- Program types and their construction features
- Statistical analysis and fuzzy logic tools
- OpenCL: Parallel computations in MQL5
- Integration with Python
Program types and their construction features
The MetaTrader 5 platform package includes a modern development environment MetaEditor which enables the creation of various programs for algorithmic trading. Programs can be written in the specially designed programming language called MetaQuotes Language 5 (MQL5). The language syntax is closely aligned with C++, enabling programming in an object-oriented style. This facilitates the transition to using MQL5 for a large community of programmers.
The interaction of the MetaTrader 5 platform with programs is organized in such a way that the price movements of instruments and changes in the trading account are tracked by the platform. When predetermined changes occur, the platform generates events in the instrument chart open in the platform. When an event occurs, the user programs attached to the chart are checked. These can be software Expert Advisors, indicators, and scripts. Event handlers are defined in the platform for each event and program type.
An event handler is a special function defined by the MQL5 programming language. Such a function has a strictly specified name, return value type, and a list and type of parameters. Based on the return value type and the parameter types, the event handler of the client terminal identifies functions for processing the occurred event. If a function has parameters that do not match the predetermined ones or if a different return value type is specified, then such a function will not be used to process the event.
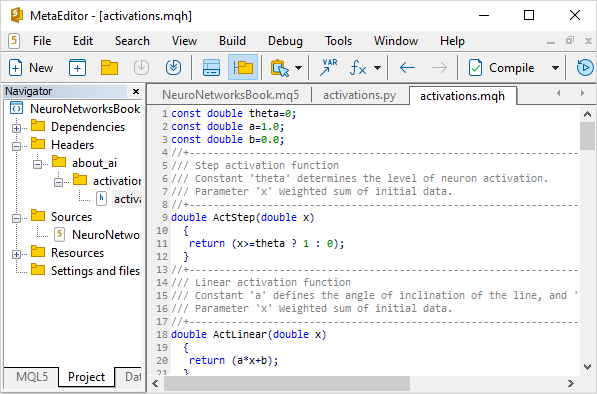
Built-in development environment MetaEditor
Each type of program can only handle certain events. Thus, if the event handler does not correspond to the program type, such a function will not be called by the terminal.
The MQL5 language includes a series of trading functions and predefined event handlers, which are used for Expert Advisors to allow them to execute the trading strategies embedded in them. It also offers an opportunity to write your own technical analysis indicators, scripts, services, and libraries of included functions.
Each program type is designed to perform its specific tasks and has special features of construction.
Expert Advisors (EAs)
Probably, at the forefront of algorithmic trading are Expert Advisors (trading robots) which are programs capable of independently analyzing the market and conducting trading operations based on a programmed strategy and its trading rules.
Technically, in MetaTrader 5, an Expert Advisor is tied to a specific chart on which it runs. In doing so, it only handles predefined events from this specific chart. The occurrence of each event triggers the corresponding functionality of the trading strategy. Among such events can be program launch and deinitialization, timer triggering, arrival of a new tick, scheduled events, and user events.
At the same time, the trading strategy of the Expert Advisor can include the analysis of other timeframes of the current instrument, as well as the analysis of any instrument in the terminal on any timeframe. This allows you to build multi-currency and multi-timeframe strategies.
In addition, Expert Advisors have the technical ability to receive data from any technical indicator installed in the terminal. This greatly expands the possibilities for building different trading strategies.
Each predefined event calls the corresponding EA function, in which the program code for event processing is written.
Immediately after launching the Expert Advisor, the terminal generates an Init event, which triggers the OnInit function. Global variables and objects are initialized in the body of this function. If necessary, a timer is started. The function has no input parameters but returns an integer value of the return code as a result of its execution. A non-zero return code indicates a failed initialization. In this case, the terminal generates a program termination event called Deinit.
//+------------------------------------------------------------------+ |
When the program is completed, the MetaTrader 5 terminal generates a Deinit event that triggers the execution of the OnDeinit function. The function has one input integer parameter which receives the code of the reason for the program termination. Inside the function body, if necessary, global variables, classes, and graphical objects are removed, data is saved in file resources, the timer initiated during program initialization is closed, and other operations required for the proper termination of the program and the cleanup of its traces in the terminal are performed.
//+------------------------------------------------------------------+ |
When a new tick arrives for the symbol chart on which the Expert Advisor is running, the NewTick event is generated. This triggers the OnTick function. This event is generated only for Expert Advisors, so the OnTick function will not be launched in other programs. Of course, the specified function can always be called forcibly from any place in the program, but it will no longer be the NewTick event processing.
The OnTick function has no input parameters and does not return any code. The main purpose of the function is to execute the price fluctuations handler in the advisor, which evaluates changes in the market situation and checks the rules of the embedded strategy for the need to perform any trading operations. Sometimes, according to the trading strategy rules, the Expert Advisor should perform operations not at every price movement, but, for example, at the opening of a new candlestick. In such cases, checking for the occurrence of the expected event is added to the OnTick function.
//+------------------------------------------------------------------+ |
If the algorithm of an Expert Advisor does not require the processing of each price movement but is based on the execution of cyclic operations with a certain time interval, even if there are no price movements observed during this time, the use of a timer can be very beneficial.
For this, when initializing the program in the OnInit function, it is necessary to initialize the timer using the EventSetTimer function. The function parameters specify the timer delay period in seconds. After that, the terminal will generate a Timer event for the chart, and the OnTimer function of the Expert Advisor will be launched for execution.
//+------------------------------------------------------------------+ |
When using a timer in the program's code, it is necessary to unload it from the terminal's memory upon program termination within the OnDeinit function. The EventKillTimer function is used for this purpose. This function has no parameters. It should be noted that the platform provides for the use of only one timer on the chart.
Within one EA, you can use both the OnTick and OnTimer functions, if necessary.
Among Expert Advisors, there is a subclass of semi-automatic Expert Advisors and trading panels. Such programs are not capable of independent trading without human intervention. Programs of this type perform trading operations at the trader's command. The programs themselves are designed to facilitate the trader's work and to take over some routine operations. This can be money management, setting stop loss and take profit levels, position maintenance, and much more.
The interaction between the program and the user is implemented through ChartEvent group events in Expert Advisors. These events trigger the execution of the OnChartEvent function, which accepts four parameters from the terminal:
- id: event identifier,
- lparam: event parameter of type long,
- dparam: event parameter of type double,
- sparam: event parameter of type string.
//+------------------------------------------------------------------+ |
The event can be generated for Expert Advisors and technical indicators. In this case, for each type of event, the input parameters of the function have certain values required to process the event.
Technical indicators
Another program type is a custom indicator. Indicators are programs that perform analytical functions to assist traders in conducting technical analysis of the market situation. During their operation, indicators process each price movement on the chart of their trading instrument. They can display various graphical objects, thus generating signals for subsequent analysis by the trader.
Like Expert Advisors, custom indicators can use data from other indicators, instruments, and timeframes in their calculations. But at the same time, indicators cannot perform trading operations. Thus, the indicator application scope is limited to the framework of technical analysis.
Similar to Expert Advisors, technical indicators have Init, Timer, and ChartEvent event handlers. The construction of functions for processing these events is similar to the corresponding functions of electronic Expert Advisors, but instead of the NewTick event, the Calculate event is generated for indicators. This event is handled by the OnCalculate function. There are two types of OnCalculate function depending on the scope of the indicator:
- shorthand
//+------------------------------------------------------------------+ |
- full
//+------------------------------------------------------------------+ |
Within a single indicator, you can only use one of the versions of the function.
Both versions of the OnCalculate function have parameters:
- rates_total: number of items in the timeseries,
- prev_calculated: number of recalculated elements of the time series at the previous run of the function.
The use of the prev_calculated parameter allows you to implement algorithms in which the indicator does not recalculate previously calculated historical values. This reduces the number of iterations in processing each new price fluctuation.
The work of indicators in the MetaTrader 5 terminal is organized as follows. The prev_calculated parameter receives the value that the function returned at the previous run. Therefore, in the general case, at the end of a successful function completion, it's sufficient to return the value of the rates_total parameter. If errors occur while the function is running, you can return the current value of prev_calculated. In this case, the function will start recalculating from the current location the next time it is run. If you return 0, upon the next launch, the indicator will be recalculated for the entire history, as it was during the initial launch.
When defined briefly, the function has only one input array of time series (price) and a parameter for shifting significant values relative to the beginning of the time series (begin). In this version, the calculation of indicator values is based on the data of one time series. Which time series will be used is set by the trader when launching the technical indicator. This can be either any of the price time series or the buffer values of another indicator.
When using the full version of the OnCalculate function, the function gets all price time series in its parameters. In such a case, the user does not have the option to choose a time series when launching the indicator. If it's necessary to use a buffer of data from another indicator, it needs to be explicitly written in the program code of the indicator.
In MetaTrader 5, there is a limitation in the ability to run only one Expert Advisor for each chart. If you need to run two or more Expert Advisors in one terminal, you should open an individual chart for each Expert Advisor. There is no such limitation for indicators as MetaTrader 5 allows you to use built-in and custom indicators in different versions in parallel on one chart of a trading instrument. The indicator can display data both on the price chart of the instrument itself and in sub-windows.
Scripts
Following launching, Expert Advisors and custom indicators remain in the terminal memory until they are forcibly closed by the trader. As certain events occur, the terminal launches the relevant functionality of Expert Advisors and indicators. Scripts are provided to perform any one-time operations. This is a separate type of program that does not handle any events other than its startup event.
Immediately after being launched, they perform the designated functionality and are unloaded from the terminal's memory. Along with Expert Advisors, scripts are able to perform trading operations, but it is impossible to run more than one script on a symbol chart at the same time.
There is only one OnStart event handler in the body of the script, which is launched immediately after the program starts. The OnStart function does not receive any parameters and does not return any codes.
//+------------------------------------------------------------------+ |
A separate program type is Services. Unlike the aforementioned types of programs, a service does not require binding to a specific price chart of a trading instrument. Just like scripts, services do not process any events other than their own launch. However, they are capable of generating custom events themselves and sending them to charts for further processing in Expert Advisors.
The MetaEditor development environment provides the possibility to create libraries and include files. These files are designed to store and distribute frequently used program blocks. Libraries are compiled files and provide individual functions for export to other running programs. The code of the executed functions itself is hidden. Plug-in files, unlike libraries, are open-source files. In terms of performance, it's preferable to use include files, but they do not ensure code secrecy during distribution.
In addition to event handlers, all programs can contain other functions and classes that will need to be called from the event handler functions. They can also have external input parameters set by the user when the program is launched.
Another technical aspect should also be taken into consideration. MetaTrader 5 is positioned as a platform with multi-threaded computing. In this case, three threads are allocated for each trading instrument's chart: one for the Expert Advisor, one for the script, and one for indicators. All indicators loaded onto one trading instrument's chart operate within the same thread as the chart itself. Therefore, it's not recommended to perform complex calculations within indicators.
Hence, we can use EAs or scripts to build our neural network.