Hi, everything is OK about my EA. However whenever i put this protection code to my ea. It stopped using TP & SL!!
I have tried to use account verification code... please take a look below
If I remove these account verification codes then EA start to use TP & SL again! Don't get it!
Brackets. You guys wanna write code like gurus without being.
string broker = AccountInfoString(ACCOUNT_COMPANY); long account = AccountInfoInteger(ACCOUNT_LOGIN); printf("The name of the broker = %s", broker); printf("Account number = %d", account); if (broker == allowed_broker) { for (int i=0; i<ArraySize(allowed_accounts); i++) { if (account == allowed_accounts[i]) { password_status = 1; Print("EA account verified"); break; } } if (password_status == -1) Print("EA is not allowed to run on this account."); }
Brackets. You guys wanna write code like gurus without being.
Thanks a lot bro... however, still have the same problem... not using any SL & TP!!!
//+------------------------------------------------------------------+ //| Stappleton I //| //| Created by Black Obsidian //| Created at Mon Jan 01 05:47:00 GMT 2018 //| //| Tested on EURUSD_fhdb, H4, 01.01.2005 - 31.12.2017 //| Spread: 2.5, Slippage: 0.0, Min distance of stop from price: 5.0 //+------------------------------------------------------------------+ #property copyright "" #property link "" #property version "1.0" const string allowed_broker = "FXTM FT Global Ltd."; const long allowed_accounts[] = { 11499765, 11499764 }; int password_status = -1; //+------------------------------------------------------------------+ // -- SL/PT Parameters //+------------------------------------------------------------------+ extern string __s2 = "----- SL/PT Parameters ----------------------"; // this is the minimum and maximum value for PT & SL used, // any bigger or smaller value will be capped to this range extern double MinimumSLPT = 200; extern double MaximumSLPT = 500; //+------------------------------------------------------------------+ // -- Money Management Parameters //+------------------------------------------------------------------+ extern string __s6 = "----- Money Management Parameters -----------"; extern bool UseMoneyManagement = false; extern double Lots = 0.1; extern int LotsDecimals = 2; extern double RiskInPercent = 2.0; extern double MaximumLots = 0.5; extern bool UseFixedMoney = false; extern double RiskInMoney = 100.0; //+------------------------------------------------------------------+ // -- Trading Logic Settings //+------------------------------------------------------------------+ extern string __s7 = "----- Trading Logic Settings ----------------"; extern int MaxTradesPerDay = 0; // 0 means unlimited extern bool LimitSignalsToRange = false; extern string TimeRangeFrom = "08:00"; extern string TimeRangeTo = "16:00"; extern bool ExitAtEndOfRange = false; extern bool ExitAtEndOfDay = false; extern string ExitTimeEOD = "00:00"; extern bool ExitOnFriday = false; extern string ExitTimeOnFriday = "00:00"; extern bool TradeLong = true; extern bool TradeShort = true; //+------------------------------------------------------------------+ // -- Trading Date Parameters //+------------------------------------------------------------------+ extern string __s8 = "----- Trading Date Parameters ---------------"; extern bool TradeSunday = true; extern bool TradeMonday = true; extern bool TradeTuesday = true; extern bool TradeWednesday = true; extern bool TradeThursday = true; extern bool TradeFriday = true; extern bool TradeSaturday = true; //+------------------------------------------------------------------+ // -- Other Parameters //+------------------------------------------------------------------+ extern string __s9 = "----- Other Parameters ----------------------"; extern int MaxSlippage = 3; extern string CustomComment = "Stappleton I"; extern int MagicNumber = 12345; extern bool EmailNotificationOnTrade = false; extern bool DisplayInfoPanel = true; //+------------------------------------------------------------------+ // -- Other Hidden Parameters //+------------------------------------------------------------------+ int MinDistanceOfStopFromPrice = 5.0; double gPointPow = 0; double gPointCoef = 0; double gbSpread = 2.5; double brokerStopDifference = 0; string eaStopDifference = ""; double eaStopDifferenceNumber = 0; int lastHistoryPosChecked = 0; int lastHistoryPosCheckedNT = 0; string currentTime = ""; string lastTime = ""; bool tradingRangeReverted = false; string sqLastPeriod; bool sqIsBarOpen; int LabelCorner = 1; int OffsetHorizontal = 5; int OffsetVertical = 20; color LabelColor = White; int lastDeletedOrderTicket = -1; bool rettmp; bool ReplacePendingOrders = false; /** * add your own parameters that will be included in every EA * into file /code/CustomParametersMT4.mq4 */ //extern bool TradeOnHour1 = true; //+------------------------------------------------------------------+ // -- Functions //+------------------------------------------------------------------+ int start() { drawStats(); if(!customStart()) return(0); if(manageTrades()) { // we are within trading range hours (if set) //------------------------------------------- // ENTRY RULES // LONG: (WMA(67) Crosses Above SMA(11)) if(TradeLong) { bool LongEntryCondition = ((iMA(NULL, 0, 67, 0, MODE_LWMA, PRICE_CLOSE, 2) < iMA(NULL, 0, 11, 0, MODE_SMA, PRICE_CLOSE, 2)) && (iMA(NULL, 0, 67, 0, MODE_LWMA, PRICE_CLOSE, 1) > iMA(NULL, 0, 11, 0, MODE_SMA, PRICE_CLOSE, 1))); if(LongEntryCondition == true) { openPosition(1); } } // SHORT: (WMA(67) Crosses Below SMA(11)) if(TradeShort) { bool ShortEntryCondition = ((iMA(NULL, 0, 67, 0, MODE_LWMA, PRICE_CLOSE, 2) > iMA(NULL, 0, 11, 0, MODE_SMA, PRICE_CLOSE, 2)) && (iMA(NULL, 0, 67, 0, MODE_LWMA, PRICE_CLOSE, 1) < iMA(NULL, 0, 11, 0, MODE_SMA, PRICE_CLOSE, 1))); if(ShortEntryCondition == true) { openPosition(-1); } } } if(getMarketPosition() != 0) { manageStop(); } return(0); } //+------------------------------------------------------------------+ int init() { { //--- string broker = AccountInfoString(ACCOUNT_COMPANY); long account = AccountInfoInteger(ACCOUNT_LOGIN); printf("The name of the broker = %s", broker); printf("Account number = %d", account); if (broker == allowed_broker) { for (int i=0; i<ArraySize(allowed_accounts); i++) { if (account == allowed_accounts[i]) { password_status = 1; Print("EA account verified"); break; } } if (password_status == -1) Print("EA is not allowed to run on this account."); } //--- return(0); } Log("--------------------------------------------------------"); Log("Starting the EA"); double realDigits; if(Digits < 2) { realDigits = 0; } else if (Digits < 4) { realDigits = 2; } else { realDigits = 4; } gPointPow = MathPow(10, realDigits); gPointCoef = 1/gPointPow; double brokerStopDifferenceNumber = MarketInfo(Symbol(),MODE_STOPLEVEL)/MathPow(10, Digits); brokerStopDifference = gPointPow*brokerStopDifferenceNumber; eaStopDifferenceNumber = MinDistanceOfStopFromPrice/gPointPow; eaStopDifference = DoubleToStr(MinDistanceOfStopFromPrice, 2); Log("Broker Stop Difference: ",DoubleToStr(brokerStopDifference, 2),", EA Stop Difference: ",eaStopDifference); if(DoubleToStr(brokerStopDifference, 2) != eaStopDifference) { Log("WARNING! EA Stop Difference is different from real Broker Stop Difference, the backtest results in MT4 could be different from results of Genetic Builder!"); if(eaStopDifferenceNumber < brokerStopDifferenceNumber) { eaStopDifferenceNumber = brokerStopDifferenceNumber; } } string brokerSpread = DoubleToStr((Ask - Bid)*gPointPow, 2); string strGbSpread = DoubleToStr(gbSpread, 2); Log("Broker spread: ",brokerSpread,", Genetic Builder test spread: ",strGbSpread); if(strGbSpread != brokerSpread) { Log("WARNING! Real Broker spread is different from spread used in Genetic Builder, the backtest results in MT4 could be different from results of Genetic Builder!"); } if(TimeStringToDateTime(TimeRangeTo) < TimeStringToDateTime(TimeRangeFrom)) { tradingRangeReverted = true; Log("Trading range s reverted, from: ", TimeRangeFrom," to ", TimeRangeTo); } else { tradingRangeReverted = false; }
& need another help... I tried to use "time limit" however, it even worse than before!! EA stopped trading all together!! NEED HELP
//+------------------------------------------------------------------+ //| Stappleton I //| //| Created by Black Obsidian //| Created at Mon Jan 01 05:47:00 GMT 2018 //| //| Tested on EURUSD_fhdb, H4, 01.01.2005 - 31.12.2017 //| Spread: 2.5, Slippage: 0.0, Min distance of stop from price: 5.0 //+------------------------------------------------------------------+ #property copyright "" #property link "" #property version "1.0" //+------------------------------------------------------------------+ // -- SL/PT Parameters //+------------------------------------------------------------------+ extern string __s2 = "----- SL/PT Parameters ----------------------"; // this is the minimum and maximum value for PT & SL used, // any bigger or smaller value will be capped to this range extern double MinimumSLPT = 200; extern double MaximumSLPT = 500; //+------------------------------------------------------------------+ // -- Money Management Parameters //+------------------------------------------------------------------+ extern string __s6 = "----- Money Management Parameters -----------"; extern bool UseMoneyManagement = false; extern double Lots = 0.1; extern int LotsDecimals = 2; extern double RiskInPercent = 2.0; extern double MaximumLots = 0.5; extern bool UseFixedMoney = false; extern double RiskInMoney = 100.0; //+------------------------------------------------------------------+ // -- Trading Logic Settings //+------------------------------------------------------------------+ extern string __s7 = "----- Trading Logic Settings ----------------"; extern int MaxTradesPerDay = 0; // 0 means unlimited extern bool LimitSignalsToRange = false; extern string TimeRangeFrom = "08:00"; extern string TimeRangeTo = "16:00"; extern bool ExitAtEndOfRange = false; extern bool ExitAtEndOfDay = false; extern string ExitTimeEOD = "00:00"; extern bool ExitOnFriday = false; extern string ExitTimeOnFriday = "00:00"; extern bool TradeLong = true; extern bool TradeShort = true; //+------------------------------------------------------------------+ // -- Trading Date Parameters //+------------------------------------------------------------------+ extern string __s8 = "----- Trading Date Parameters ---------------"; extern bool TradeSunday = true; extern bool TradeMonday = true; extern bool TradeTuesday = true; extern bool TradeWednesday = true; extern bool TradeThursday = true; extern bool TradeFriday = true; extern bool TradeSaturday = true; //+------------------------------------------------------------------+ // -- Other Parameters //+------------------------------------------------------------------+ extern string __s9 = "----- Other Parameters ----------------------"; extern int MaxSlippage = 3; extern string CustomComment = "Stappleton I"; extern int MagicNumber = 12345; extern bool EmailNotificationOnTrade = false; extern bool DisplayInfoPanel = true; //+------------------------------------------------------------------+ // -- Other Hidden Parameters //+------------------------------------------------------------------+ int MinDistanceOfStopFromPrice = 5.0; double gPointPow = 0; double gPointCoef = 0; double gbSpread = 2.5; double brokerStopDifference = 0; string eaStopDifference = ""; double eaStopDifferenceNumber = 0; int lastHistoryPosChecked = 0; int lastHistoryPosCheckedNT = 0; string currentTime = ""; string lastTime = ""; bool tradingRangeReverted = false; string sqLastPeriod; bool sqIsBarOpen; int LabelCorner = 1; int OffsetHorizontal = 5; int OffsetVertical = 20; color LabelColor = White; int lastDeletedOrderTicket = -1; bool rettmp; bool ReplacePendingOrders = false; /** * add your own parameters that will be included in every EA * into file /code/CustomParametersMT4.mq4 */ //extern bool TradeOnHour1 = true; //+------------------------------------------------------------------+ // -- Functions //+------------------------------------------------------------------+ int start() { { string expire_date = "2018.15.04"; //<-- hard coded datetime datetime e_d = StrToTime(expire_date); if (CurTime() >= e_d) { Alert ("The trial version has been expired! Purchase from "); return(0); } // your normal code! return(0); } drawStats(); if(!customStart()) return(0); if(manageTrades()) { // we are within trading range hours (if set) //------------------------------------------- // ENTRY RULES // LONG: (WMA(67) Crosses Above SMA(11)) if(TradeLong) { bool LongEntryCondition = ((iMA(NULL, 0, 67, 0, MODE_LWMA, PRICE_CLOSE, 2) < iMA(NULL, 0, 11, 0, MODE_SMA, PRICE_CLOSE, 2)) && (iMA(NULL, 0, 67, 0, MODE_LWMA, PRICE_CLOSE, 1) > iMA(NULL, 0, 11, 0, MODE_SMA, PRICE_CLOSE, 1))); if(LongEntryCondition == true) { openPosition(1); } } // SHORT: (WMA(67) Crosses Below SMA(11)) if(TradeShort) { bool ShortEntryCondition = ((iMA(NULL, 0, 67, 0, MODE_LWMA, PRICE_CLOSE, 2) > iMA(NULL, 0, 11, 0, MODE_SMA, PRICE_CLOSE, 2)) && (iMA(NULL, 0, 67, 0, MODE_LWMA, PRICE_CLOSE, 1) < iMA(NULL, 0, 11, 0, MODE_SMA, PRICE_CLOSE, 1))); if(ShortEntryCondition == true) { openPosition(-1); } } } if(getMarketPosition() != 0) { manageStop(); } return(0); }
& need another help... I tried to use "time limit" however, it even worse than before!! EA stopped trading all together!! NEED HELP
//-------------------------------------------------------------------- // simple.mq4 // To be used as an example in MQL4 book. //-------------------------------------------------------------------- int Count=0; // Global variable //-------------------------------------------------------------------- int init() // Spec. funct. init() { Alert ("Function init() triggered at start");// Alert return; // Exit init() } //-------------------------------------------------------------------- int start() // Spec. funct. start() { double Price = Bid; // Local variable Count++; // Tick counter Alert("New tick ",Count," Price = ",Price);// Alert return; // Exit start() } //-------------------------------------------------------------------- int deinit() // Spec. funct. deinit() { Alert ("Function deinit() triggered at deinitialization"); // Alert return; // Exit deinit() } //--------------------------------------------------------------------
Re arrange your code.
//+------------------------------------------------------------------+ int init() { { //--- string broker = AccountInfoString(ACCOUNT_COMPANY); long account = AccountInfoInteger(ACCOUNT_LOGIN);
Re arrange your code.
Please check again. Here i am giving you full source code. I didn't find any error at all. But still EA not using any TP & SL. I need help :(
Please check again. Here i am giving you full source code. I didn't find any error at all. But still EA not using any TP & SL. I need help :(
I don't have MT4 installed, it won't compile. But for sure that is not good.
int init()
{
{
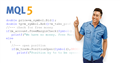
- www.mql5.com
I have fixed "time trial" prob :D & now EA does normal trading as usual... & it wasn't becoz of that bracket... it just had to be write down in lower part.. that's all...
2nd mission "Account activation" will see about that ;)
Thanks bro...
Yessssss... prob solved!!
I have fixed "time trial" prob :D & now EA does normal trading as usual... & it wasn't becoz of that bracket... it just had to be write down in lower part.. that's all...
2nd mission "Account activation" will see about that ;)
Thanks bro...
Duh.
Duh.
Great!!! I am fooled with it... its not solved!... its just showing alert correctly after time expired however, it doesn't stop EA from doing its trade! *sigh*
have to start again from the scratch!

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi, everything is OK about my EA. However whenever i put this protection code to my ea. It stopped using TP & SL!!
I have tried to use account verification code... please take a look below
If I remove these account verification codes then EA start to use TP & SL again! Don't get it!