//USE A GLOBAL VARIABLE
bool alert_buy_made=false;
bool alert_sell_made=false;
int start()
{
....
....
total = OrdersTotal();
if(total < 1)
{
if(cnt_buy ==2 && !alert_buy_made)
{
PlaySound("Alert.wav");
Alert("ALERT! Buy Now!! " + Symbol());
alert_buy_made =true;
}
if(cnt_sell == 2 && !alert_sell_made)
{
PlaySound("Alert.wav");
Alert("ALERT: Sell now!!! " + Symbol());
alert_sell_made=true;
}
}
//----
// this might have to be put other place.
alert_buy_made = (cnt_buy==2); // reset flag;
alert_sell_made = (cnt_sell==2); // reset flag;
return(0);
}
}
}
Ok, so I put in your modification and I'm getting 3-4 repeats from time to time but it's alot better than constant :) Here's what I have in now:
total = OrdersTotal();
if(total < 1)
{
if(cnt_buy ==2 && !alert_buy_made )
{
{
PlaySound("Alert.wav");
Alert("ALERT! Buy Now!! " + Symbol());
alert_buy_made =true;
}
alert1++;
}
if(cnt_sell == 2 && !alert_sell_made)
{
// Print("PRINT: Sell now...the conditions have been met");
PlaySound("Alert.wav");
Alert("ALERT: Sell now!!! " + Symbol());
alert_sell_made=true;
}
alert_buy_made = (cnt_buy==2); // reset flag;
alert_sell_made = (cnt_sell==2); // reset flag;
}
//----
return(0);
Probably other parts of the code may be modifying the values of cnt_buy and cnt_sell. If they are changed to a not 2 number, flags are set to false, enabling the alerts to fire, when the control variables are again assigned with value 2.
Well...cnt_buy and cnt_sell will vary between =0, 1, and 2.
The count changes based on the following code. Depending on what's going on...the "ADX(5) +DI" may be true so it will add 1 to the cnt_buy. If it's false it will be 0...and when it's true and SAR_DIRECTION= BOTTOM then it adds 1 to the counter and we end up with a buy scenario. So yes, it is changing above from 0 to 1 to 2 depending on the two indicators that I'm using.
int cnt_buy = 0;
int cnt_sell = 0;
if (
ADX5_DI_Plus_1Day < 11
) // good
cnt_buy++;
else
if (
ADX5_DI_Minus_1hr < 11 // good
)
cnt_sell++;
// And this too.....
if (SAR_DIRECTION == "BOTTOM")
cnt_buy++; // should = 5 by now...all conditions have been met
else
if (SAR_DIRECTION == "TOP")
cnt_sell++; //should = 2
Well...cnt_buy and cnt_sell will vary between =0, 1, and 2.
The count changes based on the following code. Depending on what's going on...the "ADX(5) +DI" may be true so it will add 1 to the cnt_buy. If it's false it will be 0...and when it's true and SAR_DIRECTION= BOTTOM then it adds 1 to the counter and we end up with a buy scenario. So yes, it is changing above from 0 to 1 to 2 depending on the two indicators that I'm using.
int cnt_buy = 0;
int cnt_sell = 0;
if (
ADX5_DI_Plus_1Day < 11
) // good
cnt_buy++;
else
if (
ADX5_DI_Minus_1hr < 11 // good
)
cnt_sell++;
// And this too.....
if (SAR_DIRECTION == "BOTTOM")
cnt_buy++; // should = 5 by now...all conditions have been met
else
if (SAR_DIRECTION == "TOP")
cnt_sell++; //should = 2
Yeah I just verified that it's not giving me true alerts :(
An alert poped up telling me to buy and yet the counter was "0". Conditions were'nt met...but it still sent the alert.
//DELETE THESE LINES
alert_buy_made = (cnt_buy==2); // reset flag;
alert_sell_made = (cnt_sell==2); // reset flag;
// You might have a buy/sell section in your EA, so adjust the code to something like this:
...
if(now_performing_buy)
{
OrderSend(.....); // make the trade
alert_buy_made = false; // enable more alerts;
}
....
if(now_performing_sell)
{
OrderSend(.....); // make the trade
alert_buy_made = false; // enable more alerts;
}
In relation to not giving true results, you must realize that code works deterministically. That is, if you have the statement "if(cnt_buy ==2 && !alert_buy_made ){.... }", it only makes the alert IF AND ONLY IF cnt_buy=2 and alert_buy_made=false, and no chance to fire in other situation.
I can help you more if take a look to your complete code.
If you dont mind post it here, or send it to abstract.mind@hotmail.com
to the Moderator:
Hi Rosh, is it ok to post my email?
Have the same question. Can you help me
here is my code.
//+------------------------------------------------------------------+
//| AlertRSI.mq5 |
//| Copyright 2024, MetaQuotes Ltd. |
//| https://www.mql5.com |
//+------------------------------------------------------------------+
#property copyright "Trang Candy +84971007267 "
#property link "Telegramchanel: https://t.me/rido_19, Telegram, Zalo Group: +84971007267;"
#property version "1.00"
#property strict
#property description "Strategists: Trang (WIFX)"
//+------------------------------
//------------------------------------+
//| Expert initialization function |
//+------------------------------------------------------------------+
input group "Alerts"
input string InpSoundName = "alert.wav"; // Sound Name
input uchar InpSoundRepetitions = 3; // Repetitions
input uchar InpSoundPause = 3; // Pause, in seconds
input bool InpUseSound = false; // Use Sound
input bool InpUseAlert = true; // Use Alert
input bool InpUseMail = true; // Use Send mail
input bool InpUseNotification = true; // Use Send notification
input int MaximumAlarm = 5;
int countAlarm = 0;
int OnInit()
{
//--- create timer
EventSetTimer(60);
//---
return(INIT_SUCCEEDED);
}
//+------------------------------------------------------------------+
//| Expert tick function |
//+------------------------------------------------------------------+
void OnTick()
{
countAlarm = 0;
string alertText = "";
if (getAlertRSI(PERIOD_W1) != ""){
alertText = alertText + getAlertRSI(PERIOD_W1);
}
if (getAlertRSI(PERIOD_D1) != ""){
alertText = alertText + getAlertRSI(PERIOD_D1);
}
if (getAlertRSI(PERIOD_H4) != ""){
alertText = alertText + getAlertRSI(PERIOD_H4);
}
if (getAlertRSI(PERIOD_H1) != ""){
alertText = alertText + getAlertRSI(PERIOD_H1);
}
if (getAlertRSI(PERIOD_M30) != ""){
alertText = alertText + getAlertRSI(PERIOD_M30);
}
if (getAlertRSI(PERIOD_M15) != ""){
alertText = alertText + getAlertRSI(PERIOD_M15);
}
if (getAlertRSI(PERIOD_M5) != ""){
alertText = alertText + getAlertRSI(PERIOD_M5);
}
while(countAlarm <= MaximumAlarm )
{
sendAlert(alertText);
countAlarm++;
printf(" countAlarm()" + countAlarm);
// if (countAlarm > MaximumAlarm){ countAlarm = 0;}
}
}
string getAlertRSI(ENUM_TIMEFRAMES time_frame){
string alertText="";
int getRSI;
double arrayRSI[];
double rsi;
getRSI = iRSI(_Symbol, time_frame, 14, PRICE_CLOSE);
ArraySetAsSeries(arrayRSI, true );
CopyBuffer(getRSI,0,0,2,arrayRSI);
rsi = NormalizeDouble(arrayRSI[1], _Digits);
if (rsi <=32)
{
alertText = " KHUNG: " + getPeriodText(time_frame) + ", RSI = " + rsi + ". ";
} else if (rsi>=70)
{
alertText = " KHUNG: " + getPeriodText(time_frame) + ", RSI = " + rsi + ". ";
}
return alertText;
}
void sendAlert(string alertText){
if (alertText != ""){
if(InpUseSound)
PlaySound(InpSoundName);
if(InpUseAlert)
Alert(alertText);
if(InpUseNotification)
SendNotification(Symbol()+"," + alertText);
}
}
string getPeriodText(ENUM_TIMEFRAMES time_frame){
if (time_frame == 5) return "M5";
else if (time_frame == 15) {
return "M15";
} else if (time_frame == 30) {
return "M30";
} else if (time_frame == 16385) {
return "H1";
} else if (time_frame == 16388){
return "H4";
} else if (time_frame == 16408){
return "D1";
} else if (time_frame == 32769) {
return "W1";
} else if (time_frame == 49153) {
return "MN1";}
return "";
}
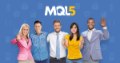
- 2024.05.31
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
The problem is that it's sending too many Alerts because the conditions are true for a long time. How can I get it to only send me one alert when it finds the conditions are true? Below is my code:
total = OrdersTotal(); if(total < 1) { if(cnt_buy ==2) { PlaySound("Alert.wav"); Alert("ALERT! Buy Now!! " + Symbol()); } if(cnt_sell == 2) { PlaySound("Alert.wav"); Alert("ALERT: Sell now!!! " + Symbol()); } } //---- return(0); }