Have you read examples form Code Base?
https://www.mql5.com/en/code/825
Debugging is tricky as MQ editor/compiler doesn't really report the errors yet.
But that is a simple indicator, I doubt you will benefit from GPU calculations.
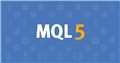
- votes: 29
- 2012.02.08
- MetaQuotes
- www.mql5.com
Have you read examples form Code Base?
https://www.mql5.com/en/code/825
Debugging is tricky as MQ editor/compiler doesn't really report the errors yet.
But that is a simple indicator, I doubt you will benefit from GPU calculations.
The GPU calculation what I am trying is not for the current values . It is for the historic data and applying some complex mathematical formula which requires huge processing capacity which GPU can give me. My CPU is working damn slow for those calculations and hence I am not able to figure the moves of the indicator.
Your CPU is faster than you thing, a single x86 core built in last 10 years running at 2 GHz can do at least 1 000 000 operations like those written in your code per second. Top end cores can do 10 even 20 000 000.
Have you written something already? I might try modifying this later, but don't promise anything.
Your CPU is faster than you thing, a single x86 core built in last 10 years running at 2 GHz can do at least 1 000 000 operations like those
written in your code per second. Top end cores can do 10 even 20 000 000.
Have you written something already? I might try modifying this later, but don't promise anything.
Hi... Have you modified it? I was looking for example. I am still in confusion to what you have said about speed.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Here is the code that I am trying to modify:
I am trying to modify an indicator file using the OpenCL library. But I couldn't understand how i can make that possible. I tried to read and understand the Documentation but could not. Kindly, help me modify the above with OpenCl, so that it will help me understand what I can do with it.