Please enlighten my path!
I am writing a class to find values of manually drawn trendlines on the chart. However at compiling this class (and the EA) the toolbox throws an error:
Line 17 is here:
and the function getBorder(...) looks like:
I'm confused as the error points towards line 17, where it is refering to a hidden method calling, wich IMHO only could be the
method.
What do I not see that you guys hopefully see so obviously? And: do you know any alternative workaround for this method?
There is nothing related to ObjectGetValueByTime here.
The warning/error comes from the way, how (ambiguously) you composed several classes, specifically with the method getBorder - I suppose it's implemented in a base class, but is replaced with something similar in a derived class (which hides the base implementation), and MQL5 compiler "is not sure" what do you mean exactly. As a result, you can get unexpected behaviour of your program, and you need to clarify your intentions by explicitly calling base implementation in the derived class, if necessary.
For more details, please, look at the algobook - example is called OverrideVsOverload.mq5.
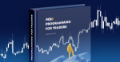
- www.mql5.com
Thnx, very fast responsive forum here!
I have indeed struggled with (obviously not completely) succesfully set up the Class: the header file and the implementation file. I don't blame chatGPT, but it helped me a lot ;)
I'll check the algobook with the example of OverrideVsOverload.mq5. I hope I can deal with it, I'm not familiair with lots of the terms and structures I encounter in the documentation files, hence the files and the question above. I hope you guys bear with me.
Here's my headers file: RateHeaders.mqh
/* Module to get position of bid in any given channel. Args accepted: @param up : upper border of channel @param lo : lower border of channel Functions: string getPosition : returns verbose position int posPercents : returns position in channel in percents between lo and up string priceDir : returns verbose direction of past bars int dirCount : returns number of bars of priceDir */ class Rate { private: string upperBorderName; string lowerBorderName; double getBorder(); double up; double lo; public: // Constructor Rate(void); //vars string position; double bid; // Functies string getPosition(); int posPercents(int shift); string priceDir(); int dirCount(); void setUpperBorderName(string name); void setlowerBorderName(string name); };
... and the implementation file RateImp.mqh :
#include "RateHeaders.mqh" // Implementatie van de constructor Rate::Rate() { }; // Implementatie van functies double getBorder(string name, int shift){ return ObjectGetValueByTime(0,name,iTime(_Symbol,PERIOD_CURRENT,shift)); } string Rate::getPosition() { return position; } int Rate::posPercents(int shift){ double res = getBorder(upperBorderName,shift); double sup = getBorder(lowerBorderName,shift); bid = SymbolInfoDouble(_Symbol,SYMBOL_BID); double width = res - sup; double diff = bid - sup; int percentage = int(floor(100. + (diff - width)/width * 100.)); return percentage; } void Rate::setUpperBorderName(string name) { upperBorderName = name; } void Rate::setlowerBorderName(string name) { lowerBorderName = name; }
Finally the EA:
//+------------------------------------------------------------------+ //| EA_25_02.mq5 | //| Copyright 2025, MetaQuotes Ltd. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2025, MetaQuotes Ltd." #property link "https://www.mql5.com" #property version "1.00" #include <RiskManagement.h> // Headerbestand #include <RiskManagement.mqh> // Implementatiebestand #include <RateHeaders.mqh> // Headerbestand #include <RateImp.mqh> // Implementatiebestand Rate rate; struct Channel{ double res; double sup; datetime prevPoint_RES; datetime latestPoint_RES; datetime prevPoint_SUP; datetime latestPoint_SUP; } channel; struct Quote{ string position; } q; string commentString; //+------------------------------------------------------------------+ int OnInit() { //--- getChannelValues(); Print(" channel.res: ",DoubleToString(channel.res,_Digits),"\n channel.sup: ",DoubleToString(channel.sup,_Digits)); //--- RiskManagement risicoManager(1.0,100.0); return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- getChannelValues(); q.position = rate.getPosition(); //--- updateComment(); } //+------------------------------------------------------------------+ void getChannelValues(){ channel.res = ObjectGetValueByTime(0,"ChannelResistance",iTime(_Symbol,PERIOD_CURRENT,0),0); channel.sup = ObjectGetValueByTime(0,"ChannelSupport",iTime(_Symbol,PERIOD_CURRENT,0),0); } //+------------------------------------------------------------------+ void updateComment(){ commentString = StringFormat( " position: %s " ,q.position ); //--- from any earlier EA, to be adapted yet //if(rate.position == "Breakout Boven" || rate.position == "Breakout Onder"){ // string adapt = ""; // double avgBar = getAVGbarLength(); // double bar1L = iHigh(_Symbol,PERIOD_CURRENT,1) - iLow(_Symbol,PERIOD_CURRENT,1); // adapt = (rate.position == "Breakout Boven" && bar1L < avgBar/3 && iClose(_Symbol,PERIOD_CURRENT,0) < iOpen(_Symbol,PERIOD_CURRENT,1) ) ? " maar op punt van bounce down" : // (rate.position == "Breakout Onder" && bar1L < avgBar/3 && iClose(_Symbol,PERIOD_CURRENT,0) > iOpen(_Symbol,PERIOD_CURRENT,1) ) ? " maar op punt van bounce up" : // " en nog niet klaar!"; // commentString = commentString + adapt; // } //commentString = commentString +" \n"+ ArrayToString(); Comment(commentString); }
Thnx, very fast responsive forum here!
I have indeed struggled with (obviously not completely) succesfully set up the Class: the header file and the implementation file. I don't blame chatGPT, but it helped me a lot ;)
I'll check the algobook with the example of OverrideVsOverload.mq5. I hope I can deal with it, I'm not familiair with lots of the terms and structures I encounter in the documentation files, hence the files and the question above. I hope you guys bear with me.
Here's my headers file: RateHeaders.mqh
... and the implementation file RateImp.mqh :
Finally the EA:
I highlighted what you missed.
P.S. Generating code is a bad idea. Write it yourself
class Rate { private : string upperBorderName; string lowerBorderName; double getBorder(string name, int shift); public : // Constructor Rate( void ); // Functies int posPercents( int shift); }; // Implementatie van de constructor Rate::Rate() { }; // Implementatie van functies double Rate::getBorder( string name, int shift){ return ObjectGetValueByTime ( 0 ,name, iTime ( _Symbol , PERIOD_CURRENT ,shift)); } int Rate::posPercents( int shift){ double res = getBorder(upperBorderName,shift); double sup = getBorder(lowerBorderName,shift); return 0; } void OnStart() {}
Please, don't request help for ChatGPT (or other A.I.) generated code. It generates horrible code, and we are not here to "correct" it's mistakes.
The forum is here to help YOU in learning to code it entirely by yourself, and usually any topics with generated code are removed completely.
So, put in the effort and time to learn to code manually, referencing the documentation and reading the programming book.
Don't use ChatGPT (or other A.I.) generator. You will only end up making it worse for your own proper coding education and prolong the learning.
Thank you so much again, for both the correction in my code and the nobel advise to avoid GPT because it leads to not/understandable code. I noticed this already at my own pace!
In my particular case, I felt it would be honest to mention the aid of GPT to get me going.
One of the struggles I had, was that the GPT agent ´told´ me to make two files. Regarding the code correction by Vladislav Boyko (and my upcoming insights reading the docs) a Class can be created in just one single file.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi,
Please enlighten my path!
I am writing a class to find values of manually drawn trendlines on the chart. However at compiling this class (and the EA) the toolbox throws an error:
Line 17 is here:
and the function getBorder(...) looks like:
I'm confused as the error points towards line 17, where it is refering to a hidden method calling, wich IMHO only could be the
ObjectGetValueByTime(...)
method.
What do I not see that you guys hopefully see so obviously? And: do you know any alternative workaround for this method?
Thnx!