I have written a bot that automatically places trades based on multiple indicators, after some trying and compiling I succeeded and went on with Metatrader 5 on Vantage, in the strategy tester it is giving me error code 4756 Invalid Request in the journal, I've already tried analysing the problem with chatgpt, but it doesn't seem to fix it..
Anyone any idea where it might go wrong?
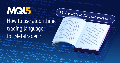
Documentation on MQL5: Constants, Enumerations and Structures / Trade Constants / Order Properties
- www.mql5.com
Requests to execute trade operations are formalized as orders. Each order has a variety of properties for reading. Information on them can be...
- Timeseries and Indicators Access
- Technical Indicators
- Custom Indicators
Mike Nobel:
I have written a bot that automatically places trades based on multiple indicators, after some trying and compiling I succeeded and went on with Metatrader 5 on Vantage, in the strategy tester it is giving me error code 4756 Invalid Request in the journal, I've already tried analysing the problem with chatgpt, but it doesn't seem to fix it..
I have written a bot that automatically places trades based on multiple indicators, after some trying and compiling I succeeded and went on with Metatrader 5 on Vantage, in the strategy tester it is giving me error code 4756 Invalid Request in the journal, I've already tried analysing the problem with chatgpt, but it doesn't seem to fix it..
Anyone any idea where it might go wrong?
Hello
You needed to add the request.action = TRADE_ACTION_DEAL;
and then call the GetFillingA function for the type filling.
void ExecuteTrade() { CalculateIndicators(); double atrDistance = ATR_Value[0] * StopLossATR_Multiplier; double stopLossDistance = atrDistance; double takeProfitDistance = stopLossDistance * RiskRewardRatio; MqlTradeRequest request = {}; MqlTradeResult result = {}; request.action = TRADE_ACTION_DEAL; request.symbol = Symbol(); request.volume = Lot_Size; request.deviation = Slippage; request.magic = MagicNumber; request.type_filling = GetFillingA(_Symbol); ENUM_ORDER_TYPE_FILLING GetFillingA( const string Symb, const uint Type = ORDER_FILLING_FOK ) { const ENUM_SYMBOL_TRADE_EXECUTION ExeMode = (ENUM_SYMBOL_TRADE_EXECUTION)::SymbolInfoInteger(Symb, SYMBOL_TRADE_EXEMODE); const int FillingMode = (int)::SymbolInfoInteger(Symb, SYMBOL_FILLING_MODE); return((FillingMode == 0 || (Type >= ORDER_FILLING_RETURN) || ((FillingMode & (Type + 1)) != Type + 1)) ? (((ExeMode == SYMBOL_TRADE_EXECUTION_EXCHANGE) || (ExeMode == SYMBOL_TRADE_EXECUTION_INSTANT)) ? ORDER_FILLING_RETURN : ((FillingMode == SYMBOL_FILLING_IOC) ? ORDER_FILLING_IOC : ORDER_FILLING_FOK)) : (ENUM_ORDER_TYPE_FILLING)Type); }
also check the order of your variables of handlBands. deviation and shift are in wrong order.

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register