I think here is the problem. From ArrayCopy documentation:
If arrays are of different types, during copying it tries to transform each element of a source array into the type of the destination array. A string array can be copied into a string array only. Array of classes and structures containing objects that require initialization aren't copied. An array of structures can be copied into an array of the same type only.
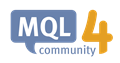
- docs.mql4.com
Yes, 'for' cycle helped.
void CopyCandlesArray(Candle &arr_source[],Candle &arr_dest[])
{
int newArrSize = ArraySize(arr_source);
ArrayResize(arr_dest, newArrSize);
for(int i=0; i<newArrSize; i++)
{
arr_dest[i] = arr_source[i];
}
}
And also it should be implement overloading for operator "=" in 'Candle' class. Like this:
void operator=(Candle& other)
{
high = other.high;
open = other.open;
close = other.close;
low = other.low;
time = other.time;
}

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Could someone say what is wrong with this code (I want to copy arr[] to candles[]):
class Pattern {
...
private:
Candle candles[];
...
void SetCandles(Candle &arr[]{
int newArrSize = ArraySize(arr);
ArrayResize(candles, newArrSize);
ArrayCopy(candles,arr,0,0,WHOLE_ARRAY);
}
...
}
Thanks for help!