What's the best way to overcome the following warning when working under the OOP paradigm: "structure have objects and cannot be copied"?
laplacianlab:
...
Documentation says :
Simple Structures
Structures that do not contain strings or objects of dynamic arrays are called simple structures; variables of such structures can be freely copied to each other, even if they are different structures. Variables of simple structures, as well as their array can be passed as parameters to functions imported from DLL.
Your structure isn't a simple structure so you can't use it as returned value of a function. You have to use parameter (passed by reference) or pointer.
Documentation says :
Your structure isn't a simple structure so you can't use it as returned value of a function. You have to use parameter (passed by reference) or pointer.
I understand now: "structure have objects and cannot be copied" The message is clear! I am going to definie a class hierarchy and relate classes to each other. Maybe this is one solution, I am also having a look to this solution: https://www.mql5.com/en/docs/basis/types/object_pointers
Thank you for clarifying this.
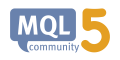
- www.mql5.com
Here it is the solution!
I have finally turned my graphic struct into a CGraphic class:
class CGraphic { public: string pair; CSymbolInfo symbol; ENUM_TIMEFRAMES timeframe; bar bars[]; };
Then I can create any class with dynamic properties of type CGraphic inside:
class CSimpleRandomWinner { private: CGraphic* _Graphic; // ... // Methods come here! CGraphic* GetGraphic();
And init those properties of complex type inside the constructor:
CSimpleRandomWinner::CSimpleRandomWinner()
{
_Graphic = new CGraphic();
}
With all the above I can program my getter method like this:
CGraphic* CSimpleRandomWinner::GetGraphic()
{
return(_Graphic);
}
I have made some tests and I think everyhing is ok right now.
Thank you very much!

- 2012.03.22
- MetaQuotes Software Corp.
- www.mql5.com
Here it is the solution!
I have finally turned my graphic struct into a CGraphic class:
Then I can create any class with dynamic properties of type CGraphic inside:
And init those properties of complex type inside the constructor:
With all the above I can program my getter method like this:
I have made some tests and I think everyhing is ok right now.
Thank you very much!

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I'm modelling a graphic in a MQL5 struct like this:
This is because later I want to define properties of type graphic in any class, for instance:
That's classical OOP! Of course, my app conceives things in real-world terms through to the use of TADs.
The problem is due to the property bar of the structure graphic which is a dynamic array. This is why I get the error "structure have objects and cannot be copied" in the methods that use that graphic structre, for example:
graphic CSimpleRandomWinner::GetGraphic() { return(_Graphic); }
This classical getter method fails when trying to return the private property graphic. I think that CSimpleRandomWinner would work in Java or C++, though I am not absolutely sure right now.
So my question is... What is the best way to work with MQL5 structs and classes that use dynamic arrays as properties? I think that struct graphic is a good example!! Can MQL5 treat graphic in the way I am showing in this thread? Maybe there's something that I am missing..
Thanks a lot for your help in advance!