#define EXPERT_MAGIC 123456 // MagicNumber of the expert
//+------------------------------------------------------------------+
//| Opening Buy position |
//+------------------------------------------------------------------+
void OnStart()
{
//--- declare and initialize the trade request and result of trade request
MqlTradeRequest request={0};
MqlTradeResult result={0};
//--- parameters of request
request.action =TRADE_ACTION_DEAL; // type of trade operation
request.symbol =Symbol(); // symbol
request.volume =0.1; // volume of 0.1 lot
request.type =ORDER_TYPE_BUY; // order type
request.price =SymbolInfoDouble(Symbol(),SYMBOL_ASK); // price for opening
request.deviation=5; // allowed deviation from the price
request.magic =EXPERT_MAGIC; // MagicNumber of the order
//--- send the request
if(!OrderSend(request,result))
PrintFormat("OrderSend error %d",GetLastError()); // if unable to send the request, output the error code
//--- information about the operation
PrintFormat("retcode=%u deal=%I64u order=%I64u",result.retcode,result.deal,result.order);
}
//+------------------------------------------------------------------+
Example of the TRADE_ACTION_DEAL trade operation for opening a Sell position:
#define EXPERT_MAGIC 123456 // MagicNumber of the expert
//+------------------------------------------------------------------+
//| Opening Sell position |
//+------------------------------------------------------------------+
void OnStart()
{
//--- declare and initialize the trade request and result of trade request
MqlTradeRequest request={0};
MqlTradeResult result={0};
//--- parameters of request
request.action =TRADE_ACTION_DEAL; // type of trade operation
request.symbol =Symbol(); // symbol
request.volume =0.2; // volume of 0.2 lot
request.type =ORDER_TYPE_SELL; // order type
request.price =SymbolInfoDouble(Symbol(),SYMBOL_BID); // price for opening
request.deviation=5; // allowed deviation from the price
request.magic =EXPERT_MAGIC; // MagicNumber of the order
//--- send the request
if(!OrderSend(request,result))
PrintFormat("OrderSend error %d",GetLastError()); // if unable to send the request, output the error code
//--- information about the operation
PrintFormat("retcode=%u deal=%I64u order=%I64u",result.retcode,result.deal,result.order);
}
//+------------------------------------------------------------------+
Example of the TRADE_ACTION_DEAL trade operation for closing positions: #define EXPERT_MAGIC 123456 // MagicNumber of the expert //+------------------------------------------------------------------+ //| Closing all positions | //+------------------------------------------------------------------+ void OnStart() { //--- declare and initialize the trade request and result of trade request MqlTradeRequest request; MqlTradeResult result; int total=PositionsTotal(); // number of open positions //--- iterate over all open positions for(int i=total-1; i>=0; i--) { //--- parameters of the order ulong position_ticket=PositionGetTicket(i); // ticket of the position string position_symbol=PositionGetString(POSITION_SYMBOL); // symbol int digits=(int)SymbolInfoInteger(position_symbol,SYMBOL_DIGITS); // number of decimal places ulong magic=PositionGetInteger(POSITION_MAGIC); // MagicNumber of the position double volume=PositionGetDouble(POSITION_VOLUME); // volume of the position ENUM_POSITION_TYPE type=(ENUM_POSITION_TYPE)PositionGetInteger(POSITION_TYPE); // type of the position //--- output information about the position PrintFormat("#%I64u %s %s %.2f %s [%I64d]", position_ticket, position_symbol, EnumToString(type), volume, DoubleToString(PositionGetDouble(POSITION_PRICE_OPEN),digits), magic); //--- if the MagicNumber matches if(magic==EXPERT_MAGIC) { //--- zeroing the request and result values ZeroMemory(request); ZeroMemory(result); //--- setting the operation parameters request.action =TRADE_ACTION_DEAL; // type of trade operation request.position =position_ticket; // ticket of the position request.symbol =position_symbol; // symbol request.volume =volume; // volume of the position request.deviation=5; // allowed deviation from the price request.magic =EXPERT_MAGIC; // MagicNumber of the position //--- set the price and order type depending on the position type if(type==POSITION_TYPE_BUY) { request.price=SymbolInfoDouble(position_symbol,SYMBOL_BID); request.type =ORDER_TYPE_SELL; } else { request.price=SymbolInfoDouble(position_symbol,SYMBOL_ASK); request.type =ORDER_TYPE_BUY; } //--- output information about the closure PrintFormat("Close #%I64d %s %s",position_ticket,position_symbol,EnumToString(type)); //--- send the request if(!OrderSend(request,result)) PrintFormat("OrderSend error %d",GetLastError()); // if unable to send the request, output the error code //--- information about the operation PrintFormat("retcode=%u deal=%I64u order=%I64u",result.retcode,result.deal,result.order); //--- } } } //+------------------------------------------------------------------+See: https://www.mql5.com/en/docs/constants/structures/mqltraderequest
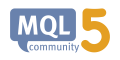
Documentation on MQL5: Standard Constants, Enumerations and Structures / Data Structures / Trade Request Structure
- www.mql5.com
Interaction between the client terminal and a trade server for executing the order placing operation is performed by using trade requests. The trade request is represented by the special predefined structure of MqlTradeRequest type, which contain all the fields necessary to perform trade deals. The request processing result is represented by...
At the bottom of that page.
hello
where and how can i use tp or sl un this script

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
I have all the script and code and parameters that i want the EA I am working on to abide by.
Only thing missing is the most important part, TELLING IT TO OPEN/CLOSE A TRADE! Lol
Does anyone know where i can find this information? Thanks