When you change period or chart, the EA unloads itself and Deinit function is triggered (with reason = REASON_CHARTCHANGE or 3). The EA is then reloaded and Init function is triggered. This happens in sequence. You can see this behavior in the Expert window of the Terminal panel (Ctrl+T).
You would have to assign radio button to global variables (on radio button change, or on deinit function) and then reload those variables to the radio buttons on init function.
![]() Play video | Please edit your
post. For large amounts of code, attach it |
hmm isn't that what I did? I believe I'm using timeframe, timeframe2 and TradeStatus globally.
Their default value is 0. After clicking on a radiobutton the value should change to a menuPeriod
void CheckRadioButton()
{...
if (guiIsChecked(hwnd, RChart6)) {timeframe = MenuPeriod6; }
...}
, which is global as well. All three of those variables are printed on the chart
if(TradeStatus==0){
guiPrintXY(MenuPair1,OrangeRed,gUIXPosition,gUIYPosition-60,14,"Arial Bold");
}
...
guiPrintXY(MenuPair4,Tomato,gUIXPosition,gUIYPosition-60,14,"Arial Bold");
}
guiPrintXY(timeframe,LightGreen,gUIXPosition,gUIYPosition-35,14,"Arial Bold");
guiPrintXY(timeframe2,LightBlue,gUIXPosition+60,gUIYPosition-35,14,"Arial Bold");
}
, to see the current status and the corresponding radio button will be checked (if timeframe==menuPeriodX) if you open the chartmenu.
{...
if(timeframe == MenuPeriod1) guiSetChecked(hwnd, RChart1, true);
...}
Up to this point everything works as intended. Unfortunately they still get reset after changing the period.
hmm isn't that what I did? I believe I'm using timeframe, timeframe2 and TradeStatus globally.
Their default value is 0. After clicking on a radiobutton the value should change to a menuPeriod
void CheckRadioButton()
{...
if (guiIsChecked(hwnd, RChart6)) {timeframe = MenuPeriod6; }
...}
, which is global as well. All three of those variables are printed on the chart
if(TradeStatus==0){
guiPrintXY(MenuPair1,OrangeRed,gUIXPosition,gUIYPosition-60,14,"Arial Bold");
}
...
guiPrintXY(MenuPair4,Tomato,gUIXPosition,gUIYPosition-60,14,"Arial Bold");
}
guiPrintXY(timeframe,LightGreen,gUIXPosition,gUIYPosition-35,14,"Arial Bold");
guiPrintXY(timeframe2,LightBlue,gUIXPosition+60,gUIYPosition-35,14,"Arial Bold");
}
, to see the current status and the corresponding radio button will be checked (if timeframe==menuPeriodX) if you open the chartmenu.
{...
if(timeframe == MenuPeriod1) guiSetChecked(hwnd, RChart1, true);
...}
Up to this point everything works as intended. Unfortunately they still get reset after changing the period.
No. Global variables are defined by GlobalVariableSet(name,value), and retrieved by GlobalVariableGet(name). timeframe, timeframe2 and TradeStatus reset to null when EA unloads.
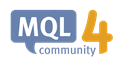
- docs.mql4.com
No. Global variables are defined by GlobalVariableSet(name,value), and retrieved by GlobalVariableGet(name). timeframe, timeframe2 and TradeStatus reset to null when EA unloads.
Ohhh, thanks. That explains a lot.
I tried to change my code with that in mind.
I added these on a global scale:
this on init:
BuildInterface();
timeframe = GlobalVariableGet(Gltimeframe);
timeframe2 = GlobalVariableGet(Gltimeframe2);
TradeStatus = GlobalVariableGet(GlTradeStatus);
StatusPrint();
this to CheckRadioButton():
{
if (guiIsChecked(hwnd, RChart1)) {timeframe = MenuPeriod1; GlobalVariableSet(Gltimeframe, timeframe);}
if (guiIsChecked(hwnd, RChart9)) {timeframe2 = MenuPeriod1; GlobalVariableSet(Gltimeframe2, timeframe2);}
if (guiIsChecked(hwnd, RTerm1)) {TradeStatus=0; GlobalVariableSet(GlTradeStatus, TradeStatus);}
and this to StatusPrint() as a control:
guiPrintXY(GlobalVariableGet(Gltimeframe2), LightBlue, gUIXPosition-50,gUIYPosition-35,14,"Arial Bold");
guiPrintXY(GlobalVariableGet(GlTradeStatus), LightGreen, gUIXPosition-80,gUIYPosition-60,14,"Arial Bold");
Unfortunately I still can't seem to get it right. If I change the period with the radiobuttons now, it will set all three global variables to the same value.
Your post | case 43200: return("MN1"); break; } } void StatusPrint(){ if(TradeStatus==0){ guiPrintXY(MenuPair1,OrangeRed,gUIXPosition,gUIYPosition-60,14,"Arial Bold"); |
Your post using SRC | case 43200: See the differencereturn("MN1"); break; } } void StatusPrint(){ if(TradeStatus==0){ guiPrintXY(MenuPair1,OrangeRed,gUIXPosition,gUIYPosition-60,14,"Arial Bold"); |
Your post | case 43200: return("MN1"); break; } } void StatusPrint(){ if(TradeStatus==0){ guiPrintXY(MenuPair1,OrangeRed,gUIXPosition,gUIYPosition-60,14,"Arial Bold"); |
Your post using SRC | case 43200: See the differencereturn("MN1"); break; } } void StatusPrint(){ if(TradeStatus==0){ guiPrintXY(MenuPair1,OrangeRed,gUIXPosition,gUIYPosition-60,14,"Arial Bold"); |
I get that, but I'm sorry, I don't see any code, that I didn't write inside of a src div. The only thing is, that doesn't seem to work properly is my first post. I should have probably attached that instead.
@Quinius - If it's possible when you solve your ' Global Variables ' issue, then post that part of code with us that who struggle to solve it. Thanks for your attention. Good luck!
Sure I will do that. Unfortunately I have no idea what the problem is at this point. I don't know why it won't save my variables seperately...
Ohhh, thanks. That explains a lot.
I tried to change my code with that in mind.
I added these on a global scale:
this on init:
BuildInterface();
timeframe = GlobalVariableGet(Gltimeframe);
timeframe2 = GlobalVariableGet(Gltimeframe2);
TradeStatus = GlobalVariableGet(GlTradeStatus);
StatusPrint();
this to CheckRadioButton():
{
if (guiIsChecked(hwnd, RChart1)) {timeframe = MenuPeriod1; GlobalVariableSet(Gltimeframe, timeframe);}
if (guiIsChecked(hwnd, RChart9)) {timeframe2 = MenuPeriod1; GlobalVariableSet(Gltimeframe2, timeframe2);}
if (guiIsChecked(hwnd, RTerm1)) {TradeStatus=0; GlobalVariableSet(GlTradeStatus, TradeStatus);}
and this to StatusPrint() as a control:
guiPrintXY(GlobalVariableGet(Gltimeframe2), LightBlue, gUIXPosition-50,gUIYPosition-35,14,"Arial Bold");
guiPrintXY(GlobalVariableGet(GlTradeStatus), LightGreen, gUIXPosition-80,gUIYPosition-60,14,"Arial Bold");
Unfortunately I still can't seem to get it right. If I change the period with the radiobuttons now, it will set all three global variables to the same value.
G1timeframe,G1timeframe2, and G1TradeStatus should be defined as string as they are names to which the values are assigned. Lets see if it works.
I changed them to string. But that didn't help. In fact it seems to be worse. Double should be correct.
I attached my current version.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hey,
I'm trying to build my first EA and I've run into a problem that I can't solve.
I used mt4gui to build an interface for my ea and it's working fine. It changes the timeframe and tradestatus when I click on the corresponding radiobutton.
Unfortunately the variables (timeframe, timeframe2 and TradeStatus) always reset to default when I'm changing the timeframe in MT4, instead of saving them.
I would really appreciate any explanation or possibly solution to that problem.
Thank you in advance.
Quinius
#define SIGNAL_NONE 0
#define SIGNAL_BUY 1
#define SIGNAL_SELL 2
#define SIGNAL_CLOSEBUY 3
#define SIGNAL_CLOSESELL 4
#include <mt4gui2.mqh>
extern int MagicNumber = 12345;
extern bool SignalMail = False;
extern double Lots = 1.0;
extern int Slippage = 3;
extern bool UseStopLoss = True;
extern int StopLoss = 20;
extern bool UseTakeProfit = False;
extern int TakeProfit = 30;
extern bool UseTrailingStop = False;
extern int TrailingStop = 30;
extern int speed = 400;
extern double timeframe;
extern double timeframe2;
extern double sma_short = 55;
extern double sma_long = 55;
extern bool isSizingOn = true;
extern double Risk = 1;
int P = 1;
int Order = SIGNAL_NONE;
int Total, Ticket, Ticket2;
double StopLossLevel, TakeProfitLevel, StopLevel;
double sma10_1, sma10_2, sma40_1, sma40_2;
double atr_current, atr_past;
bool isYenPair = false;
bool first_time = True;
int BarsCount = 0;
// GUI Object Handles
// Settings
int hwnd = 0;
int menuTerminalBtn, menuChartBtn;
int controlPanelHeader, controlPanelMenuTerminal, controlPanelMenuChart;
bool chartMenuOpen=false, terminalMenuOpen=false;
int RChart1,RChart2,RChart3,RChart4,RChart5,RChart6,RChart7,RChart8,RChart9,RChart10,RChart11,RChart12,RChart13,RChart14,RChart15,RChart16;
int RTerm1, RTerm2, RTerm3, RTerm4;
int gUIXPosition, gUIYPosition;
extern int TradeStatus;
// Declare variables that are adjustable in the EA properties inputs
extern string MENUPAIRS = "--- Choose menu pairs ---",
MenuPair1 = "Do Not Trade",
MenuPair2 = "Trade Both",
MenuPair3 = "Long Only",
MenuPair4 = "Short Only";
extern string MENUPERIODS = "--- Choose menu periods ---";
extern int MenuPeriod1 = 1, //1Min
MenuPeriod2 = 5, //5Min
MenuPeriod3 = 15, //15Min
MenuPeriod4 = 30, //30Min
MenuPeriod5 = 60, //1Hour
MenuPeriod6 = 240, //4Hours
MenuPeriod7 = 1440, //1Day
MenuPeriod8 = 10080; //1Week
extern string GUICOLORS = "--- Color settings ---";
extern color ChartBtnColor = PaleGreen,
ChartMenuColor = LightGreen,
ChartMenuColor2 = LightBlue,
TerminalBtnColor = CornflowerBlue,
TerminalMenuColor = RoyalBlue;
extern string GUIOFFSET = "--- Offset from borders ---";
extern int GUIXOffset = -180,
GUIYOffset = 80;
//+------------------------------------------------------------------+
//| Expert initialization function |
//+------------------------------------------------------------------+
int init() {
if(Digits == 5 || Digits == 3 || Digits == 1)P = 10;else P = 1; // To account for 5 digit brokers
if(Digits == 3 || Digits == 2) isYenPair = true; // Adjust for YenPair
hwnd = WindowHandle(Symbol(),Period());
ObjectsDeleteAll();
guiRemoveAll(hwnd);
AutoPositionControlPanel();
BuildInterface();
StatusPrint();
return(0);
}
//+------------------------------------------------------------------+
//| Expert initialization function - END |
//+------------------------------------------------------------------+
//+------------------------------------------------------------------+
//| Expert deinitialization function |
//+------------------------------------------------------------------+
int deinit() {
if (hwnd>0) { guiRemoveAll(hwnd); guiCleanup(hwnd ); }
return(0);
}
//+------------------------------------------------------------------+
//| Expert deinitialization function - END |
//+------------------------------------------------------------------+
//+------------------------------------------------------------------+
//| Expert start function |
//+------------------------------------------------------------------+
int start() {
if(gUIXPosition != (guiGetChartWidth(hwnd)+GUIXOffset)){
guiRemoveAll(hwnd);
guiCleanup(hwnd);
chartMenuOpen=false;
terminalMenuOpen=false;
ObjectDelete(StringConcatenate("pxy3txt_", gUIXPosition, "_", gUIYPosition-60));
ObjectDelete(StringConcatenate("pxy3txt_", gUIXPosition, "_", gUIYPosition-35));
ObjectDelete(StringConcatenate("pxy3txt_", gUIXPosition+60, "_", gUIYPosition-35));
AutoPositionControlPanel();
BuildInterface();
}
ManageEvents();
StatusPrint();
if (Bars > BarsCount)
{
Total = OrdersTotal();
Order = SIGNAL_NONE;
StopLevel = MarketInfo(Symbol(), MODE_STOPLEVEL) + MarketInfo(Symbol(), MODE_SPREAD); // Defining minimum StopLevel
if (StopLoss < StopLevel) StopLoss = StopLevel;
if (TakeProfit < StopLevel) TakeProfit = StopLevel;
if (isSizingOn == true) {
Lots = Risk * 0.01 * AccountBalance() / (MarketInfo(Symbol(),MODE_LOTSIZE) * StopLoss * P * Point); // Sizing Algo based on account size
if(isYenPair == true) Lots = Lots * 100; // Adjust for Yen Pairs
Lots = NormalizeDouble(Lots, 2); // Round to 2 decimal place
}
//+------------------------------------------------------------------+
//| Variable Setup - END |
//+------------------------------------------------------------------+
//Check position
bool IsTrade = False;
for (int i = 0; i < Total; i ++) {
Ticket2 = OrderSelect(i, SELECT_BY_POS, MODE_TRADES);
if(OrderType() <= OP_SELL && OrderSymbol() == Symbol() && OrderMagicNumber() == MagicNumber) {
IsTrade = True;
if(OrderType() == OP_BUY) {
//Close
//+------------------------------------------------------------------+
//| Signal Begin(Exit Buy) |
//+------------------------------------------------------------------+
//+------------------------------------------------------------------+
//| Signal End(Exit Buy) |
//+------------------------------------------------------------------+
if (Order == SIGNAL_CLOSEBUY) {
Ticket2 = OrderClose(OrderTicket(), OrderLots(), Bid, Slippage, MediumSeaGreen);
if (SignalMail) SendMail("[Signal Alert]", "[" + Symbol() + "] " + DoubleToStr(Bid, Digits) + " Close Buy");
IsTrade = False;
continue;
}
//Trailing stop
if(UseTrailingStop && TrailingStop > 0) {
if(Bid - OrderOpenPrice() > P * Point * TrailingStop) {
if(OrderStopLoss() < Bid - P * Point * TrailingStop) {
Ticket2 = OrderModify(OrderTicket(), OrderOpenPrice(), Bid - P * Point * TrailingStop, OrderTakeProfit(), 0, MediumSeaGreen);
continue;
}
}
}
} else {
//Close
//+------------------------------------------------------------------+
//| Signal Begin(Exit Sell) |
//+------------------------------------------------------------------+
//+------------------------------------------------------------------+
//| Signal End(Exit Sell) |
//+------------------------------------------------------------------+
if (Order == SIGNAL_CLOSESELL) {
Ticket2 = OrderClose(OrderTicket(), OrderLots(), Ask, Slippage, DarkOrange);
if (SignalMail) SendMail("[Signal Alert]", "[" + Symbol() + "] " + DoubleToStr(Ask, Digits) + " Close Sell");
continue;
}
//Trailing stop
if(UseTrailingStop && TrailingStop > 0) {
if((OrderOpenPrice() - Ask) > (P * Point * TrailingStop)) {
if((OrderStopLoss() > (Ask + P * Point * TrailingStop)) || (OrderStopLoss() == 0)) {
Ticket2 = OrderModify(OrderTicket(), OrderOpenPrice(), Ask + P * Point * TrailingStop, OrderTakeProfit(), 0, DarkOrange);
continue;
}
}
}
}
}
}
//+------------------------------------------------------------------+
//| Signal Begin(Entries) |
//+------------------------------------------------------------------+
//+------------------------------------------------------------------+
//| Signal End |
//+------------------------------------------------------------------+
//Buy
if (Order == SIGNAL_BUY) {
if(!IsTrade) {
//Check free margin
if (AccountFreeMargin() < (1000 * Lots)) {
Print("We have no money. Free Margin = ", AccountFreeMargin());
return(0);
}
if (UseStopLoss) StopLossLevel = Ask - StopLoss * Point * P; else StopLossLevel = 0.0;
if (UseTakeProfit) TakeProfitLevel = Ask + TakeProfit * Point * P; else TakeProfitLevel = 0.0;
Ticket = OrderSend(Symbol(), OP_BUY, Lots, Ask, Slippage, StopLossLevel, TakeProfitLevel, "Buy(#" + MagicNumber + ")", MagicNumber, 0, DodgerBlue);
if(Ticket > 0) {
if (OrderSelect(Ticket, SELECT_BY_TICKET, MODE_TRADES)) {
Print("BUY order opened : ", OrderOpenPrice());
if (SignalMail) SendMail("[Signal Alert]", "[" + Symbol() + "] " + DoubleToStr(Ask, Digits) + " Open Buy");
} else {
Print("Error opening BUY order : ", GetLastError());
}
}
return(0);
}
}
//Sell
if (Order == SIGNAL_SELL) {
if(!IsTrade) {
//Check free margin
if (AccountFreeMargin() < (1000 * Lots)) {
noMoneyPrint();
return(0);
}
if (UseStopLoss) StopLossLevel = Bid + StopLoss * Point * P; else StopLossLevel = 0.0;
if (UseTakeProfit) TakeProfitLevel = Bid - TakeProfit * Point * P; else TakeProfitLevel = 0.0;
Ticket = OrderSend(Symbol(), OP_SELL, Lots, Bid, Slippage, StopLossLevel, TakeProfitLevel, "Sell(#" + MagicNumber + ")", MagicNumber, 0, DeepPink);
if(Ticket > 0) {
if (OrderSelect(Ticket, SELECT_BY_TICKET, MODE_TRADES)) {
Print("SELL order opened : ", OrderOpenPrice());
if (SignalMail) SendMail("[Signal Alert]", "[" + Symbol() + "] " + DoubleToStr(Bid, Digits) + " Open Sell");
} else {
Print("Error opening SELL order : ", GetLastError());
}
}
return(0);
}
}
BarsCount = Bars;
}
return(0);
}
//+------------------------------------------------------------------+
//+------------------------------------------------------------------+
// FUNCTION LIBRARY |
//+------------------------------------------------------------------+
//+------------------------------------------------------------------+
// Customized Print |
//+------------------------------------------------------------------+
void noMoneyPrint(){
Print("We have no money. Free Margin = ", AccountFreeMargin());
}
void ManageEvents()
{
if (guiIsClicked(hwnd,menuTerminalBtn)) TerminalMenu();
if (guiIsClicked(hwnd,menuChartBtn)) ChartMenu();
CheckRadioButton();
}
// MT4GUI functions to build Interface with labels, buttons & textfields
//+-------------------------------------------------------------------------------------------+
void BuildInterface()
{
// Build control panel (labels) and set look and feel for it
controlPanelHeader = guiAdd(hwnd,"label",gUIXPosition,gUIYPosition,120,20," ControlPanel");
guiSetBgColor(hwnd,controlPanelHeader, Gainsboro);
guiSetTextColor(hwnd,controlPanelHeader,Black);
// Create control panel menu buttons, Terminal & Chart and set look and feel for them
menuTerminalBtn = guiAdd(hwnd,"button",gUIXPosition,gUIYPosition+20,60,20,"");
guiSetBorderColor(hwnd,menuTerminalBtn,TerminalMenuColor);
guiSetBgColor(hwnd,menuTerminalBtn, TerminalBtnColor);
guiSetTextColor(hwnd,menuTerminalBtn,White);
guiSetText(hwnd,menuTerminalBtn,"Status",15,"Arial Bold");
menuChartBtn = guiAdd(hwnd,"button",gUIXPosition+60,gUIYPosition+20,60,20,"");
guiSetBorderColor(hwnd,menuChartBtn,ChartMenuColor);
guiSetBgColor(hwnd,menuChartBtn,ChartBtnColor);
guiSetTextColor(hwnd,menuChartBtn,Black);
guiSetText(hwnd,menuChartBtn,"Time",15,"Arial Bold");
}
// MT4GUI functions to change chart settings within GUI
//+-------------------------------------------------------------------------------------------+
void ChartMenu()
{
if (chartMenuOpen)
{
RemoveCPMC();
}
else
{
RemoveCPMT();
controlPanelMenuChart = guiAdd(hwnd,"label",gUIXPosition,gUIYPosition+40,120,80,"");
guiSetBgColor(hwnd,controlPanelMenuChart,ChartMenuColor);
chartMenuOpen=true;
guiGroupRadio(hwnd);
// Radio Buttons for chart functions
RChart1 = guiAdd(hwnd,"radio",gUIXPosition,gUIYPosition+40,120,20,PeriodToText(MenuPeriod1));
RChart2 = guiAdd(hwnd,"radio",gUIXPosition,gUIYPosition+60,120,20,PeriodToText(MenuPeriod2));
RChart3 = guiAdd(hwnd,"radio",gUIXPosition,gUIYPosition+80,120,20,PeriodToText(MenuPeriod3));
RChart4 = guiAdd(hwnd,"radio",gUIXPosition,gUIYPosition+100,120,20,PeriodToText(MenuPeriod4));
RChart5 = guiAdd(hwnd,"radio",gUIXPosition,gUIYPosition+120,120,20,PeriodToText(MenuPeriod5));
RChart6 = guiAdd(hwnd,"radio",gUIXPosition,gUIYPosition+140,120,20,PeriodToText(MenuPeriod6));
RChart7 = guiAdd(hwnd,"radio",gUIXPosition,gUIYPosition+160,120,20,PeriodToText(MenuPeriod7));
RChart8 = guiAdd(hwnd,"radio",gUIXPosition,gUIYPosition+180,120,20,PeriodToText(MenuPeriod8));
guiGroupRadio(hwnd);
RChart9 = guiAdd(hwnd,"radio",gUIXPosition,gUIYPosition+200,120,20,StringConcatenate("Slow ",PeriodToText(MenuPeriod1)));
RChart10 = guiAdd(hwnd,"radio",gUIXPosition,gUIYPosition+220,120,20,StringConcatenate("Slow ",PeriodToText(MenuPeriod2)));
RChart11 = guiAdd(hwnd,"radio",gUIXPosition,gUIYPosition+240,120,20,StringConcatenate("Slow ",PeriodToText(MenuPeriod3)));
RChart12 = guiAdd(hwnd,"radio",gUIXPosition,gUIYPosition+260,120,20,StringConcatenate("Slow ",PeriodToText(MenuPeriod4)));
RChart13 = guiAdd(hwnd,"radio",gUIXPosition,gUIYPosition+280,120,20,StringConcatenate("Slow ",PeriodToText(MenuPeriod5)));
RChart14 = guiAdd(hwnd,"radio",gUIXPosition,gUIYPosition+300,120,20,StringConcatenate("Slow ",PeriodToText(MenuPeriod6)));
RChart15 = guiAdd(hwnd,"radio",gUIXPosition,gUIYPosition+320,120,20,StringConcatenate("Slow ",PeriodToText(MenuPeriod7)));
RChart16 = guiAdd(hwnd,"radio",gUIXPosition,gUIYPosition+340,120,20,StringConcatenate("Slow ",PeriodToText(MenuPeriod8)));
// Look and feel for chart radio buttons
guiSetBgColor(hwnd,RChart1,ChartMenuColor);guiSetBgColor(hwnd,RChart2,ChartMenuColor);guiSetBgColor(hwnd,RChart3,ChartMenuColor);guiSetBgColor(hwnd,RChart4,ChartMenuColor);
guiSetBgColor(hwnd,RChart5,ChartMenuColor);guiSetBgColor(hwnd,RChart6,ChartMenuColor);guiSetBgColor(hwnd,RChart7,ChartMenuColor);guiSetBgColor(hwnd,RChart8,ChartMenuColor);
guiSetBgColor(hwnd,RChart9,ChartMenuColor2);guiSetBgColor(hwnd,RChart10,ChartMenuColor2);guiSetBgColor(hwnd,RChart11,ChartMenuColor2);guiSetBgColor(hwnd,RChart12,ChartMenuColor2);
guiSetBgColor(hwnd,RChart13,ChartMenuColor2);guiSetBgColor(hwnd,RChart14,ChartMenuColor2);guiSetBgColor(hwnd,RChart15,ChartMenuColor2);guiSetBgColor(hwnd,RChart16,ChartMenuColor2);
if(timeframe == MenuPeriod1) guiSetChecked(hwnd, RChart1, true);
if(timeframe == MenuPeriod2) guiSetChecked(hwnd, RChart2, true);
if(timeframe == MenuPeriod3) guiSetChecked(hwnd, RChart3, true);
if(timeframe == MenuPeriod4) guiSetChecked(hwnd, RChart4, true);
if(timeframe == MenuPeriod5) guiSetChecked(hwnd, RChart5, true);
if(timeframe == MenuPeriod6) guiSetChecked(hwnd, RChart6, true);
if(timeframe == MenuPeriod7) guiSetChecked(hwnd, RChart7, true);
if(timeframe == MenuPeriod8) guiSetChecked(hwnd, RChart8, true);
if(timeframe2 == MenuPeriod1) guiSetChecked(hwnd, RChart9, true);
if(timeframe2 == MenuPeriod2) guiSetChecked(hwnd, RChart10, true);
if(timeframe2 == MenuPeriod3) guiSetChecked(hwnd, RChart11, true);
if(timeframe2 == MenuPeriod4) guiSetChecked(hwnd, RChart12, true);
if(timeframe2 == MenuPeriod5) guiSetChecked(hwnd, RChart13, true);
if(timeframe2 == MenuPeriod6) guiSetChecked(hwnd, RChart14, true);
if(timeframe2 == MenuPeriod7) guiSetChecked(hwnd, RChart15, true);
if(timeframe2 == MenuPeriod8) guiSetChecked(hwnd, RChart16, true);
}
}
// MT4GUI functions to change terminal actions within GUI
//+-------------------------------------------------------------------------------------------+
void TerminalMenu()
{
if (terminalMenuOpen)
{
RemoveCPMT();
}
else
{
RemoveCPMC();
controlPanelMenuTerminal = guiAdd(hwnd,"label",gUIXPosition,gUIYPosition+40,120,80,"");
guiSetBgColor(hwnd,controlPanelMenuTerminal,TerminalMenuColor);
terminalMenuOpen=true;
// Radio Buttons for terminal functions
RTerm1 = guiAdd(hwnd,"radio",gUIXPosition,gUIYPosition+40,120,20,MenuPair1);
RTerm2 = guiAdd(hwnd,"radio",gUIXPosition,gUIYPosition+60,120,20,MenuPair2);
RTerm3 = guiAdd(hwnd,"radio",gUIXPosition,gUIYPosition+80,120,20,MenuPair3);
RTerm4 = guiAdd(hwnd,"radio",gUIXPosition,gUIYPosition+100,120,20,MenuPair4);
// Look and feel for terminal radio buttons
guiSetBgColor(hwnd,RTerm1,OrangeRed);guiSetBgColor(hwnd,RTerm2,TerminalMenuColor);guiSetBgColor(hwnd,RTerm3,PaleGreen);guiSetBgColor(hwnd,RTerm4,Tomato);
if(TradeStatus == 0) guiSetChecked(hwnd, RTerm1, true);
if(TradeStatus == 1) guiSetChecked(hwnd, RTerm2, true);
if(TradeStatus == 2) guiSetChecked(hwnd, RTerm3, true);
if(TradeStatus == 3) guiSetChecked(hwnd, RTerm4, true);
}
}
// MT4GUI functions to remove controlPanelMenuTerminal
//+-------------------------------------------------------------------------------------------+
void RemoveCPMC()
{
guiRemove(hwnd,controlPanelMenuChart);
guiRemove(hwnd,RChart1);guiRemove(hwnd,RChart2);guiRemove(hwnd,RChart3);guiRemove(hwnd,RChart4);
guiRemove(hwnd,RChart5);guiRemove(hwnd,RChart6);guiRemove(hwnd,RChart7);guiRemove(hwnd,RChart8);
guiRemove(hwnd,RChart9);guiRemove(hwnd,RChart10);guiRemove(hwnd,RChart11);guiRemove(hwnd,RChart12);
guiRemove(hwnd,RChart13);guiRemove(hwnd,RChart14);guiRemove(hwnd,RChart15);guiRemove(hwnd,RChart16);
chartMenuOpen=false;
}
// MT4GUI functions to remove controlPanelMenuChart
//+-------------------------------------------------------------------------------------------+
void RemoveCPMT()
{
guiRemove(hwnd,controlPanelMenuTerminal);
guiRemove(hwnd,RTerm1);guiRemove(hwnd,RTerm2);guiRemove(hwnd,RTerm3);guiRemove(hwnd,RTerm4);
terminalMenuOpen=false;
}
// MT4GUI functions check radio buttons and change chart pair/period or execute terminal function
//+-------------------------------------------------------------------------------------------+
void CheckRadioButton()
{
if (guiIsChecked(hwnd, RChart1)) {timeframe = MenuPeriod1; }
if (guiIsChecked(hwnd, RChart2)) {timeframe = MenuPeriod2; }
if (guiIsChecked(hwnd, RChart3)) {timeframe = MenuPeriod3; }
if (guiIsChecked(hwnd, RChart4)) {timeframe = MenuPeriod4; }
if (guiIsChecked(hwnd, RChart5)) {timeframe = MenuPeriod5; }
if (guiIsChecked(hwnd, RChart6)) {timeframe = MenuPeriod6; }
if (guiIsChecked(hwnd, RChart7)) {timeframe = MenuPeriod7; }
if (guiIsChecked(hwnd, RChart8)) {timeframe = MenuPeriod8; }
if (guiIsChecked(hwnd, RChart9)) {timeframe2 = MenuPeriod1; }
if (guiIsChecked(hwnd, RChart10)) {timeframe2 = MenuPeriod2; }
if (guiIsChecked(hwnd, RChart11)) {timeframe2 = MenuPeriod3; }
if (guiIsChecked(hwnd, RChart12)) {timeframe2 = MenuPeriod4; }
if (guiIsChecked(hwnd, RChart13)) {timeframe2 = MenuPeriod5; }
if (guiIsChecked(hwnd, RChart14)) {timeframe2 = MenuPeriod6; }
if (guiIsChecked(hwnd, RChart15)) {timeframe2 = MenuPeriod7; }
if (guiIsChecked(hwnd, RChart16)) {timeframe2 = MenuPeriod8; }
//If Radio terminal buttons is checked call terminal function
if (guiIsChecked(hwnd, RTerm1)) {TradeStatus=0;} //Minimize terminal
if (guiIsChecked(hwnd, RTerm2)) {TradeStatus=1;} //Maximize terminal
if (guiIsChecked(hwnd, RTerm3)) {TradeStatus=2;} //Close terminal
if (guiIsChecked(hwnd, RTerm4)) {TradeStatus=3;} //Close chart
}
// MT4GUI Function for getting Chart Width and postition X/Y for ControlPanel (GUI)
//+-------------------------------------------------------------------------------------------+
void AutoPositionControlPanel()
{
gUIXPosition = guiGetChartWidth(hwnd)+GUIXOffset;
gUIYPosition = GUIYOffset;
}
// MT4/MQL4 Function to convert period number to text string
//+-------------------------------------------------------------------------------------------+
string PeriodToText(int period)
{
switch (period)
{
case 1:
return("M1");
break;
case 5:
return("M5");
break;
case 15:
return("M15");
break;
case 30:
return("M30");
break;
case 60:
return("H1");
break;
case 240:
return("H4");
break;
case 1440:
return("D1");
break;
case 10080:
return("W1");
break;
case 43200:
return("MN1");
break;
}
}
void StatusPrint(){
if(TradeStatus==0){
guiPrintXY(MenuPair1,OrangeRed,gUIXPosition,gUIYPosition-60,14,"Arial Bold");
}
if(TradeStatus==1){
guiPrintXY(MenuPair2,RoyalBlue,gUIXPosition,gUIYPosition-60,14,"Arial Bold");
}
if(TradeStatus==2){
guiPrintXY(MenuPair3,PaleGreen,gUIXPosition,gUIYPosition-60,14,"Arial Bold");
}
if(TradeStatus==3){
guiPrintXY(MenuPair4,Tomato,gUIXPosition,gUIYPosition-60,14,"Arial Bold");
}
guiPrintXY(timeframe,LightGreen,gUIXPosition,gUIYPosition-35,14,"Arial Bold");
guiPrintXY(timeframe2,LightBlue,gUIXPosition+60,gUIYPosition-35,14,"Arial Bold");
}