1. I noticed that in the OnChartEvent handler you modified the values of panelXX and panelYY and call ChartRedraw().
But it won't move the objects because after the objects were created, there is no relationship between panelXX/panelYY and the objects' coordinates.
2. The Standard Control classes can handle the mouse events very well and you don't have to do it by yourself. The first line in your code is already enough to handle the OnChartEvent. That's the reason why you can move the panel successfully :)
3. The trick is that you need to add the "BidColor", "BidLabel", "AskColor" and "AskLabel" as the child controls of the "myPanel". This can be done by the "myPanel.Add()" function. I modified your code a little for your reference:
int OnInit() { //--- Panel create myPanel.Create(0, "TEST PANEL", 0, panelXX, panelYY, panelWidth, panelHeight); //--- Bid label BidColor.Create(0,"Bid Background Color",0,5,25,panelWidth-45,45); BidColor.ColorBackground(clrYellow); myPanel.Add(BidColor); BidLabel.Create(0,"Bid Text",0,8,25,0,0); BidLabel.Text("Bid "+DoubleToString(SymbolInfoDouble(_Symbol,SYMBOL_BID),_Digits)); BidLabel.Color(clrBlue); myPanel.Add(BidLabel); //--- Ask label AskColor.Create(0,"Ask Background Color",0,5,105,panelWidth-45,125); AskColor.ColorBackground(clrAqua); myPanel.Add(AskColor); AskLabel.Create(0,"Ask Text",0,8,105,0,0); AskLabel.Text("Ask "+DoubleToString(SymbolInfoDouble(_Symbol,SYMBOL_ASK),_Digits)); AskLabel.Color(clrRed); myPanel.Add(AskLabel); //--- Run panel myPanel.Run(); //--- Define frequência do timer EventSetTimer(1); //--- Return return(0); }
The highlighted part is my modification. They simply did the following:
1. Change the label and the background coordinates to relative coordinates (remove the panelXX, panelYY), modify the panelWidth-25 to panelWidth-45, otherwise it is too wide to fit in the myPanel dialog box.
2. Call myPanel.Add() to add the controls as myPanel's child controls.
And the OnChartEvent() can be modified as simple as:
void OnChartEvent(const int id, const long &lparam, const double &dparam, const string &sparam) { //--- myPanel.ChartEvent(id, lparam, dparam, sparam); //--- }
I tested the modified code in my environment and it works fine :)
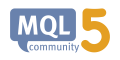
- www.mql5.com
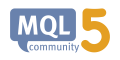
Such a fast and high-level answer is exactly what makes this community so professional !!!
Thanks a lot, Jian! Works perfectly !!!

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi, I'm trying to create a simple panel with texts, colors and dynamic information inside it...
I'm using MQL5's standard library to do most of the tasks, and I'm actually able to create the panel itself and some content inside the panel.
However, when I click and drag the panel with the mouse, only the panel itself is moving, while the labels and background colors inside the panel are fixed.
I'm showing below the code and I really don't know what I'm doing wrong, but I believe it has something to do with the CHARTEVENT_OBJECT_DRAG option inside OnChartEvent().
I trully appreciate any help! Thanks!