Hello all, hope this note finds you great!
I need to get the chart history data for multiple symbols in an EA, but I have to open the chart for each symbol at each timeframe manually and scroll the chart to the extent the data is available. I am trying to automate this process such that in MQL, we can force downloading all the available history data for a given symbol and timeframe. Any ideas on how this could be possible?
Thanks in advance!
Download history (suggest Dukascopy) and import into MT4, strongly suggest a separate Instance.
Hello all, hope this note finds you great!
I need to get the chart history data for multiple symbols in an EA, but I have to open the chart for each symbol at each timeframe manually and scroll the chart to the extent the data is available. I am trying to automate this process such that in MQL, we can force downloading all the available history data for a given symbol and timeframe. Any ideas on how this could be possible?
Thanks in advance!
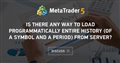
- 2018.03.01
- www.mql5.com
Your Broker's server will not contain the 'entire' history
Your Broker's server will not contain the 'entire' history
Of course I know it but OP wanted available broker's data history (by scrolling the chart. see the first post) not Dukascopy's history. I did code for this.
This will get him started
//+------------------------------------------------------------------+ //| TimeFrameUpdateAllCharts-A01.mq4 | //| Copyright 2018, rod178 | //| metdat@gmail.com | //+------------------------------------------------------------------+ // updates all timeframe on all charts // to update initialising cart placeduplice chart symbol #property copyright "Copyright © 2014 rod178" #property link "metdat@gmail.com" #property version "1.00" #property strict bool UseInitialChartTimeframe=true; long firstChartID = ChartFirst(); long initialChartID = ChartID(); string initialChartSymbol = ChartSymbol(initialChartID); int initialChartTimeframe = ChartPeriod(initialChartID); long previousChartID = firstChartID; long currentChartID = previousChartID; string currentChartSymbol = ChartSymbol(currentChartID); int currentChartTimeframe = ChartPeriod(currentChartID); int maxOpenCharts = 128; int i = 0; int timeframeArrayA[9]; int k; void OnStart() { timeframeArrayA[0] = PERIOD_M1; timeframeArrayA[1] = PERIOD_M5; timeframeArrayA[2] = PERIOD_M15; timeframeArrayA[3] = PERIOD_M30; timeframeArrayA[4] = PERIOD_H1; timeframeArrayA[5] = PERIOD_H4; timeframeArrayA[6] = PERIOD_D1; timeframeArrayA[7] = PERIOD_W1; timeframeArrayA[8] = PERIOD_MN1; long initialChartIDsame = initialChartID; string initialChartSymbolsame = initialChartSymbol; long previousChartIDsame = previousChartID; string currentChartSymbolsame = currentChartSymbol; long currentChartIDsame = currentChartID; bool symbolsSame = true; bool timeframeSame = true; while(i<maxOpenCharts) { if(ChartPeriod(currentChartIDsame) != ChartPeriod(ChartNext(currentChartIDsame)) && ChartPeriod(ChartNext(currentChartIDsame)) != 0) { timeframeSame=false; break; } currentChartIDsame = ChartNext(previousChartIDsame); if(currentChartIDsame<0){ break; } //end of chart list, currentChartID = -1 currentChartSymbolsame = ChartSymbol(currentChartIDsame); currentChartTimeframe = ChartPeriod(currentChartIDsame); previousChartIDsame =currentChartIDsame; Sleep(1000); i=i+1; } i=0; // while(i<maxOpenCharts && timeframeSame==false) while(i<maxOpenCharts) { if(currentChartID != initialChartID) { ChartSetSymbolPeriod(currentChartID,currentChartSymbol,timeframeArrayA[0]);Sleep(1000);WindowRedraw(); ChartSetSymbolPeriod(currentChartID,currentChartSymbol,timeframeArrayA[1]);Sleep(1000); ChartSetSymbolPeriod(currentChartID,currentChartSymbol,timeframeArrayA[2]);Sleep(1000); ChartSetSymbolPeriod(currentChartID,currentChartSymbol,timeframeArrayA[3]);Sleep(1000); ChartSetSymbolPeriod(currentChartID,currentChartSymbol,timeframeArrayA[4]);Sleep(1000); ChartSetSymbolPeriod(currentChartID,currentChartSymbol,timeframeArrayA[5]);Sleep(1000); ChartSetSymbolPeriod(currentChartID,currentChartSymbol,timeframeArrayA[6]);Sleep(1000); ChartSetSymbolPeriod(currentChartID,currentChartSymbol,timeframeArrayA[7]);Sleep(1000); ChartSetSymbolPeriod(currentChartID,currentChartSymbol,timeframeArrayA[8]);Sleep(1000); ChartSetSymbolPeriod(currentChartID,currentChartSymbol,currentChartTimeframe); //resets to initial TF } if(currentChartID != initialChartID && UseInitialChartTimeframe) { ChartSetSymbolPeriod(currentChartID,currentChartSymbol,initialChartTimeframe); } currentChartID = ChartNext(previousChartID); if(currentChartID<0){Print("break-->",currentChartID); break; } //end of chart list, currentChartID = -1 currentChartSymbol = ChartSymbol(currentChartID); currentChartTimeframe = ChartPeriod(currentChartID); previousChartID = currentChartID; Sleep(1000); i=i+1; } ChartNext(previousChartID); return; }
This will get him started
Here is my code (function) that does the job in my opinion but it is a part of a comprehensive class and therefore it must be edited for independent work first. But it serves as a good example.
bool cSymbol::LoadAllAvailableHistory(void) { long currID=ChartID(); ResetLastError(); long ID=ChartOpen(m_Symbol,m_TimeFrame); if(ID==0) { if(m_Verbose) printf("Opening the chart %s, %s failed ; Error: %s (%d)",m_Symbol,EnumToString(m_TimeFrame),ErrorDescription(_LastError),_LastError); return(false); } ChartRedraw(ID); ChartSetInteger(currID,CHART_BRING_TO_TOP,true); ChartRedraw(currID); ResetLastError(); if(!ChartSetInteger(ID,CHART_AUTOSCROLL,false)) { if(m_Verbose) printf("Switching off the autoscroll on the chart %s, %s failed ; Error: %s (%d)",m_Symbol,EnumToString(m_TimeFrame),ErrorDescription(_LastError),_LastError); return(false); } ChartRedraw(ID); ResetLastError(); if(!ChartSetInteger(ID,CHART_SCALE,0)) { if(m_Verbose) printf("Setting the scale on the chart %s, %s failed ; Error: %s (%d)",m_Symbol,EnumToString(m_TimeFrame),ErrorDescription(_LastError),_LastError); return(false); } ChartRedraw(ID); int jump=1000,countBars=0,count=0; bool res=false; uint start=GetTickCount(),timeout=4000,ms; do { ResetLastError(); res=ChartNavigate(ID,CHART_BEGIN,(-jump)*res); if(!res) { if(m_Verbose) printf("Navigate on the chart %s, %s failed ; Error: %s (%d)",m_Symbol,EnumToString(m_TimeFrame),ErrorDescription(_LastError),_LastError); } ChartRedraw(ID); Sleep(30); if(countBars==SeriesInfoInteger(m_Symbol,m_TimeFrame,SERIES_BARS_COUNT)) count++; else count=0; res&=count<7; countBars=(int)SeriesInfoInteger(m_Symbol,m_TimeFrame,SERIES_BARS_COUNT); ms=GetTickCount()-start; } while(res && ms<timeout && !IsStopped()); ChartClose(ID); if(m_Verbose) printf("Loading all available history for the symbol: %s (%s) has been successful",m_Symbol,EnumToString(m_TimeFrame)); return(true); }

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello all, hope this note finds you great!
I need to get the chart history data for multiple symbols in an EA, but I have to open the chart for each symbol at each timeframe manually and scroll the chart to the extent the data is available. I am trying to automate this process such that in MQL, we can force downloading all the available history data for a given symbol and timeframe. Any ideas on how this could be possible?
Thanks in advance!