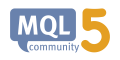
- www.mql5.com
- CTrade
- My EA doesn't execute my sell orders, it just executes my buy orders
- Ctrade - trade.sell
1. Like I have said in mql4 forum, https://www.mql5.com/en/forum/142265, you are indeed need to learn more.
2. When posting codes in forum, use SRC button to post the codes
4. When requesting Bid and Ask price, do NOT use SymbolInfoDouble(_Symbol,SYMBOL_ASK) or SymbolInfoDouble(_Symbol,SYMBOL_BID); instead use SymbolInfoTick() (https://www.mql5.com/en/docs/marketinformation/symbolinfotick) with MqlTick (https://www.mql5.com/en/docs/constants/structures/mqltick) - click that , there's example in there.
5. When opening a pending order, Always check against Freeze_Level and Stop_Level, if the pending price is withing Freeze_Level and/or Stop_Level then the pending can not be opened. In your example, you set the pending price at 5 points which is half pips without checking the Freeze_Level and Stop_Level. Click here SymbolInfoInteger() (https://www.mql5.com/en/docs/marketinformation/symbolinfointeger)
6. OrdersTotal() is total number of pending order while PositionTotal() is number of opened position. For one symbol, there can be only one position and several orders. Therefore your code here does not makes any sense for one symbol.
TotalRecords = PositionsTotal(); if (TotalRecords > OrdersTotal()) //--- does NOT make any sense.
7. Please do not show off your programming skill and call it "Simplest Step" if you still learning !. You are misleading many rookie.
- www.mql5.com
You can use the edit button on your previous post right, bottom corner.
#include <Trade\Trade.mqh> CTrade Execution; int TotalRecords,RecordsInPos; MqlTick AskBidValue; input double New_Amount_Greater_than_ACCOUNT_EQUITY = 0;//Amount Enter Should be Greater Account Equity void OnTick() { //----------------------------------------------------------------------------------------------------------------------- if (New_Amount_Greater_than_ACCOUNT_EQUITY > ACCOUNT_EQUITY && New_Amount_Greater_than_ACCOUNT_EQUITY - ACCOUNT_EQUITY >1.00) { if (OrdersTotal()==0) { if(SymbolInfoTick(Symbol(),AskBidValue)) { Execution.BuyStop(0.1,AskBidValue.ask+5,_Symbol,AskBidValue.ask-5,AskBidValue.ask+15); Execution.SellStop(0.1,AskBidValue.bid-5,_Symbol,AskBidValue.bid+5,AskBidValue.bid-15); } TotalRecords=OrdersTotal(); } //************************************************************************************* if (PositionsTotal()==0) { RecordsInPos=PositionsTotal(); } if (RecordsInPos>PositionsTotal()) { if(SymbolInfoTick(Symbol(),AskBidValue)) { Execution.BuyStop(0.1,AskBidValue.ask+5,_Symbol,AskBidValue.ask-5,AskBidValue.ask+15); Execution.SellStop(0.1,AskBidValue.bid-5,_Symbol,AskBidValue.bid+5,AskBidValue.bid-15); } RecordsInPos=PositionsTotal(); } //************************************************************************************* if (RecordsInPos<PositionsTotal()) {RecordsInPos=PositionsTotal();} //************************************************************************************* } //----------------------------------------------------------------------------------------------------------------------- }

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use