Hi,
I am trying to link buttons states according each other.
When I press (true) a button, all the others are unpressed (false).
But it doesn't work.
Can you help me please ?
Regards
The easy way...
#property strict #include <Arrays\ArrayObj.mqh> #include <ChartObjects\ChartObjectsTxtControls.mqh> class ButtonCollection : public CArrayObj { public: CChartObjectButton* operator[](int i){ return this.At(i);} }; //+------------------------------------------------------------------+ ButtonCollection buttons; int OnInit() { int x = 0; CChartObjectButton *b; for(int i=0;i<10;i++) { b = new CChartObjectButton(); b.Create(0,string(i),0,x,100,100,100); b.Description(b.Name()); b.Color(clrGray); buttons.Add(b); x+=100; } return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ void OnTick(){} //+------------------------------------------------------------------+ void OnChartEvent(const int id, const long &lparam, const double &dparam, const string &sparam) { if(id==CHARTEVENT_OBJECT_CLICK) for(int i=buttons.Total()-1;i>=0;i--) if(sparam == buttons[i].Name()) for(int j=buttons.Total()-1;j>=0;j--) if(j!=i) buttons[j].State(false); } //+------------------------------------------------------------------+
The easy way...
Thank you.
I am looking at it.
The easy way...
For me i have used the official standard source code given by mql for creating the buttons :
//+------------------------------+ //| tools | //+------------------------------+ bool TLR_Button_Create(const long chart_ID=0, // chart's ID const string name="TLR_Button_", // button name const int sub_window=0, // subwindow index const int xx=0, // X coordinate const int yy=0, // Y coordinate const int width=30, // button width const int height=30, // button height const ENUM_BASE_CORNER cornerr=CORNER_RIGHT_LOWER, // chart corner for anchoring const string text="TLR", // text const string font="Arial", // font const int font_size=7, // font size const color clr=clrBlack, // text color const color back_clr=C'236,233,216', // background color const bool state=false, // pressed/released const bool back=false, // in the background const bool selection=false, // highlight to move const bool hidden=true, // hidden in the object list const long z_order=0) // priority for mouse click //const color border_clr=clrNONE; // border color { //--- reset the error value ResetLastError(); //--- create the button if(ObjectFind(chart_ID,name)<0) { if(!ObjectCreate(chart_ID,name,OBJ_BUTTON,sub_window,0,0)) { Print(__FUNCTION__, ": failed to create the button! Error code = ",GetLastError()); return(false); } //--- set button coordinates ObjectSetInteger(chart_ID,name,OBJPROP_XDISTANCE,xx); ObjectSetInteger(chart_ID,name,OBJPROP_YDISTANCE,yy); //--- set button size ObjectSetInteger(chart_ID,name,OBJPROP_XSIZE,width); ObjectSetInteger(chart_ID,name,OBJPROP_YSIZE,height); //--- set the chart's corner, relative to which point coordinates are defined ObjectSetInteger(chart_ID,name,OBJPROP_CORNER,cornerr); //--- set the text ObjectSetString(chart_ID,name,OBJPROP_TEXT,text); //--- set text font ObjectSetString(chart_ID,name,OBJPROP_FONT,font); //--- set font size ObjectSetInteger(chart_ID,name,OBJPROP_FONTSIZE,font_size); //--- set text color ObjectSetInteger(chart_ID,name,OBJPROP_COLOR,clr); //--- set background color ObjectSetInteger(chart_ID,name,OBJPROP_BGCOLOR,back_clr); //--- display in the foreground (false) or background (true) ObjectSetInteger(chart_ID,name,OBJPROP_BACK,back); //--- set button state ObjectSetInteger(chart_ID,name,OBJPROP_STATE,state); //--- enable (true) or disable (false) the mode of moving the button by mouse ObjectSetInteger(chart_ID,name,OBJPROP_SELECTABLE,selection); ObjectSetInteger(chart_ID,name,OBJPROP_SELECTED,selection); //--- hide (true) or display (false) graphical object name in the object list ObjectSetInteger(chart_ID,name,OBJPROP_HIDDEN,hidden); //--- set the priority for receiving the event of a mouse click in the chart ObjectSetInteger(chart_ID,name,OBJPROP_ZORDER,z_order); //--- set border color //ObjectSetInteger(chart_ID,name,OBJPROP_BORDER_COLOR,border_clr); //--- successful execution } return(true); } //+------------------------------------------------------------------+
Your way is very good.
I have tested it and it works very well.
I have to re-read it to understand it now.
Thank you very much.
The easy way...
When i change the time frame, the pressed button's state change to false (unpressed).
How can i fix it please ?
I just tried to add a color background and the editor told me that it's undefined .... why ? is "ColorBackground" not an inside keyword ?
b.ColorBackground(clrGold);
I have tried another way to get the same result by creatin the buttons in a loop, without "include" and "class".
void _Buttons_OutChart_Panel() { int _XPOSITION = 0; for(int _i=0;_i<4;_i++) // Creating Buttons in a loop { _XPOSITION+=_XSIZE_M; _Button_Create(0, string(_i), _Panel_Subwindow, _XPOSITION, _YPOSITION_Line1, _XSIZE_M,_YSIZE, _Panel_Corner_Buttons, string(_i), Font, SizeFont, _Button_Color_Unpressed_Font, _Button_Color_Unpressed_Background, false, false, false, true, 1); } }
from this mql given code :
bool _Button_Create(const long chart_ID=0, // chart's ID const string name="_Button_", // button name const int sub_window=0, // subwindow index const int xx=0, // X coordinate const int yy=0, // Y coordinate const int width=30, // button width const int height=30, // button height const ENUM_BASE_CORNER cornerr=CORNER_RIGHT_LOWER, // chart corner for anchoring const string text="", // text const string font="Arial", // font const int font_size=7, // font size const color clr=clrBlack, // text color const color back_clr=C'236,233,216', // background color const bool state=false, // pressed/released const bool back=false, // in the background const bool selection=false, // highlight to move const bool hidden=true, // hidden in the object list const long z_order=0) // priority for mouse click //const color border_clr=clrNONE; // border color { //--- reset the error value ResetLastError(); //--- create the button if(ObjectFind(chart_ID,name)<0) { if(!ObjectCreate(chart_ID,name,OBJ_BUTTON,sub_window,0,0)) { Print(__FUNCTION__, ": failed to create the button! Error code = ",GetLastError()); return(false); } //--- set button coordinates ObjectSetInteger(chart_ID,name,OBJPROP_XDISTANCE,xx); ObjectSetInteger(chart_ID,name,OBJPROP_YDISTANCE,yy); //--- set button size ObjectSetInteger(chart_ID,name,OBJPROP_XSIZE,width); ObjectSetInteger(chart_ID,name,OBJPROP_YSIZE,height); //--- set the chart's corner, relative to which point coordinates are defined ObjectSetInteger(chart_ID,name,OBJPROP_CORNER,cornerr); //--- set the text ObjectSetString(chart_ID,name,OBJPROP_TEXT,text); //--- set text font ObjectSetString(chart_ID,name,OBJPROP_FONT,font); //--- set font size ObjectSetInteger(chart_ID,name,OBJPROP_FONTSIZE,font_size); //--- set text color ObjectSetInteger(chart_ID,name,OBJPROP_COLOR,clr); //--- set background color ObjectSetInteger(chart_ID,name,OBJPROP_BGCOLOR,back_clr); //--- display in the foreground (false) or background (true) ObjectSetInteger(chart_ID,name,OBJPROP_BACK,back); //--- set button state ObjectSetInteger(chart_ID,name,OBJPROP_STATE,state); //--- enable (true) or disable (false) the mode of moving the button by mouse ObjectSetInteger(chart_ID,name,OBJPROP_SELECTABLE,selection); ObjectSetInteger(chart_ID,name,OBJPROP_SELECTED,selection); //--- hide (true) or display (false) graphical object name in the object list ObjectSetInteger(chart_ID,name,OBJPROP_HIDDEN,hidden); //--- set the priority for receiving the event of a mouse click in the chart ObjectSetInteger(chart_ID,name,OBJPROP_ZORDER,z_order); //--- set border color //ObjectSetInteger(chart_ID,name,OBJPROP_BORDER_COLOR,border_clr); //--- successful execution } return(true); }
How can i get the desired state effect in a loop please ?
Thierry Ramaniraka:
hello,
I just tried to add a color background and the editor told me that it's undefined .... why ? is "ColorBackground" not an inside keyword ?
b.ColorBackground(clrGold);
Can someone find a solution for this problem please ?
I am always searching a solution without success for now.
Can someone find a solution for this problem please ?
I am always searching a solution without success for now.
Perhaps you should try a tutorial on OOP...
b.ColorBackground(clrGold);
...is not an "inside keyword", it is a call to a class method that does not exist. A method does exist for your desired result and it's also documented.
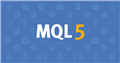
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I am trying to link buttons states according each other.
When I press (true) a button, all the others are unpressed (false).
But it doesn't work.
Can you help me please ?
Regards