edddim wrote:
When there is local function variable initialized example: _localFunction ( int function_Variable ){}
it is not available to other functions but it's taking space in memory, with every call of that function, variable is initialized again.
My question is does the every call of the function variable is taking new space of available memory/cache or the previous one catch is cleared?
When there is local function variable initialized example: _localFunction ( int function_Variable ){}
it is not available to other functions but it's taking space in memory, with every call of that function, variable is initialized again.
My question is does the every call of the function variable is taking new space of available memory/cache or the previous one catch is cleared?
I guess local variable storage is implemented using a sort of stack, so every time you call a function its parameters are pushed onto the stack (i.e. some memory is allocated on the stack). When the function terminates all local variables including the function parameters are removed from the stack (thus the allocated memory is freed).
edddim:
When there is local function variable initialized example: _localFunction ( int function_Variable ){}
it is not available to other functions but it's taking space in memory, with every call of that function, variable is initialized again.
My question is does the every call of the function variable is taking new space of available memory/cache or the previous one catch is cleared?
When there is local function variable initialized example: _localFunction ( int function_Variable ){}
it is not available to other functions but it's taking space in memory, with every call of that function, variable is initialized again.
My question is does the every call of the function variable is taking new space of available memory/cache or the previous one catch is cleared?
edddim - what you are referring to is not a local variable but an input parameter to the function. Below is an example of a function with a local variable iMinStopLevel (it also has 2 input parameters: int iOp and double fSuggestedEntryLevel ):
//+------------------------------------------------------------------+ // make sure the buystop or sellstop levels are not too close to market double AdjustStopOrder(int iOp, double fSuggestedEntryLevel) { int iMinStopLevel = MarketInfo( Symbol(), MODE_STOPLEVEL ); if( OP_BUYSTOP == iOp ) { if( fSuggestedEntryLevel <= (Ask+iMinStopLevel*Point) ) { fSuggestedEntryLevel = Ask+(iMinStopLevel+1)*Point; } } else if( OP_SELLSTOP == iOp ) { if( fSuggestedEntryLevel >= (Bid-iMinStopLevel*Point) ) { fSuggestedEntryLevel = Bid-(iMinStopLevel+1)*Point; } } else { Alert("AdjustStopOrder: Invalid Operation code ", iOp); return (0.0); } return ( NormalizeDouble(fSuggestedEntryLevel, Digits) ); }In most programming languages input parameters and local variables are implemented by using the stack. Sometimes compilers may optimize these and use CPU registers for input parameters or local variables or both.
Unless you funciton is recursive, you don't need to worry about your input params or local vars wasting memory each time the funciton is called - as soon as your function is done this memory will be available again for other functions to use. If you don't know what a recursive function is then, again - don't worry about this because it will not be a problem for you.
Recursive --> function to call itself. I know, it's a giant eater of memory.
No, I have code like this:
//---- market change=0; while(change<1){ if(IsTradeAllowed())_market(); //---- routines _routine();} return(0);} //+----------------------------- market ----------------------------+ int _market(){ if(MarketInfo(simbol,9)!=mi[s]){ mi[s]=MarketInfo(simbol,9);change=-1;} return(change+1);} //+---------------------------- routine ----------------------------+ int _routine(){ _conditions(); _trades(); _mails(); return(0);}I am trying to eliminate extra memory usage.
the only possible problem I see in your code is that you seem to be using global
variables for all your variables (which is not a very good practice). Also - you
are returning change+1 from _market (even though it is a global variable which
is available throughout your program) but you are not assigning this returned value
to anything. These are not bugs - just inconsistencies in you programming. They
may lead to bugs in the future, though, so beter try eliminate them.
If change and s are not global variables, e.g. if change and s are local variables of the "start" funciton then you have a problem and I don't think your program would work...
In terms of using less memory - using global variables should actually reduce your memory usage because your variables are all in the "initialized data" memory, which unlike the stack does not change it's size with every funciton call. But I would not switch all my functions to use global variables because of this. It will make code a mess and is not worth it.
Why are you trying to reduce your memory usage? Are you're having memory problems? If yes - why do you think so?
If change and s are not global variables, e.g. if change and s are local variables of the "start" funciton then you have a problem and I don't think your program would work...
In terms of using less memory - using global variables should actually reduce your memory usage because your variables are all in the "initialized data" memory, which unlike the stack does not change it's size with every funciton call. But I would not switch all my functions to use global variables because of this. It will make code a mess and is not worth it.
Why are you trying to reduce your memory usage? Are you're having memory problems? If yes - why do you think so?
The program (expert) is working. They are "all" global variables, and
when they were not there was more slowing down and memory picks. Also and indicator
is working on same principe fine. That loop where is "change", before
was *infinite*.
I have posted some screen shots of indicator here, I am just making now more functions separate as I have noticed improvements in speed and memory.
If you want to see results of testing my first expert:
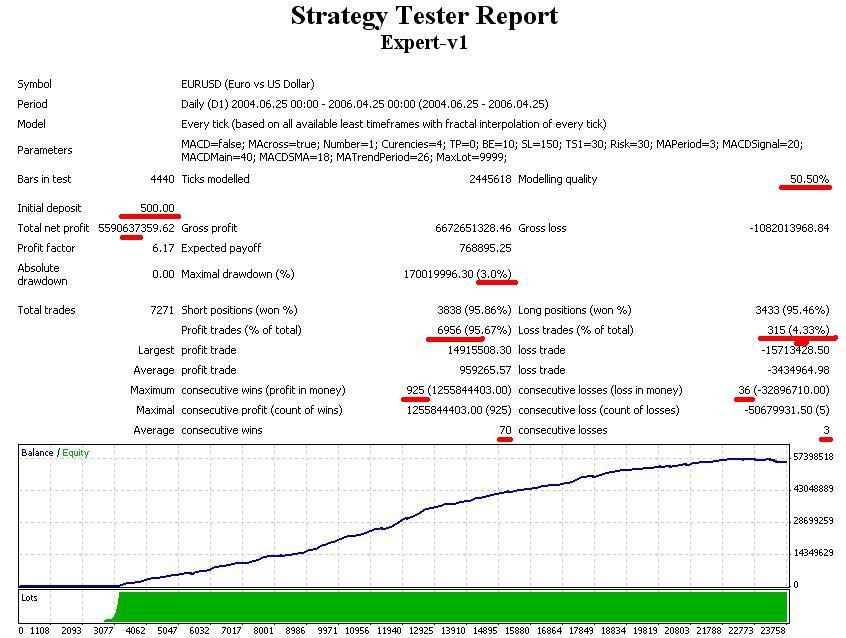
Also my first indicator:
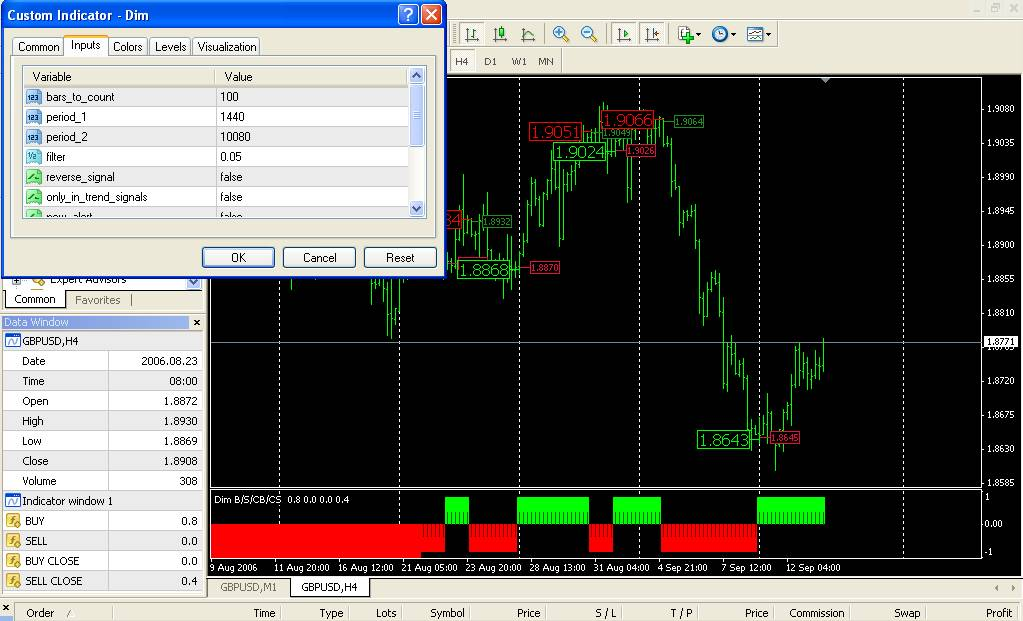
Full report of the expert you can view here, I want to point your attention on date of the rar file was created. I don't think mql4 has bugs.
I have posted some screen shots of indicator here, I am just making now more functions separate as I have noticed improvements in speed and memory.
If you want to see results of testing my first expert:
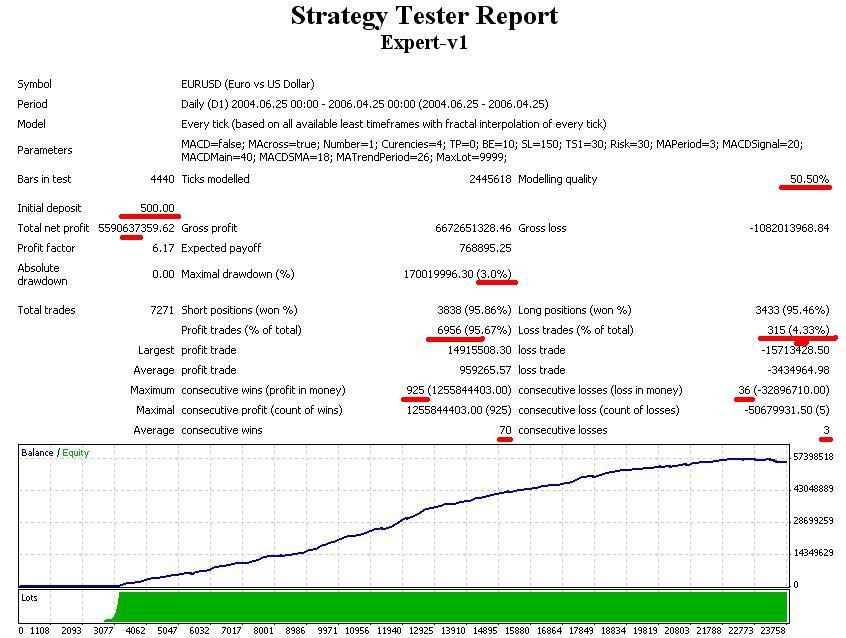
Also my first indicator:
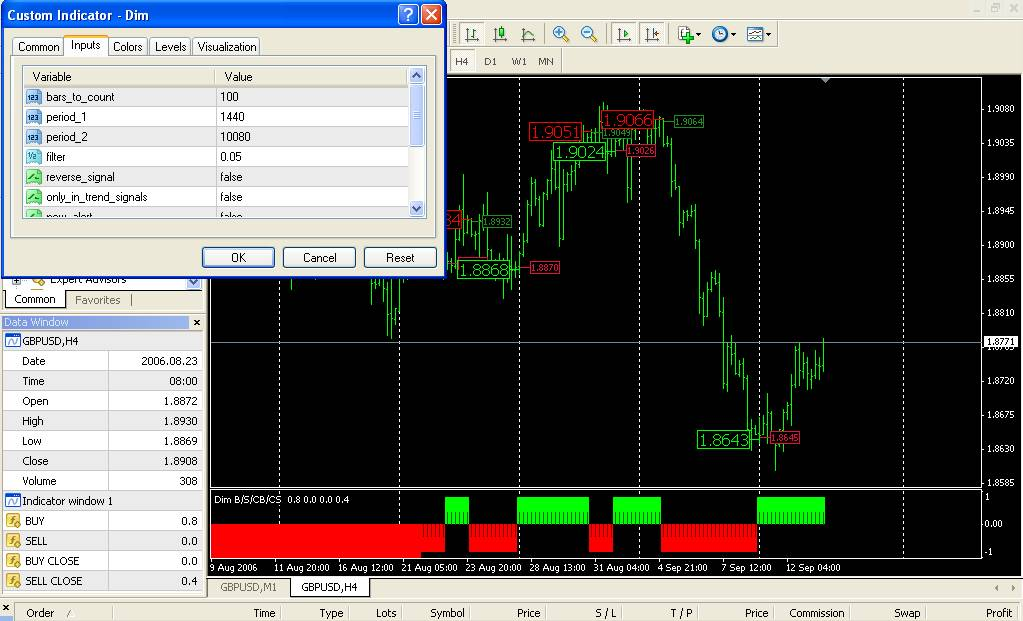
Full report of the expert you can view here, I want to point your attention on date of the rar file was created. I don't think mql4 has bugs.
4x4ever wrote:
... But I would not switch all my functions to use global variables because of this. It will make code a mess and is not worth it...
This is the same expert as previous with only global variables changed ==> Modelling
quality 30.1%.... But I would not switch all my functions to use global variables because of this. It will make code a mess and is not worth it...
End result is lower profit achieved than the testing with 50.50%.
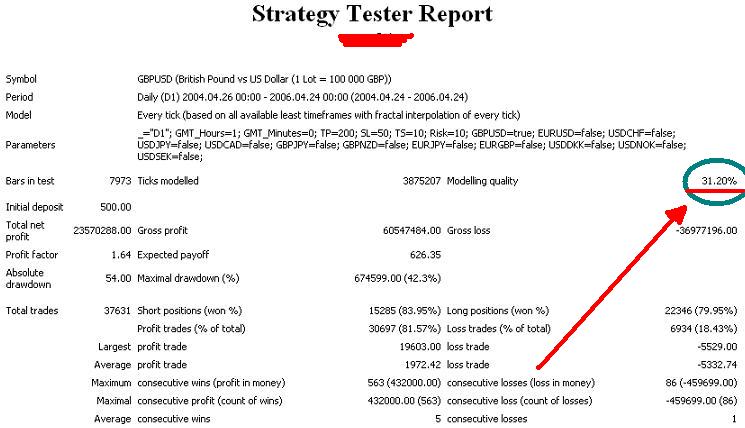
As expected higher modeling quality would achieve lower results if the calculations or code were not good.
So it is not always modeling quality and bars, it is how you writte the code. :)

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
it is not available to other functions but it's taking space in memory, with every call of that function, variable is initialized again.
My question is does the every call of the function variable is taking new space of available memory/cache or the previous one catch is cleared?