Your code has the OrderClose inside here
if(total < 1) { }
Your code has the OrderClose inside here
Now the code is giving me the error code 4051, which parameters in the orderclose function could be whats causing this error and the trades to not close?
It also says invalid ticket, do I need to code in a OrderSelect for the orderclose script, when I am only trying to get it to work on 1 chart?
Now the code is giving me the error code 4051, which parameters in the orderclose function could be whats causing this error and the trades to not close?
It also says invalid ticket, do I need to code in a OrderSelect for the orderclose script, when I am only trying to get it to work on 1 chart?
Yes, everything like OrderTicket() OrderClosePrice() etc needs an OrderSelect() first.
You can find error codes here: https://book.mql4.com/appendix/errors
The price you should use to close an order is OrderClosePrice() (not Bid or Ask)
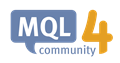
Yes, everything like OrderTicket() OrderClosePrice() etc needs an OrderSelect() first.
You can find error codes here: https://book.mql4.com/appendix/errors
The price you should use to close an order is OrderClosePrice() (not Bid or Ask)
if(OrderSelect(1,SELECT_BY_TICKET,MODE_TRADES)) { if(OrderType()==OP_BUY) { if(fastma < slowma) { if(OrderClose(OrderTicket(),lots,OrderClosePrice(),10,clrNONE)) Print("Position Closed : ", OrderClosePrice()); else Print("Failed to close : ", GetLastError()); } } if(OrderType()==OP_SELL) { if(fastma > slowma) { if(OrderClose(OrderTicket(),lots,OrderClosePrice(),10,clrNONE)) Print("Position Closed : ", OrderClosePrice()); else Print("Failed to close : ", GetLastError()); } } } } }
Here is my orderclose function now, I got it to open and close trade for 1 trade in tester but then it gave me the error 4108, how do I keep it going from here?
ERR_INVALID_TICKET | 4108 | Invalid ticket. |
Unless you have a ticket numbered 1 (highly unlikely) it is going to fail.
Presuming you only ever have 1 order open at a time (not a good presumption, but it will get you started)
if(OrderSelect(0,SELECT_BY_POS,MODE_TRADES))
But you do have more fundamental problems with your code. It is going to be constantly opening/closing orders, except on the very (very) rare occasion that fastma == slowma
ERR_INVALID_TICKET | 4108 | Invalid ticket. |
Unless you have a ticket numbered 1 (highly unlikely) it is going to fail.
Presuming you only ever have 1 order open at a time (not a good presumption, but it will get you started)
But you do have more fundamental problems with your code. It is going to be constantly opening/closing orders, except on the very (very) rare occasion that fastma == slowma
fastma = iMA(Symbol(),0,5,0,MODE_EMA,PRICE_CLOSE,1); slowma = iMA(Symbol(),0,8,0,MODE_SMA,PRICE_CLOSE,1);
Will it still do that if the Moving average are calculated through the next candle?
As well as it is now opening and closing trades perfectly on the tester
input double lots = .10; input int MN = 123; void start() { //Find Moving Averages double fastma, slowma; double total; bool ticket; fastma = iMA(Symbol(),0,5,0,MODE_EMA,PRICE_CLOSE,1); slowma = iMA(Symbol(),0,8,0,MODE_SMA,PRICE_CLOSE,1); total = OrdersTotal(); //Start operations if(total < 1) { if(fastma > slowma) { ticket = OrderSend(Symbol(),OP_BUY,lots,Ask,10,0,0,NULL,MN,0,Blue); if(ticket > 0) { Print("Order opened : ", OrderOpenPrice()); } else Print("Error : ", GetLastError()); } if(fastma < slowma) { ticket = OrderSend(Symbol(),OP_SELL,lots,Bid,10,0,0,NULL,MN,0,Red); if(ticket > 0) { Print("Order opened : ", OrderOpenPrice()); } else Print("Error : ", GetLastError()); } } { { for(int i = OrdersTotal();i<=-1;i--) { if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES)==false) break; if(OrderMagicNumber()==MN && OrderSymbol()==Symbol()) continue; { if(OrderType()==OP_BUY) { if(fastma < slowma) { if(OrderClose(OrderTicket(),lots,OrderClosePrice(),10,clrNONE)) Print("Position Closed : ", OrderClosePrice()); else Print("Failed to close : ", GetLastError()); } } if(OrderType()==OP_SELL) { if(fastma > slowma) { if(OrderClose(OrderTicket(),lots,OrderClosePrice(),10,clrNONE)) Print("Position Closed : ", OrderClosePrice()); else Print("Failed to close : ", GetLastError()); } } } } } } }I am now trying to work in a way to use this EA on multiple charts, as is it is running perfectly on one chart, on the slightly older code meant for one chart, but i'd like to be able to run it on more than one. Is there anything that I can change in my code to enable it to properly sort between different trades?
if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES)==false) break; if(OrderMagicNumber()==MN && OrderSymbol()==Symbol()) continue;You select an order. This if it is for the current pair and the EAs magic number, you reject it.
You select an order. This if it is for the current pair and the EAs magic number, you reject it.
I have removed the ==false in my orderselect function now I am getting the error 4051, do you know which parameter is incorrect for this function?
Edit: Nevermind it is in the orderclose function
I have removed the ==false in my orderselect function now I am getting the error 4051, do you know which parameter is incorrect for this function?
Edit: Nevermind it is in the orderclose function
The ==false was not the problem. No if you select any order, you reject it. The break should have been a continue.
The next line is the problem. You only process orders that are not using MN or not on the current chart pair.
The OrderClose function is irrelevant.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Awhile back I had an EA that worked just fine on a single chart, but when I loaded it up in the tester, it started to open trades on every candle. So I started this one, and it opens trades just fine, but will not close trades. Does anyone know how to get the EA to close trades based off the parameters in the code?