
You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Hello all,
I am working on modifying a currency strength meter to show the strength of the currency based on the time from of the chart. At present, the code used is:
aLow = MarketInfo(mySymbol,MODE_LOW); // set a low today
aBid = MarketInfo(mySymbol,MODE_BID); // set a last bid
aAsk = MarketInfo(mySymbol,MODE_ASK);I understand MODE_HIGH and MODE_LOW get the current days highs and low. How could I get the high and low of the charts current time frame instead?
Thanks!
P.S. I am not a programmer, so use small words please:)You do not have to use MarketInfo(). If you want to get the current High, use High[0] and Low[n], Close[n], Open[n]. 'n' signifies the offset from the current bar. For example to get the High of the bar before the current, you can use High[1] since the current is 0 and the bar before it has an offset of 1 from the current.
To check for Highs, Lows, Opens, Closes of other timeframes other than you are viewing on the chart, you may use iHigh(), iLow(), iClose(), and iOpen functions.
Sample usage below.
double high_of_gbpusd_4hours_current = iHigh("gbpusd", period_h4, 0);
Thanks! You guys rock!
I think I pretty much have it working (not sure??), but for some reason the array gets filled with a lot of '0' values along with the legitimate values.
This is how I'd do it:
#define TICKET 0
#define PRICES 1
double ResumeArray[0,2];
int CheckForResume()
{
ResumeCheck = TimeLocal()+(PERIOD_M1*60)*5;
bool success = false;
int k;
for (int i = 0; i<OrdersTotal();i++)
{
if(!OrderSelect(i,SELECT_BY_POS,MODE_TRADES))continue;
if (OrderType() > OP_SELL || OrderProfit()> Cutoff)continue;
int arraycount=ArrayRange(ResumeArray,TICKET);
bool found = false;
for (k=arraycount-1; k>=0; k--)
{
if (ResumeArray[k,TICKET] == OrderTicket())
{
found = true;
if (ResumeArray[k,PRICES] + Cutoff < OrderProfit())break;
else
{
success=true;
Print ("ELSE");
ResumeArray[k,PRICES] = OrderProfit();
break;
}
}
}
if (found == false)
{
arraycount = ArrayResize(ResumeArray,arraycount+1)/2;
ResumeArray[arraycount-1,TICKET] = OrderTicket();
ResumeArray[arraycount-1,PRICES] = OrderProfit();
success=true;
}
}
if(success)return (1);
else return(0);
}
You don't have to use the define TICKET and PRICES, I've just included them to show how a 2 dimension array works. Simply replace all occurences of TICKET and PRICES with 0 and 1 respectively.
I've replaced the 'return' commands in the 'for' loop with 'break', so all open orders will be processed.
One thing to note is that closed orders are never removed from the array, so it will continue to grow in size, and slow down your platform.
d4v3
Bars history limit
I would be very grateful if somone can add bars limit option to this RenkoLiveChart_v3.2 script.
It takes takes too much computer resources.
Basic Alerting EA
................[solved] ....................
noise reduction
hi all - I have been looking at a way to limit the noise on a trade and making a faster cutoff
The idea is actually quite simple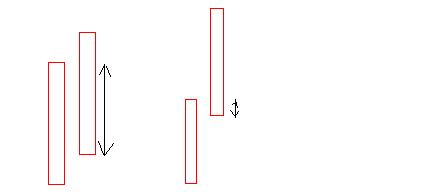
the noise is shown in this pic its dynamic meaning it keeps changing to suit the market condition
here is some code I have made for the close if anyone wants to use it feel free it just needs some multipliers putting into it.
int Trades = ScanTrades(0,0);
if (Trades == 0) TS1 = 0;
if ((ScanTrades(2,0) > 0) && (profit < 0)) TS= ((MarketInfo(Symbol(),MODE_BID)) + noise + Slippage);
if ((ScanTrades(2,0) > 0) && (profit > 0)) TS= ((MarketInfo(Symbol(),MODE_BID)) + noise );
if ((ScanTrades(1,0) > 0) && (profit < 0)) TS= ((MarketInfo(Symbol(),MODE_BID)) - noise - Slippage);
if ((ScanTrades(1,0) > 0) && (profit > 0)) TS= ((MarketInfo(Symbol(),MODE_BID)) - noise );
//short
if ((ScanTrades(2,0) > 0)&& (TS1 ==0 )) TS1 = TS;
if ((ScanTrades(2,0) > 0)&& (TS < TS1)) TS1 = TS;
if ((ScanTrades(2,0) > 0) && (MarketInfo(Symbol(),MODE_BID) > TS1)) CloseOrder(2);
//long
if ((ScanTrades(1,0) > 0)&& (TS1 ==0 )) TS1 = TS;
if ((ScanTrades(1,0) > 0)&& (TS > TS1)) TS1 = TS;
if ((ScanTrades(1,0) > 0) && (MarketInfo(Symbol(),MODE_BID) < TS1)) CloseOrder(1);
what would be the best way of getting the current and previous high and low off a bar chart. I need to create the variable "noise"
I tried ths but its hit and miss t1 and l1 are sometime 0 its most depressing as its all that stands between the whole ea being "finished" if there ever is such a thing.
double ll2=Low;
double hh3=High;
double ll3=Low;
double t1,l1,noise;
//noise = overlap of previous bars
if ((hh2 - hh3) > 0 ) t1 =(hh3); //basically picking the lowest "high" bar between the two -bullish
if ((hh3- hh2) > 0 ) t1=(hh2); //bearish
if ((ll2 - ll3) > 0 ) l1 =(ll2); //picking the highest low bar bullish
if ((ll3-ll2) > 0 ) l1=(ll3); //bearish
noise =(t1-l1);
Print("t1 = ",t1,"; l1 = ",l1);the typical results of print are t1=0 l1=94.3 its most annoying its because hh2 and hh3 are == i just dont know what to do I just cant see a problem with my code.
who canhelp me ?
can someone show me how to make a new indicator which is based on 3 familiar indicators? I make a strategy based on 3 indicators which show very strong buy/sell signals,but I want to create 1 indicator...
ea ammendment please
Please help me implement the following changes to this ea
1)Add in hour
A) fisher_ 11 indicator with settings 33,0.5,0.5
B) tcf smoothed indicator settings 17, 8, 0.7
c) Also I want the dinap _basic indicator not to allow trades when it has drawn against signal direction i.e if it draws up ,entering buy signals are NOT allowed, if it draws down ,entering sell signals are NOT allowed.
2) Add in 15 minutes
A) supertrend indicator
B) Trendlord indicator
Money management of
1) Open 2 trades at 3 % stop loss of account total ( 1.5% )each
2) One at 80 pips take profit or 2.2 % and the second at 3.8% take profit
3) Double lot size for fifth trade after 4 consecutive losses then linear lots increment for subsequent trades till two consecutive wins and back to default lot size.
4) Trailing stop of 12pips (above for sell and below for buy of former candle atrstops_v1 indicator for all trades.
That’s all please help
You could have achieved exactly the same thing with one line of code.
GlobalVariableSet("TRENDSET_"+Symbol());
You could also add the time frame to make it even more unique.
Not quite sure what the point of your post was to be honest.
Regards
Lux
How to call value from H1 using iCustom ?
Hello,
I'm trying to code a very simple indicator, but i'm unable to call Laguerre value from H1.
what i want to do :
Using 1M TF
buy signal : Laguerre crossing 0.15 level head up and Laguerre H1 > 0.75
sell signal : Laguerre crossing 0.75 level head down and Laguerre H1 < 0.15
i made the indicator for the simple cross and it works, the problem is when i try to check the value from Laguerre H1: it's a mess!
I tried 2 ways:
First one:
#property indicator_buffers 2
#property indicator_color1 SeaGreen
#property indicator_color2 Red
#property indicator_width1 3
#property indicator_width2 3
double CrossUp[];
double CrossDown[];
extern bool SoundON=true;
double alertTag;
//+------------------------------------------------------------------+
//| Custom indicator initialization function |
//+------------------------------------------------------------------+
int init()
{
//---- indicators
SetIndexStyle(0, DRAW_ARROW, EMPTY,3);
SetIndexArrow(0, 233);
SetIndexBuffer(0, CrossUp);
SetIndexStyle(1, DRAW_ARROW, EMPTY,3);
SetIndexArrow(1, 234);
SetIndexBuffer(1, CrossDown);
//----
return(0);
}
//+------------------------------------------------------------------+
//| Custom indicator deinitialization function |
//+------------------------------------------------------------------+
int deinit()
{
//----
//----
return(0);
}
//+------------------------------------------------------------------+
//| Custom indicator iteration function |
//+------------------------------------------------------------------+
int start() {
int limit, i;
int counted_bars=IndicatorCounted();
//---- check for possible errors
if(counted_bars<0) return(-1);
//---- last counted bar will be recounted
if(counted_bars>0) counted_bars--;
limit=Bars-counted_bars;
for(i = 0; i <= limit; i++) {
if ( (iCustom(NULL,0,"Laguerre",0.66,9500,0,i) > 0.15) && (iCustom(NULL,0,"Laguerre",0.66,9500,0,i+1) < 0.15)
&&(iCustom(Symbol(),PERIOD_H1,"Laguerre",0.66,9500,0,i) > 0.75)) //check for buy signal
{
CrossUp = Close;
}
else if ( (iCustom(NULL,0,"Laguerre",0.66,9500,0,i) 0.75)
&& (iCustom(Symbol(),PERIOD_H1,"Laguerre",0.66,9500,0,i) < 0.15)) //check for sell signal
{
CrossDown = Close;
}
if (SoundON==true && i==1 && CrossUp > CrossDown && alertTag!=Time[0]){
Alert("SHORT signal on ",Symbol()," ",Period());
alertTag = Time[0];
}
if (SoundON==true && i==1 && CrossUp < CrossDown && alertTag!=Time[0]){
Alert("Long Signal on ",Symbol()," ",Period());
alertTag = Time[0];
}
}
return(0);
}[/CODE]
second one:
[CODE]//+------------------------------------------------------------------+
//| Shift.mq4 |
//| me |
//|
//+------------------------------------------------------------------+
#property copyright "me"
#property indicator_chart_window
#property indicator_buffers 2
#property indicator_color1 Lime
#property indicator_color2 Red
#property indicator_width1 4
#property indicator_width2 4
//---- buffers
double ExtMapBuffer1[];
double ExtMapBuffer2[];
bool Fact_Up = true; // Fact of report that market..
bool Fact_Dn = true; //..is bullish or bearish
//+------------------------------------------------------------------+
//| Custom indicator initialization function |
//+------------------------------------------------------------------+
int init()
{
//---- indicators
SetIndexStyle(0,DRAW_ARROW);
SetIndexArrow(0,241);
SetIndexBuffer(0,ExtMapBuffer1);
SetIndexEmptyValue(0,0.0);
SetIndexStyle(1,DRAW_ARROW);
SetIndexArrow(1,242);
SetIndexBuffer(1,ExtMapBuffer2);
SetIndexEmptyValue(1,0.0);
//----
return(0);
}
//+------------------------------------------------------------------+
//| Custom indicator deinitialization function |
//+------------------------------------------------------------------+
int deinit()
{
//----
//----
return(0);
}
//+------------------------------------------------------------------+
//| Custom indicator iteration function |
//+------------------------------------------------------------------+
int start()
{
//int limit;
int counted_bars=IndicatorCounted();
//---- last counted bar will be recounted
if(counted_bars>0) counted_bars--;
if(counted_bars<0) return(-1);
//limit=Bars-counted_bars;
//----
double MA; // MA value on 0 bar
double MA2;
double MA3;
double MA4;
//--------------------------------------------------------------------
double value1, value2, value3;
for (int i=Bars-1;i>=0;i--)
//---- main loop
//for(int i=0; i<limit; i++)
{value1=0.0;
value2=0.0;
value3=0.0;
//--------------------------------------------------------------------
// Checking if bullish
if( (iCustom(Symbol(),NULL,"Laguerre",0,i) > 0.15) && (iCustom(Symbol(),NULL,"Laguerre",0,i+1) < 0.15) && Fact_Up == true
&&(iCustom(Symbol(),PERIOD_H1,"Laguerre",0,i) > 0.75))
{
Fact_Dn = true; // Report about bullish market
Fact_Up = false; // Don't report bullish market
// Alert("BUY SIGNAL.");
value1=Open;
}
//--------------------------------------------------------------------
// Checking if bearish
if( (iCustom(Symbol(),NULL,"Laguerre",0,i) 0.75) && Fact_Dn == true
&&(iCustom(Symbol(),PERIOD_H1,"Laguerre",0,i) < 0.15))
{
Fact_Up = true; // Report about bearish market
Fact_Dn = false; // Don't report bearish market
// Alert("SELL SIGNAL.");
value2=Open;
}
ExtMapBuffer1=value1;
ExtMapBuffer2=value2;
}
//----
return(0);
}
//+------------------------------------------------------------------+both don't work, the RED line is the problem, without the RED line it works perfectly.
i tried (iCustom(Symbol(),PERIOD_H1,"Laguerre",0,i) < .....)
(iCustom(Symbol(),PERIOD_H1,"Laguerre",0,0) < ....)
(iCustom(Symbol(),60,"Laguerre",0,i) < ....)
(iCustom(Symbol(),60,"Laguerre",0,0) < .....)
I tried also with variables: (iCustom(Symbol(),PERIOD_H1,"Laguer re",0.66,9500,0,i)
iF i put:
Print(iCustom(Symbol(),60,"Laguerre ",0,i)); before the "if" it returns random number...
but with: Print(iCustom(Symbol(),60,"Laguerre ",0,0)); it returns the good value from H1
now the problem remain the same if i use iCustom(Symbol(),60,"Laguerre",0,0); inside the "if" it doesn't work...
the problem is with the "for" and "i" and the iCustom "shift" value but i can't figure it out.
your help would be very welcome !
i attached the Laguerre indicator i use if you want give a try.
if someone is ready to recode it from scratch please don't hesitate
it's driving me nutz...