Du hast ein Problem mit den Indices der letzten Bars 0,1 .., rates_total-1 in MQL5 bzw rates_total-1, .. 1,0 in Mql4. Mir scheint Du musst da etwas umdrehen.
So entstehen wohl Wert gleich oder nahe Null so dass (Division durch Null) einmal unendlich oder etwas viel zu Großes und einmal Null im Indikatorpuffer steht.
Ich würde den Debugger verwenden - solange die Märkte noch zu sind - und ihn für die letzten 33 starten => if (time[i]>D'2018.09.28 23:40') DebugBreak();
BTW, ich würde in mql4 den Index der Bars von rates_total bis 0 laufen lassen, denn eigentlich ist bar #0 die aktuelle (in mql4) - check mal time[i] im Debugger!
Hast Du das bedacht:
PS: be aware that Henderson's filters are from a family of centered smoothers, i.e. they recalculate half period bars. (von https://www.mql5.com/en/code/21605)
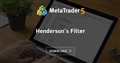
- www.mql5.com

- Freie Handelsapplikationen
- Über 8.000 Signale zum Kopieren
- Wirtschaftsnachrichten für die Lage an den Finanzmärkte
Sie stimmen der Website-Richtlinie und den Nutzungsbedingungen zu.
Hallo,
ich habe den Henderson Filter in MQL4 entwickelt, aber es gibt das Problem, dass es am Ende anscheinend Fehlberechnungen gibt. Ich habe lange Zeit gesucht und den Fehler nicht gefunden.