#define KEY_LEFT 37
#define KEY_UP 38
#define KEY_RIGHT 39
#define KEY_DOWN 40
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
void OnInit()
{
Comment("PRESS ' UP ARROW ' TO DISPLAY BOTH ORDERS"+
" "+"\nPRESS ' DOWN ARROW ' TO CLOSE BOTH ORDERS"
" "+"\nPRESS ' LEFT ARROW ' TO CLOSE BUY AND PLACE NEW BUY ORDERS"
" "+"\nPRESS ' RIGHT ARROW ' TO CLOSE SELL AND PLACE NEW SELL ORDERS"
" "+"\nMOUSE CLICK ON CHART TO PLACE BUY OR SELL ORDER"
);
}
//+------------------------------------------------------------------+
//| ChartEvent function |
//+------------------------------------------------------------------+
bool Points;
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
void OnChartEvent(const int id,
const long &lparam,
const double &dparam,
const string &sparam)
{
if(id==CHARTEVENT_CLICK)
{
int result_message=MessageBox("BUY ORDER TO BE PLACED PRESS YES ELSE PRESS NO FOR SELL ORDER ?","Warning",MB_YESNOCANCEL);
if(result_message==IDYES)
{
if(OrdersTotal()<2)
{
Points=OrderSend(Symbol(),0,0.1,OrderOpenPrice(),3,0,0,"AUTO BUY",0,0,clrNONE);
OrderPrint();
GlobalVariableSet("BUY VOLUME",OrderLots());
}
}
if(result_message==IDNO)
{
if(OrdersTotal()<2)
{
Points=OrderSend(Symbol(),1,0.1,OrderOpenPrice(),3,0,0,"AUTO SELL",0,0,clrNONE);
OrderPrint();
GlobalVariableSet("SELL VOLUME",OrderLots());
}
}
if(OrdersTotal()>=2 && result_message!=IDCANCEL)
{
MessageBox("NOT POSSIBLE TO PLACE ORDER THERE IS 2 OR MORE ORDERS PRESENT");
}
}
if(id==CHARTEVENT_KEYDOWN)
{
if(lparam==KEY_UP)
{
BOTH_ORDERS_EXECUTED();
}
if(lparam==KEY_DOWN)
{
CLOSE_ALL_RECORDS();
}
if(lparam==KEY_LEFT)
{
BUY_CLOSE_THEN_NEW_BUY();
}
if(lparam==KEY_RIGHT)
{
SELL_CLOSE_THEN_SELL();
}
}
}
//+------------------------------------------------------------------+
void BOTH_ORDERS_EXECUTED()
{
if(OrdersTotal()==0)
{
GlobalVariableSet(AccountNumber(),AccountBalance()+10);
Points=OrderSend(Symbol(),0,0.1,OrderOpenPrice(),3,0,0,"AUTO BUY",0,0,clrNONE);
OrderPrint();
GlobalVariableSet("BUY VOLUME",OrderLots());
Points=OrderSend(Symbol(),1,0.1,OrderOpenPrice(),3,0,0,"AUTO SELL",0,0,clrNONE);
OrderPrint();
GlobalVariableSet("SELL VOLUME",OrderLots());
}
if(OrdersTotal()>0)
{MessageBox("WILL NOT EXECUTE THERE IS "+OrdersTotal()+" RECORD");}
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
void CLOSE_ALL_RECORDS()
{
if(AccountProfit()>0)
{
Points=OrderSelect(0,SELECT_BY_POS);
int TN1=OrderTicket();
Points=OrderSelect(1,SELECT_BY_POS);
int TN2=OrderTicket();
Points=OrderCloseBy(TN1,TN2,clrNONE);
OrderPrint();
}
if(AccountProfit()<0)
{MessageBox("NOT SATISFIED");}
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
void BUY_CLOSE_THEN_NEW_BUY()
{
double BV;
Points=OrderSelect(0,SELECT_BY_POS);
if(OrderProfit()>=10 && OrderType()==OP_BUY)
{
BV=OrderLots();
Points=OrderClose(OrderTicket(),OrderLots(),OrderClosePrice(),3,clrNONE);
OrderPrint();
Points=OrderSend(Symbol(),0,BV-0.01,OrderOpenPrice(),3,0,0,"AUTO BUY",0,0,clrNONE);
OrderPrint();
}
//----------------------------------------------
Points=OrderSelect(1,SELECT_BY_POS);
if(OrderProfit()>=10 && OrderType()==OP_BUY)
{
BV=OrderLots();
Points=OrderClose(OrderTicket(),OrderLots(),OrderClosePrice(),3,clrNONE);
OrderPrint();
Points=OrderSend(Symbol(),0,BV-0.01,OrderOpenPrice(),3,0,0,"AUTO BUY",0,0,clrNONE);
OrderPrint();
}
if(OrderProfit()<10)
{MessageBox("CONDITION NOT MET FOR BUY ORDER");}
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
void SELL_CLOSE_THEN_SELL()
{
double VS;
Points=OrderSelect(0,SELECT_BY_POS);
if(OrderProfit()>=10 && OrderType()==OP_SELL)
{
VS=OrderLots();
Points=OrderClose(OrderTicket(),OrderLots(),OrderClosePrice(),3,clrNONE);
OrderPrint();
Points=OrderSend(Symbol(),1,VS-0.01,OrderOpenPrice(),3,0,0,"AUTO SELL",0,0,clrNONE);
OrderPrint();
}
//---------------------------------------------------------------------
Points=OrderSelect(1,SELECT_BY_POS);
if(OrderProfit()>=10 && OrderType()==OP_SELL)
{
VS=OrderLots();
Points=OrderClose(OrderTicket(),OrderLots(),OrderClosePrice(),3,clrNONE);
OrderPrint();
Points=OrderSend(Symbol(),1,VS-0.01,OrderOpenPrice(),3,0,0,"AUTO SELL",0,0,clrNONE);
OrderPrint();
}
MessageBox("CONDITION NOT MET FOR SELL ORDER");
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
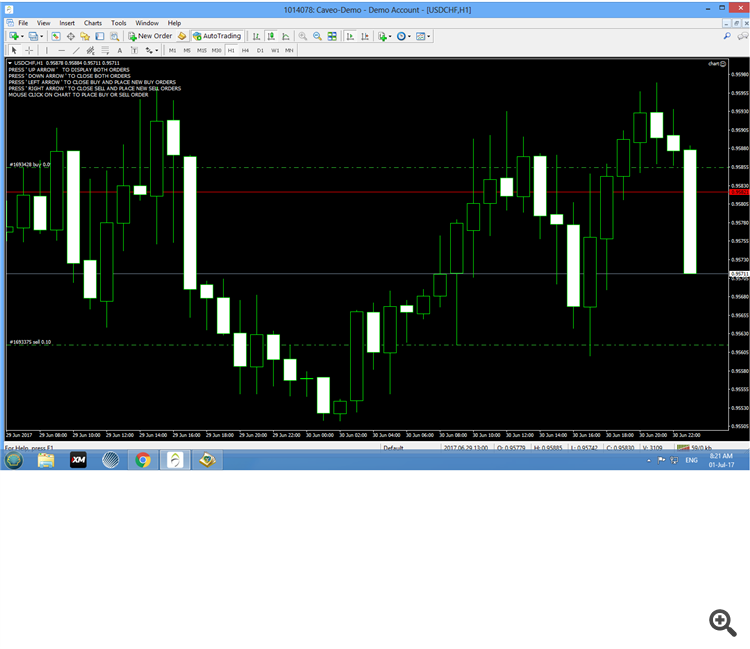
Files:
MQL_4_STEPS.txt
7 kb
IMAGE.png
94 kb
- Function to continually close positions per ticket per conditions
- Help me fix this code pls
- Help me please about code MT4 Button
Hello,
Something doesn't look right here.
void BOTH_ORDERS_EXECUTED() { if(OrdersTotal()==0) { GlobalVariableSet(AccountNumber(),AccountBalance()+10); Points=OrderSend(Symbol(),0,0.1,OrderOpenPrice(),3,0,0,"AUTO BUY",0,0,clrNONE); OrderPrint(); GlobalVariableSet("BUY VOLUME",OrderLots()); Points=OrderSend(Symbol(),1,0.1,OrderOpenPrice(),3,0,0,"AUTO SELL",0,0,clrNONE); OrderPrint(); GlobalVariableSet("SELL VOLUME",OrderLots()); } if(OrdersTotal()>0) {MessageBox("WILL NOT EXECUTE THERE IS "+OrdersTotal()+" RECORD");} }
I think Points needs to be (int Points;) not bool.
GlobalVariableSet(AccountNumber(),AccountBalance()+10);
I haven't used GlobalVariableSet before. I usually do this.
I not sure what +10 is meant to do, but if works so be it.
double Acc = AccountBalance(); int AccNum = (AccountNumber());
GrumpyDuckMan:
Hello,
Something doesn't look right here.
I think Points needs to be (int Points;) not bool.
I haven't used GlobalVariableSet before. I usually do this.
I not sure what +10 is meant to do, but if works so be it.
JAMPART:
+10 here I mean when equity goes more than the initial investment by 10 then close transactions if a person more than a account for that purpose. I didn't do the second part. You can gusset what it could be.
Points=OrderSelect(0,SELECT_BY_POS); : if(OrdersTotal()==0){ : Points=OrderSend(Symbol(),0,0.1,OrderOpenPrice(),3,0,0,"AUTO BUY",0,0,clrNONE); OrderPrint(); GlobalVariableSet("BUY VOLUME",OrderLots()); |
|

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register