Please see:
https://www.mql5.com/en/docs/check/mqlinfointeger
For information about the type of the running program, values of ENUM_PROGRAM_TYPE are used.
Identifier |
Description |
PROGRAM_SCRIPT |
Script |
PROGRAM_EXPERT |
Expert |
PROGRAM_INDICATOR |
Indicator |
Example:
switch(mql_program)
{
case PROGRAM_SCRIPT:
{
Print(__FILE__+" is script");
break;
}
case PROGRAM_EXPERT:
{
Print(__FILE__+" is Expert Advisor");
break;
}
case PROGRAM_INDICATOR:
{
Print(__FILE__+" is custom indicator");
break;
}
default:Print("MQL5 program type value is ",mql_program);
}
And if you want to remove the expert:
ExpertRemove
The function stops an Expert Advisor and unloads it from a chart.
https://www.mql5.com/en/docs/common/expertremove
WindowExpertName
Returns the name of the executed Expert Advisor, script, custom indicator, or library.
Parameters
None.
Returned value
The name of the executed Expert Advisor, script, custom indicator, or library, depending on the MQL4 program, from which this function has been called.
Example:
GlobalVariablesDeleteAll(name);
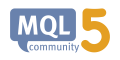
- www.mql5.com
Hi All,
How can know from EA that it's already running in the terminal?
The solution I'm thinking of is to set global variable onInit EA_ACTIVE and remove it on deInit, when you try to run another copy of EA it will check that EA_ACTIVE exists and return onInit_fail. The problem here that the user can easily delete this variable manually, or in some case this variable will not be deleted by deInit and will stay in terminal despite no EA is running.
Is there any MQL5 build-in function like TerminalGetRunningExpertsNames() to get running experts names...
Thanks
You have 2 options.
1. You do not actually need to remove expert adviser from a chart if you think this is unwanted copy. Instead you can disable all copies of EA except one of them (just stop trading). Anyway to enforce a single instance of EA you need to write a unique signature of every EA instance into the same global variable (the signatures can be generated as random numbers or taken from window handles). EA should write its signatue to the global variable from OnInit. Then EA should check if the global variable exists and contains its signature in OnTick or other events. If signature is different or does not exist, this copy of EA is blocked (or you can remove it, if you want), because this means that other copy was started.
2. You can enumerate all open charts and broadcast a custom event to them. In OnChartEvent handler, if the custom event is arrived, you also should stop this EA, because the fact of its sending means another copy of EA exists.
Here is a code example from a working product (MT4, for MT5 there exists a full equivalent):
int init()
{
//...
GlobalVariableSet(GLOBAL_NAME, WindowHandle(Symbol(), Period()));
return(0);
}
int DemoState = 0;
int start()
{
if(DemoState == 0)
{
if(GlobalVariableGet(GLOBAL_NAME) != WindowHandle(Symbol(), Period()))
{
DemoState = -1;
IndicatorShortName("Program is disabled (duplicate is found)");
Print("Only 1 instance is allowed. This one is terminated bacause other one is already running.");
// TODO: you can wipe out buffers in case of indicator or call ExpertRemove in EA
return(-1);
}
else
{
DemoState = +1;
}
}
else if(DemoState == -1)
{
return(-1);
}
//...
}

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi All,
How can know from EA that it's already running in the terminal?
The solution I'm thinking of is to set global variable onInit EA_ACTIVE and remove it on deInit, when you try to run another copy of EA it will check that EA_ACTIVE exists and return onInit_fail. The problem here that the user can easily delete this variable manually, or in some case this variable will not be deleted by deInit and will stay in terminal despite no EA is running.
Is there any MQL5 build-in function like TerminalGetRunningExpertsNames() to get running experts names...
Thanks