4 1 //---- input parameters extern int GrossPeriod=60; extern int Barss=500; //---- buffers double ExtMapBuffer1[]; double ExtMapBuffer2[]; double ExtMapBuffer3[]; double ExtMapBuffer4[]; datetime daytimes[]; //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int init() { //---- indicators SetIndexStyle(0,DRAW_HISTOGRAM); SetIndexArrow(0,241); SetIndexBuffer(0,ExtMapBuffer1); SetIndexEmptyValue(0,0.0); SetIndexLabel(0,"upline"); SetIndexStyle(1,DRAW_HISTOGRAM); SetIndexArrow(1,242); SetIndexBuffer(1,ExtMapBuffer2); SetIndexEmptyValue(1,0.0); SetIndexLabel(1,"downline"); SetIndexStyle(2,DRAW_NONE); SetIndexArrow(2,159); SetIndexBuffer(2,ExtMapBuffer3); SetIndexEmptyValue(2,0.0); SetIndexLabel(2,"upstopline"); SetIndexStyle(3,DRAW_LINE); SetIndexArrow(3,159); SetIndexBuffer(3,ExtMapBuffer4); SetIndexEmptyValue(3,0.0); SetIndexLabel(3,"signalline"); IndicatorShortName("!!UpDownBars-"+GrossPeriod); //---- //---- if (Period()>GrossPeriod) {GrossPeriod=Period();} ArrayCopySeries(daytimes,MODE_TIME,Symbol(),GrossPeriod); return(0); } //+------------------------------------------------------------------+ //| Custor indicator deinitialization function | //+------------------------------------------------------------------+ int deinit() { //---- //---- return(0); } //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int start() { int counted_bars=IndicatorCounted(); int limit,bigshift; double value1,value2,value3,value4; if (counted_bars<0) return(-1); if (counted_bars>0) counted_bars--; if (Barss>0) limit=Barss-counted_bars; else limit=Bars-counted_bars; //---- for (int i=0; i<limit; i++) { if(Time[i]>=daytimes[0]) bigshift=0; else { bigshift = ArrayBsearch(daytimes,Time[i-1],WHOLE_ARRAY,0,MODE_DESCEND); if(Period()<=GrossPeriod) bigshift++; } for (int cnt=bigshift; cnt<(1+bigshift); cnt++) { value1=iCustom(NULL,GrossPeriod,"UpDownBars",0,cnt); value2=iCustom(NULL,GrossPeriod,"UpDownBars",1,cnt); value3=iCustom(NULL,GrossPeriod,"UpDownBars",2,cnt); value4=iCustom(NULL,GrossPeriod,"UpDownBars",3,cnt); } ExtMapBuffer1[i]=value1; ExtMapBuffer2[i]=value2; ExtMapBuffer3[i]=value3; ExtMapBuffer4[i]=value4; ObjectsRedraw(); } //---- return(0); } //+------------------------------------------------------------------+
"rockyhoangdn".... the chart with your code does not draw a new bar automatically... has to be refreshed manually... It also repaints a number of bars.
rplust:
"rockyhoangdn".... the chart with your code does not draw a new bar automatically... has to be refreshed manually... It also repaints a number of bars.
find: "rockyhoangdn".... the chart with your code does not draw a new bar automatically... has to be refreshed manually... It also repaints a number of bars.
ArrayResize(UpDown,limit);
and replace with
ArrayResize(UpDown,Bars);
rockyhoangdn:
rplust:
"rockyhoangdn".... the chart with your code does not draw a new bar automatically... has to be refreshed manually... It also repaints a number of bars.
find: "rockyhoangdn".... the chart with your code does not draw a new bar automatically... has to be refreshed manually... It also repaints a number of bars.
ArrayResize(UpDown,limit);
and replace with
ArrayResize(UpDown,Bars);
thanks, but this chart works fine... limit or Bars.. both work... I was referring to the other chart " Grossperiod 60" it does not auto refresh and also repaints. I'm not a coder but I guess it is possible to code it so it does not repaint history bars.
rplust:
thanks, but this chart works fine... limit or Bars.. both work... I was referring to the other chart " Grossperiod 60" it does not auto refresh and also repaints. I'm not a coder but I guess it is possible to code it so it does not repaint history bars.
rockyhoangdn:
rplust:
"rockyhoangdn".... the chart with your code does not draw a new bar automatically... has to be refreshed manually... It also repaints a number of bars.
find: "rockyhoangdn".... the chart with your code does not draw a new bar automatically... has to be refreshed manually... It also repaints a number of bars.
ArrayResize(UpDown,limit);
and replace with
ArrayResize(UpDown,Bars);
thanks, but this chart works fine... limit or Bars.. both work... I was referring to the other chart " Grossperiod 60" it does not auto refresh and also repaints. I'm not a coder but I guess it is possible to code it so it does not repaint history bars.
It will auto refresh with "Bars", but if use "Grossperiod 60" its allway repaint.

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
UpDownBars:
Author: Matus German