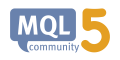
- www.mql5.com
Thanks phi.nuts - I have read that topic - and it would seem that guy has the exact same problem...
So because my broker decides not to have historical data before a certain date, then there is no way for me to get that data, and backtesting becomes impossible.
This is very bad news, and it looks like I will have to look for another trading platform where this problem does not exist.
Thanks phi.nuts - I have read that topic - and it would seem that guy has the exact same problem...
So because my broker decides not to have historical data before a certain date, then there is no way for me to get that data, and backtesting becomes impossible.
This is very bad news, and it looks like I will have to look for another trading platform where this problem does not exist.
Yes, I guess that's somewhat of a solution, but it doesn't seem very robust wouldn't you say?
The points that MaxTrader makes here are still valid no?
Hi Lori,
I am on Alpari and it has data

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I was surprised to find that 2000 - 2008 only has daily data; 2009 has hourly, and 2010 has hourly up until 7th April, and then minute bars from then until today.
So I then contacted my broker (Alpari UK) and their response was:
They suggested I try contact MetaQuotes.
I just find it so hard to believe that this is not supported.
If I go to the history folder (MetaQuotes\Terminal\...\bases\AlpariUK-MT5\history\EURUSD), the entire history for 2011 is 15MB. For ~15MB of data per year, I don't think it's that colossal to be honest.
In any event, I'm finding it hard to believe that Alpari and/or MetaTrader doesn't support back-testing before 2010, because it's just such a fundamental part of automated trading.
Is this a technical shortcoming, or is it the way Alpari has configured their server?
On a related note, does anyone know of an MT5 broker who does support minute bars back to 2000?
My script: