I just noticed the following code within PositionInfo.mqh
//+------------------------------------------------------------------+ //| Get the property value "POSITION_COMMISSION" | //+------------------------------------------------------------------+ double CPositionInfo::Commission(void) const { return(PositionGetDouble(POSITION_COMMISSION)); }
I cannot find the property POSITION_COMISSION listed in MQL5 documentation
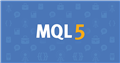
Documentation on MQL5: Constants, Enumerations and Structures / Trade Constants / Position Properties
- www.mql5.com
Position ticket. Unique number assigned to each newly opened position. It usually matches the ticket of an order used to open the position except when the ticket is changed as a result of service operations on the server, for example, when charging swaps with position re-opening. To find an order used to open a position, apply the...
Forum on trading, automated trading systems and testing trading strategies
Question about pending orders access
fxsaber, 2018.03.27 19:43
#include <MT4Orders.mqh> // https://www.mql5.com/en/code/16006 void OnStart() { for (int i = OrdersTotal() - 1; i >= 0; i--) if (OrderSelect(i, SELECT_BY_POS)) OrderPrint(); }
OrderSwap() and OrderCommission().
I have encountered the same problem.

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Hello. I made a simple script that prints information about positions and deals. I noticed that PositionGetDouble(POSITION_COMMISSION) does not return comission data, and HistoryDealGetDouble(_ticket,DEAL_SWAP) does not return swap data. Is there an easier way to get these data or am I doing something wrong?
Output of the above script where I highlighted the discrepancies