- Bitte editiere Dein Post, sodass der Code als Code (mit Alt+S oder dem Icon </> aus der Editierzeile) formatiert wird - ist für uns leichter lesbar.
- Was ist 5P?
- Kontrolliere bei solchen Problemen Bid,Ask,SL StopLoss und TP(TakeProfit) mit dem Debugger: https://www.mql5.com/de/articles/654 // Zur Fehlerbehebung von MQL5-Programmen
- In der Hilfe (F1) gibt es viele Beispiele, von denen man einfach kopieren kann.
- Sonst in der CodeBase suchen: oben rechts die Lupe: "modify", dann links auf CodeBase klicken.
- Hier in den Beispielen gibt es funktionierende Beispiel-EAs, zB. den MACD "..\Experts\Examples\MACD\MACD Sample.mq5"
Sind so ne Art Forumsregeln.
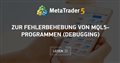
Zur Fehlerbehebung von MQL5-Programmen (Debugging)
- www.mql5.com
Dieser Artikel richtet sich primär an Programmierer, die die Sprache zwar bereits gelernt haben, die allerdings noch keine Meister ihres Fachs sind. Er wird auf verschiedene Debugging-Techniken eingehen, die der gebündelten Erfahrung des Autors sowie vieler anderer Programmierer entspringen.

Sie verpassen Handelsmöglichkeiten:
- Freie Handelsapplikationen
- Über 8.000 Signale zum Kopieren
- Wirtschaftsnachrichten für die Lage an den Finanzmärkte
Registrierung
Einloggen
Sie stimmen der Website-Richtlinie und den Nutzungsbedingungen zu.
Wenn Sie kein Benutzerkonto haben, registrieren Sie sich
Hallo,
ich will in diesem Code einen SL einbinden, der sofort nach Eröffnung einer Order greift, falls die 5P negativ wird.
Leider bin ich noch nicht soweit dies selbst einzubinden.
Kann mir jemand dabei helfen?
MfG
Hier der Code
#include <Trade\PositionInfo.mqh>
#include <Trade\Trade.mqh>
#include <Trade\SymbolInfo.mqh>
CPositionInfo m_position; // trade position object
CTrade m_trade; // trading object
CSymbolInfo m_symbol; // symbol info object
//--- input parameters
input double InpLots = 0.1; // Lots
input ushort InpTakeProfit = 50; // Virtual Take Profit (in pips)
input ushort InpStep = 15; // Step (in pips)
input ushort InpStoploss =5; wurde von mir eingefügt, aber es scheint was zu fehlen
input ulong m_magic = 101175802;// magic number
//---
ulong m_slippage=10; // slippage
double ExtTakeProfit=0.0;
double ExtStoploss=0.0;
double ExtStep=0.0;
double m_adjusted_point; // point value adjusted for 3 or 5 points
double price_last_deal_buy=0.0;
double price_last_deal_sell=0.0;
//+------------------------------------------------------------------+
//| Expert initialization function |
//+------------------------------------------------------------------+
int OnInit()
{
//---
if(!m_symbol.Name(Symbol())) // sets symbol name
return(INIT_FAILED);
RefreshRates();
string err_text="";
if(!CheckVolumeValue(InpLots,err_text))
{
Print(__FUNCTION__,", ERROR: ",err_text);
return(INIT_PARAMETERS_INCORRECT);
}
//---
m_trade.SetExpertMagicNumber(m_magic);
m_trade.SetMarginMode();
m_trade.SetTypeFillingBySymbol(m_symbol.Name());
//---
m_trade.SetDeviationInPoints(m_slippage);
//--- tuning for 3 or 5 digits
int digits_adjust=1;
if(m_symbol.Digits()==3 || m_symbol.Digits()==5)
digits_adjust=10;
m_adjusted_point=m_symbol.Point()*digits_adjust;
ExtTakeProfit = InpTakeProfit* m_adjusted_point;
ExtStep = InpStep * m_adjusted_point;
//---
return(INIT_SUCCEEDED);
}
//+------------------------------------------------------------------+
//| Expert deinitialization function |
//+------------------------------------------------------------------+
void OnDeinit(const int reason)
{
//---
}
//+------------------------------------------------------------------+
//| Expert tick function |
//+------------------------------------------------------------------+
void OnTick()
{
if(price_last_deal_buy==0.0 && price_last_deal_sell==0.0)
{
m_trade.Buy(InpLots);
return;
}
//---
if(!RefreshRates())
return;
//--- signal buy
if(price_last_deal_buy==0.0 || (price_last_deal_buy!=0.0 && price_last_deal_buy-m_symbol.Ask()>=ExtStep))
{
m_trade.Buy(InpLots);
return;
}
//--- signal sell
if(price_last_deal_sell==0.0 || (price_last_deal_sell!=0.0 && m_symbol.Bid()-price_last_deal_sell>=ExtStep))
{
m_trade.Sell(InpLots);
return;
}
//--- virtual take profit
bool close=false;
for(int i=PositionsTotal()-1;i>=0;i--)
if(m_position.SelectByIndex(i)) // selects the position by index for further access to its properties
if(m_position.Symbol()==m_symbol.Name() && m_position.Magic()==m_magic)
{
if(m_position.PositionType()==POSITION_TYPE_BUY)
if(m_position.PriceCurrent()-m_position.PriceOpen()>=ExtTakeProfit)
{
m_trade.PositionClose(m_position.Ticket()); // close a position by the specified symbol
close=true;
}
if(m_position.PositionType()==POSITION_TYPE_SELL)
if(m_position.PriceOpen()-m_position.PriceCurrent()>=ExtTakeProfit)
{
m_trade.PositionClose(m_position.Ticket()); // close a position by the specified symbol
close=true;
}
}
if(close)
return;
//---
int count_buys=0,count_sells=0;
CalculatePositions(count_buys,count_sells);
if(count_buys==0.0 && count_sells!=0.0)
price_last_deal_buy=0.0;
else if(count_buys!=0.0 && count_sells==0.0)
price_last_deal_sell=0.0;
}
//+------------------------------------------------------------------+
//| TradeTransaction function |
//+------------------------------------------------------------------+
void OnTradeTransaction(const MqlTradeTransaction &trans,
const MqlTradeRequest &request,
const MqlTradeResult &result)
{
double res=0.0;
int losses=0.0;
//--- get transaction type as enumeration value
ENUM_TRADE_TRANSACTION_TYPE type=trans.type;
//--- if transaction is result of addition of the transaction in history
if(type==TRADE_TRANSACTION_DEAL_ADD)
{
long deal_ticket =0;
long deal_order =0;
long deal_time =0;
long deal_time_msc =0;
long deal_type =-1;
long deal_entry =-1;
long deal_magic =0;
long deal_reason =-1;
long deal_position_id =0;
double deal_volume =0.0;
double deal_price =0.0;
double deal_commission =0.0;
double deal_swap =0.0;
double deal_profit =0.0;
string deal_symbol ="";
string deal_comment ="";
string deal_external_id ="";
if(HistoryDealSelect(trans.deal))
{
deal_ticket =HistoryDealGetInteger(trans.deal,DEAL_TICKET);
deal_order =HistoryDealGetInteger(trans.deal,DEAL_ORDER);
deal_time =HistoryDealGetInteger(trans.deal,DEAL_TIME);
deal_time_msc =HistoryDealGetInteger(trans.deal,DEAL_TIME_MSC);
deal_type =HistoryDealGetInteger(trans.deal,DEAL_TYPE);
deal_entry =HistoryDealGetInteger(trans.deal,DEAL_ENTRY);
deal_magic =HistoryDealGetInteger(trans.deal,DEAL_MAGIC);
deal_reason =HistoryDealGetInteger(trans.deal,DEAL_REASON);
deal_position_id =HistoryDealGetInteger(trans.deal,DEAL_POSITION_ID);
deal_volume =HistoryDealGetDouble(trans.deal,DEAL_VOLUME);
deal_price =HistoryDealGetDouble(trans.deal,DEAL_PRICE);
deal_commission =HistoryDealGetDouble(trans.deal,DEAL_COMMISSION);
deal_swap =HistoryDealGetDouble(trans.deal,DEAL_SWAP);
deal_profit =HistoryDealGetDouble(trans.deal,DEAL_PROFIT);
deal_symbol =HistoryDealGetString(trans.deal,DEAL_SYMBOL);
deal_comment =HistoryDealGetString(trans.deal,DEAL_COMMENT);
deal_external_id =HistoryDealGetString(trans.deal,DEAL_EXTERNAL_ID);
}
else
return;
if(deal_reason!=-1)
int d=0;
if(deal_symbol==Symbol() && deal_magic==m_magic)
if(deal_entry==DEAL_ENTRY_IN)
{
if(deal_type==DEAL_TYPE_BUY)
price_last_deal_buy=deal_price;
if(deal_type==DEAL_TYPE_SELL)
price_last_deal_sell=deal_price;
}
}
}
//+------------------------------------------------------------------+
//| Refreshes the symbol quotes data |
//+------------------------------------------------------------------+
bool RefreshRates(void)
{
//--- refresh rates
if(!m_symbol.RefreshRates())
{
Print("RefreshRates error");
return(false);
}
//--- protection against the return value of "zero"
if(m_symbol.Ask()==0 || m_symbol.Bid()==0)
return(false);
//---
return(true);
}
//+------------------------------------------------------------------+
//| Check the correctness of the position volume |
//+------------------------------------------------------------------+
bool CheckVolumeValue(double volume,string &error_description)
{
//--- minimal allowed volume for trade operations
double min_volume=m_symbol.LotsMin();
if(volume<min_volume)
{
error_description=StringFormat("Volume is less than the minimal allowed SYMBOL_VOLUME_MIN=%.2f",min_volume);
return(false);
}
//--- maximal allowed volume of trade operations
double max_volume=m_symbol.LotsMax();
if(volume>max_volume)
{
error_description=StringFormat("Volume is greater than the maximal allowed SYMBOL_VOLUME_MAX=%.2f",max_volume);
return(false);
}
//--- get minimal step of volume changing
double volume_step=m_symbol.LotsStep();
int ratio=(int)MathRound(volume/volume_step);
if(MathAbs(ratio*volume_step-volume)>0.0000001)
{
error_description=StringFormat("Volume is not a multiple of the minimal step SYMBOL_VOLUME_STEP=%.2f, the closest correct volume is %.2f",
volume_step,ratio*volume_step);
return(false);
}
error_description="Correct volume value";
return(true);
}
//+------------------------------------------------------------------+
//| Calculate positions Buy and Sell |
//+------------------------------------------------------------------+
void CalculatePositions(int &count_buys,int &count_sells)
{
count_buys=0;
count_sells=0;
for(int i=PositionsTotal()-1;i>=0;i--)
if(m_position.SelectByIndex(i)) // selects the position by index for further access to its properties
if(m_position.Symbol()==m_symbol.Name() && m_position.Magic()==m_magic)
{
if(m_position.PositionType()==POSITION_TYPE_BUY)
count_buys++;
if(m_position.PositionType()==POSITION_TYPE_SELL)
count_sells++;
}
//---
return;
}
//+------------------------------------------------------------------+