You should implement OnChartEvent() and catch user interaction.
See here for more details: Types of Chart Events
- docs.mql4.com
Thanks for the quick reply Drazen!
I want to change the objects at random first (think of that carnival game 'whack a mole'. The mole pops up through a hole, you hit it before it goes back in and pops up another hole, you get a point). I'm at the stage right now just randomly moving the mole around. Once I figure that out, I'll add the user interaction.
In that case, you should iterate through all objects on the chart.
See example for the ObjectsTotal() function.
Read in help everything about chart Objects.
To change object properties see functions ObjectSetInteger(), ObjectSetDouble(), ObjectSetString().
In that case, you should iterate through all objects on the chart.
See example for the ObjectsTotal() function.
Read in help everything about chart Objects.
To change object properties see functions ObjectSetInteger(), ObjectSetDouble(), ObjectSetString().
Well, that sucks. I was really hoping for a modifier method in the class.
This works:
//+------------------------------------------------------------------+ //| Build Grid.mq4 | //| Copyright 2014, Nondisclosure007 | //| http://no.link.yet | //+------------------------------------------------------------------+ #property copyright "Copyright 2014, Nondisclosure007" #property link "http://no.link.yet" #property version "1.00" #property strict extern int Box=15; extern int NumberOfRows=35; extern int NumberOfColumns=68; extern int Xindent=5; extern int Yindent=15; int Xrandom=0,Yrandom=0,ColorRandom=0; string LastCell, CurrCell; #include <ChartObjects\ChartObjectsTxtControls.mqh> CChartObjectEdit Cell; //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- X=Time Y=Price EventSetTimer(1); BuildIt(); //ChartRedraw(); return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- EventKillTimer(); ObjectsDeleteAll(); } //+------------------------------------------------------------------+ //| Expert Time function | //+------------------------------------------------------------------+ void BuildIt() { int Xmultiplier=0,Ymultiplier=0; for(int Column=0;Column<NumberOfColumns;Column++) { Xmultiplier=Column; if(Column>0) { Xmultiplier=(Column*Box)-Column; } for(int Row=0;Row<NumberOfRows;Row++)//row { Ymultiplier=Row; if(Row>0) { Ymultiplier=(Row*Box)-Row; } SetEditBox(StringConcatenate("Column:",Column," Row:",Row),0,Xindent+Xmultiplier,Yindent+Ymultiplier,Box,Box,clrBlack,clrGray); } } } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ void OnTimer() { WhackAMole(); //ChartRedraw(); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- } void WhackAMole() { ObjectSetInteger(0,CurrCell,OBJPROP_BGCOLOR,clrBlack); Xrandom=rand()%NumberOfColumns; Yrandom=rand()%NumberOfRows; ColorRandom=rand()%5; color newBackColor=clrNONE; switch(ColorRandom) { case 0:newBackColor=clrRed; break; case 1:newBackColor=clrYellow; break; case 2:newBackColor=clrBlue; break; case 3:newBackColor=clrLime; break; case 4:newBackColor=clrMagenta; break; default: break; } CurrCell=StringConcatenate("Column:",Xrandom," Row:",Yrandom); ObjectSetInteger(0,CurrCell,OBJPROP_BGCOLOR,newBackColor); } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ void SetEditBox(string name,int sub_window,int x,int y,int width,int height,color bg_color,color border_clr) { //--- reset the error value ResetLastError(); //--- create edit field Cell.Create(0,name,0,x,y,width,height); Cell.SetString(OBJPROP_TEXT," "); Cell.Font("Ariel"); Cell.FontSize(7); Cell.TextAlign(ALIGN_CENTER); Cell.ReadOnly(true); Cell.Corner(CORNER_LEFT_UPPER); Cell.Color(clrWhite); Cell.BackColor(bg_color); Cell.BorderColor(border_clr); Cell.Background(false); Cell.Selectable(false); Cell.Z_Order(0); }
There are OO wrappers around basic chart objects.
Look into the Include\Controls folder in your MetaEditor.
There is no documentation for MQL4, but this is partial implementation of the MQL5 standard library - https://www.mql5.com/en/docs/standardlibrary/cchart
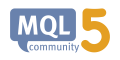
- www.mql5.com
There are OO wrappers around basic chart objects.
Look into the Include\Controls folder in your MetaEditor.
There is no documentation for MQL4, but this is partial implementation of the MQL5 standard library - https://www.mql5.com/en/docs/standardlibrary/cchart
Thanks.
I've seen those, but in the CEdit OO wrapper; the right methods aren't exposed.
I'll just have to create my own wrapper.
Again, thanks!

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I'm creating a kind of 'whack a mole' game here as a graphical board in MT4. The purpose isn't to be anything useful than an exercise in using the built in libraries that come with MT4.
The problem in my 'game' is that the last cell created is the only cell that changes color.
What I can't figure out for the life of me is why I can't seem to access an object later in program execution once I've created it. How can I then select the object and change it's properties?
Here's the code (I've put a comment in where I can't figure out where to select the object to modify it's properties):